API Reference
The Recharge API is primarily a REST API with some RPC endpoints to support common operations. It has predictable, resource-oriented URLs, accepts JSON-encoded request bodies, returns JSON-encoded responses, and uses standard HTTP response codes, authentication, and methods.
Related guides: Generate API tokens, Using the API
API and Platforms compatibility
Recharge offers hosted solutions and integrates with various ecommerce platforms to process recurring transactions with the setup of your choice. In order to be compatible with those platforms some of our API resources and endpoints may be limited in use to a subset of platforms. When that is the case we will flag with the help of tags the checkout/platform association for which that feature is compatible.
When there is no restriction of compatibility no tags will appear.
Below is a legend of the tags you may come across:
Tag | Checkout solution | Ecommerce platform |
---|---|---|
BigCommerce | Recharge hosted | BigCommerce |
Custom | Recharge hosted or API-first | Custom |
RCS | Recharge hosted | Shopify |
SCI | Shopify hosted | Shopify |
You may also come across other tags specifying regional restrictions (e.g. USA Only) or new releases (e.g. Alpha, Beta).
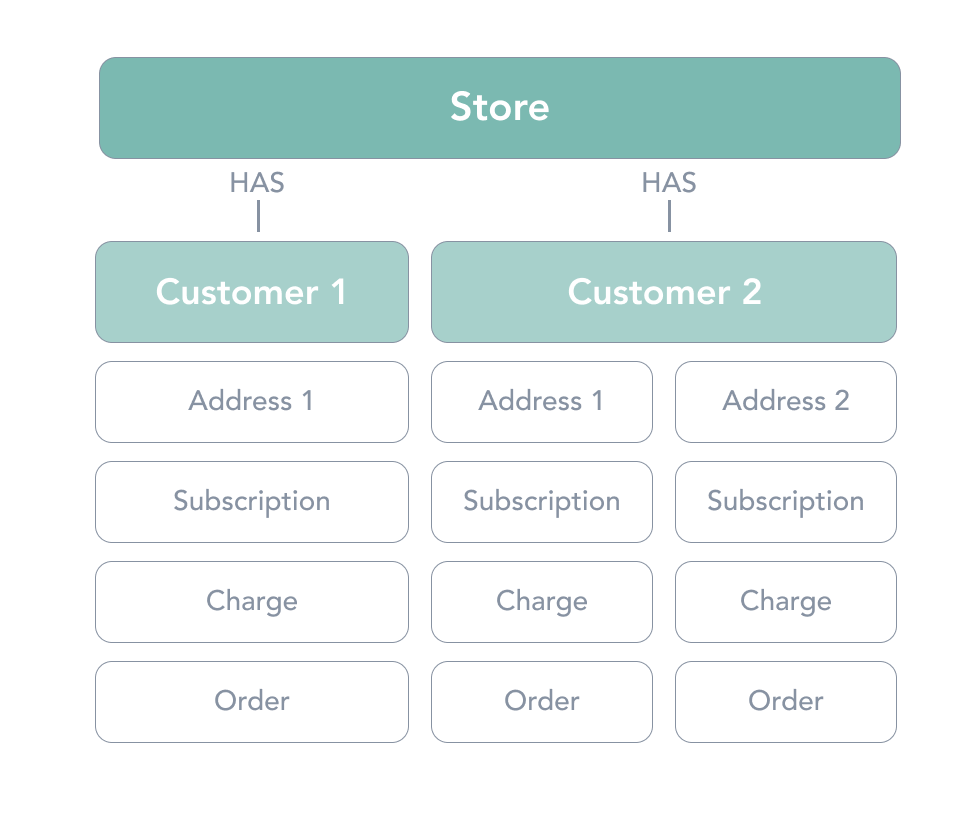
https://api.rechargeapps.com
Authentication
Recharge uses API keys to authenticate requests.
Each request to the API should contain an API token in the following header:
X-Recharge-Access-Token:store_api_token
Replace store_api_token
with your API key.
All requests must be made over HTTPS.
API Token Scopes
Scopes can be set up from the API token edit page in Recharge to control the level of access of an API token.
The API currently supports the scopes below:
Write | Read |
---|---|
read_accounts |
|
write_batches |
read_batches |
write_customers |
read_customers |
write_discounts |
read_discounts |
read_events |
|
write_notifications |
|
write_orders |
read_orders |
write_payment_methods |
read_payment_methods |
write_products |
read_products |
write_subscriptions |
read_subscriptions |
read_store |
|
read_credit_accounts |
|
read_credit_adjustments |
curl -i -H 'X-Recharge-Access-Token: your_api_token'
-X GET
Versioning
All requests will use your account API settings, unless you send a X-Recharge-Version
header to specify the version.
You can use the same token to make calls to all versions. When no version is specified it will default to the default version on your store.
Existing API Versions | Release notes |
---|---|
2021-11 |
2021-11 release notes |
2021-01 |
Responses
Recharge uses conventional HTTP
response codes to indicate the success or failure of an API request. In general, codes in the 2xx
range indicate success, codes in the 4xx
range indicate an error that failed given the information provided ( e.g. a required parameter was omitted, a charge failed, etc ), and codes in the 5xx
range indicate an error with Recharge’s servers.
200 - OK: Everything worked as expected.
201 - OK: The request was successful, created a new resource, and resource created is in the body.
202 - OK: The request has been accepted and is in processing.
204 - OK: The server has successfully fulfilled the request and there is no content to send in the response body.
400 - Bad Request: The request was unacceptable, often due to a missing required parameter.
401 - Unauthorized: No valid API key was provided.
402 - Request Failed: The parameters were valid but the request failed.
403 - The request was authenticated but not authorized for the requested resource (permission scope error).
403 - Uninstalled Store.
404 - Not Found: The requested resource doesn’t exist.
405 - Method Not Allowed: The method is not allowed for this URI.
406 - The request was unacceptable, or requesting a data source which is not allowed although permissions permit the request.
409 - Conflict: You will get this error when you try to send two requests to edit an address or any of its child objects at the same time, in order to avoid out of date information being returned.
415 - The request body was not a JSON object.
422 - The request was understood but cannot be processed due to invalid or missing supplemental information.
426 - The request was made using an invalid API version.
429 - The request has been rate limited.
500 - Internal server error.
501 - The resource requested has not been implemented in the current version but may be implemented in the future.
503 - A 3rd party service on which the request depends has timed out.
Extending responses
Our API endpoints and webhooks allow developers to extend responses with additional data in order to optimize calls, allowing for simpler and more efficient implementations.
The API supports including additional objects when using a GET
request to retrieve a list or a GET
request to retrieve a record by a specific id. This is achieved by using an include
query parameter in the request URL. The include
value contains the object or objects you want to include in the response of your request. On routes where multiple includes are available, you are able to pass multiple values separated by a comma (include=customer,metafields
). The below table defines available include
values for commonly used resources of the API.
Webhooks support included_objects
on the topics listed below. Webhook included_objects
accepts an array of supported values ("included_objects": [ "customer", "metafields"]
). Specifying included_objects
will return an enriched payload, containing the original resource and the associated included objects.
When including charge_activities
in API calls or webhooks, note that only the last 90 days of activities will be included in the response.
Resource | Endpoints | Webhook topics | Supported include values |
Supported included_objects values |
---|---|---|---|---|
Addresses |
GET /addresses GET /addresses/{id} |
address/created address/updated |
charge_activities customer discount payment_methods subscriptions |
customer payment_methods |
Charges |
GET /charges GET /charges/{id} |
charge/created charge/failed charge/max_retries_reached charge/paid charge/refunded charge/uncaptured charge/upcoming charge/updated charge/deleted |
charge_activities (beta)customer metafields payment_methods |
customer metafields payment_methods |
Customers |
GET /customers GET /customers/{id} |
customer/activated customer/created customer/deactivated customer/payment_method_updated customer/updated customer/deleted |
addresses metafields payment_methods subscriptions |
addresses metafields payment_methods |
Orders |
GET /orders GET /orders/{id} |
order/cancelled order/created order/deleted order/processed order/payment_captured order/upcoming order/updated order/success |
customer metafields |
customer metafields |
Payment Methods |
GET /payment_methods GET /payment_methods/{id} |
addresses |
addresses |
|
Subscriptions |
GET /subscriptions GET /subscriptions/{id} |
subscription/activated subscription/cancelled subscription/created subscription/deleted subscription/skipped subscription/updated subscription/unskipped subscription/paused |
address charge_activities customer metafields bundle_product bundle_selections |
customer metafields |
Cursor Pagination
By default, calls for a list of objects will return 50 results. Using the limit
parameter, that can be increased to 250 results per response.
When there are more results than the current limit
a cursor may be used to request additional results.
The next_cursor
and previous_cursor
attributes are are included in all list responses.
To request the next set of results, find the next_cursor
in the list response and include it in the url with the cursor parameter e.g. GET https://api.rechargeapps.com/subscriptions?limit=250&cursor=<next_cursor>
To request the previous set of results, find the previous_cursor
in the list response and include it in the url with the cursor parameter e.g. GET https://api.rechargeapps.com/subscriptions?limit=250&cursor=<previous_cursor>
Retrieving total number of records
Starting with the 2021-11
version of the API, you will not be able to retrieve a count of total records for a given GET
request. If you are building a UI page that allows end users to paginate through result sets (such as paginating through a list of orders or subscriptions), we recommend that your pagination implementation allow users to go to the next and previous page of results (as opposed to allowing users to jump to specific page in the results). This aligns well with the previous_cursor
and the next_cursor
fields included in all list responses.
URL="https://api.rechargeapps.com/charges?limit=5"
response=$(curl -s -w "%{http_code}"\
-H 'X-Recharge-Access-Token: your_api_token' \
-H 'X-Recharge-Version: 2021-11' \ -X GET $URL)
content=$(sed '$ d' <<< "$response") # get all but the last line which contains the status code
# Display results
echo $content | jq "."
# parse next url
echo "Next URL"
next_cursor=$(jq ".next_cursor" <<< "${content}")
# Notice next_cursor value is passed as page_info query param
echo "$URL&page_info=$next_cursor"
Sorting
The API supports sorting of results when using a GET
request to retrieve a list. Sorting is achieved using a sort_by
query parameter in the request URL. The sort_by
value contains the parameter and sort direction for your results (ascending or descending), and available sort_by values vary between resources. The below table defines available sort_by
options for commonly used resources.
Resource | Supported sort_by_values |
---|---|
Address |
Default: id-desc Options: id-asc id-desc updated_at-asc updated_at-desc |
Async Batch |
Default: id-desc Options: id-asc id-desc created_at-asc created_at-desc |
Charge |
Default: id-asc Options: id-asc id-desc created_at-asc created_at-desc updated_at-asc updated_at-desc scheduled_at-asc scheduled_at-desc |
Customer |
Default: id-desc Options: id-asc id-desc created_at-asc created_at-desc updated_at-asc updated_at-desc |
Discount |
Default: id-desc Options: id-asc id-desc created_at-asc created_at-desc updated_at-asc updated_at-desc |
Metafield |
Default: id-desc Options: id-asc id-desc updated_at-asc updated_at-desc |
Onetime |
Default: id-desc Options: id-asc id-desc created_at-asc created_at-desc updated_at-asc updated_at-desc |
Order |
Default: id-desc Options: id-asc id-desc updated_at-asc updated_at-desc processed_at-asc processed_at-desc scheduled_at-asc scheduled_at-desc |
Plan |
Default: id-desc Options: id-asc id-desc updated_at-asc updated_at-desc |
Subscription |
Default: id-desc Options: id-asc id-desc created_at-asc created_at-desc updated_at-asc updated_at-desc |
Webhook |
Default: id-desc Options: id-asc id-desc |
Addresses
An Addresses record represents a shipping address. Each customer can have multiple addresses. Subscriptions are a child object of an address.
The address object
An Addresses record represents a shipping address. Each customer can have multiple addresses. Subscriptions are a child object of an address.
Attributes
- idinteger
Unique numeric identifier for the
Address
. - payment_method_idinteger
Unique numeric identifier for the
Payment Method
associated to theAddress
. - address1string
The street associated with the
Address
. - address2string
Any additional information associated with the
Address
. - citystring
The city associated with the address.
- companystring
The company associated with the address.
- country_codestring
2-letter country code.
- customer_idinteger
Unique numeric identifier for the customer associated with the address.
- discountsarray
A list of discounts applied on the address. These discounts will apply to future recurring charges associated with this address.
Show object attributes - first_namestring
The customer’s first name associated with the address.
- last_namestring
The customer’s last name associated with the address.
Replaces cart_attributes. Extra information that is added to the order.
Show object attributes- order_notestring
Notes to be added to all orders associated with the address.
- phonestring
The phone number associated with the address.
The currency on the subscription contract in Shopify.
Only set if the currency is different from the store-level currency. Else, will default to store-level currency.
- provincestring
The state or province associated with the address.
- shipping_lines_conservedarray
Shipping rates that have previously been overridden via
shipping_lines_override
but are currently inactive.Show object attributes - shipping_lines_overridearray
Used when shipping rates need to be overridden.
If this parameter has value
null
, rates will be fetched whenCharge
is created or regeneratedShow object attributes - zipstring
The zip or postal code associated with the address.
- created_atdatetime
The date and time when the address was created.
- updated_atdatetime
The date and time when the address was last updated.
Error related attributes
More Attributes
{
"address": {
"id": 21317826,
"payment_method_id": 17874235,
"address1": "1776 Washington Street",
"address2": "",
"city": "Los Angeles",
"company": "Recharge",
"country_code": "US",
"created_at": "2018-11-14T09:00:01+00:00",
"customer_id": 18819267,
"discounts": [
{
"id": 12345,
"code": "TESTCODE10",
"value": 10,
"value_type": "fixed_amount"
}
],
"first_name": "John",
"last_name": "Doe",
"order_attributes": [
{
"name": "custom name",
"value": "custom value"
}
],
"order_note": "My recurring order note.",
"phone": "5551234567",
"presentment_currency": "USD",
"shipping_lines_conserved": [],
"shipping_lines_override": [
{
"code": "Standard Shipping",
"price": "0.00",
"title": "Standard Shipping"
}
],
"updated_at": "2018-11-14T09:00:01+00:00",
"zip": "90404"
}
}
Create an address
Create a new address for a customer.
Body Parameters
- customer_idinteger* Required
Unique numeric identifier for the customer associated with the address.
- address1string* Required
The street associated with the
Address
. Minimum length is 1 character. - address2string
Any additional information associated with the shipping address.
- citystring* Required
The city associated with the shipping address.
- companystring
The company associated with the shipping address.
- country_codestring* Required
2-letter country code.
Check if the store supports shipping to this country. This is set by the merchant in their Shipping Settings page.
- discountsarray
List of discounts applied on the
Address
.Show object attributes - first_namestring* Required
The customer’s first name associated with the address. Minimum length is 1 character.
- last_namestring* Required
The customer’s last name associated with the address. Minimum length is 1 character.
Extra information that is added to the order.
Show object attributes- order_notestring
Notes to be added to all orders associated with the address.
- payment_method_idinteger
Payment method id for the
Payment_method
to be associated to this address. - phonestring* Required
The phone number associated with the address. Must be included in the request schema but can be an empty string.
- presentment_currencystring
The currency that charges on this address will be processed in. If no presentment_currency is passed, it will be set to your default store currency.
- provincestring* Required
The state or province associated with the address. Check if country requires a province
COUNTRIES_REQUIRING_PROVINCE
. - shipping_lines_overridearray
Used when shipping rates need to be overridden. If this parameter has value
null
, rates will be fetched when a relatedCharge
is created or regeneratedShow object attributes - zipstring* Required
The zip or postal code associated with the address.
Check if the
country
requires azip
codeCOUNTRIES_NOT_REQUIRING_ZIP
. If not included in the list then a zip code with the minimum length of 1 character is required. If the country isUnited States
validate against regexUNITED_STATES_ZIP_REGEX
. If country isUnited Kingdom
then validate against regexUNITED_KINGDOM_ZIP_REGEX
.
More Parameters
Responses
- 201
OK: The request was successful, created a new resource, and resource created is in the body.
Show response object
curl https://api.rechargeapps.com/addresses \
-H 'X-Recharge-Version: 2021-11' \
-H 'Content-Type: application/json' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d '{
"customer_id": 22152215,
"address1": "1776 Washington Street",
"address2": "",
"city": "Los Angeles",
"company": "Recharge",
"country_code": "US",
"first_name": "John",
"last_name": "Doe",
"order_attributes": [
{
"name": "custom name",
"value": "custom value"
}
],
"phone": "5551234567",
"presentment_currency": "USD",
"province": "California",
"zip": "90404"
}'
{
"address": {
"id": 42171447,
"address1": "1030 Barnum Ave",
"address2": "Suite 101",
"city": "Stratford",
"company": "Fake Company",
"country_code": "US",
"created_at": "2022-10-12T20:16:09+00:00",
"customer_id": 37657002,
"discounts": [],
"first_name": "Fake First",
"last_name": "Fake Last",
"order_attributes": [
{
"name": "custom name",
"value": "custom value"
}
],
"order_note": "My recurring order note",
"phone": "999-999-9999",
"presentment_currency": "USD",
"province": "Connecticut",
"shipping_lines_conserved": [],
"shipping_lines_override": [],
"updated_at": "2022-10-12T20:16:09+00:00",
"zip": "06614"
}
}
Retrieve an address
Retrieves address for customer based on specified address id.
Responses
- 200
successful response
Show response object
curl 'https://api.rechargeapps.com/addresses/21317826' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token'
{
"address": {
"id": 42171447,
"payment_method_id": 17874235,
"address1": "1030 Barnum Ave",
"address2": "Suite 101",
"city": "Stratford",
"company": "Fake Company",
"country_code": "US",
"created_at": "2022-10-12T20:16:09+00:00",
"customer_id": 37657002,
"discounts": [],
"first_name": "Fake First",
"last_name": "Fake Last",
"order_attributes": [
{
"name": "custom name",
"value": "custom value"
}
],
"order_note": "My recurring order note",
"phone": "999-999-9999",
"presentment_currency": "USD",
"province": "Connecticut",
"shipping_lines_conserved": [],
"shipping_lines_override": [],
"updated_at": "2022-10-12T20:16:09+00:00",
"zip": "06614"
}
}
Update an address
Updates an existing address to match the specified parameters.
Example: remove discounts from an address
To remove all discounts from an address, set thediscounts
parameter to an empty array: {"discounts": []}
Note: When updating the country
property you will have to update the zip
property as well, otherwise you will receive an error.
Body Parameters
- address1string
The street associated with the
Address
. Minimum length is 1 character. - address2string
Any additional information associated with the shipping address.
- citystring
The city associated with the shipping address. Minimum length is 1 character.
- companystring
The company associated with the shipping address.
- country_codestring
2-letter country code.
Check if the
store
supports shipping to this country. This is set by the merchant in their Shipping Settings page. - discountsarray
A list of discounts applied on the address. These discounts will apply to future recurring charges associated with this address.
Show object attributes - first_namestring
The customer’s first name associated with the address. Minimum length is 1 character.
- last_namestring
The customer’s last name associated with the address. Minimum length is 1 character.
Extra information that is added to the order.
Show object attributes- order_notestring
Notes to be added to all orders associated with the address.
- payment_method_idinteger
Payment method id for the
Payment_method
to be associated to this address. - phonestring
The phone number associated with the address. Must be included in the request schema but can be an empty string.
- provincestring
The state or province associated with the address. Check if country requires a province
COUNTRIES_REQUIRING_PROVINCE
. Used when shipping rates need to be overridden. If this parameter has value
null
, rates will be fetched when a relatedCharge
is created or regenerated.Show object attributes- zipstring
The zip or postal code associated with the address. Check if the country requires a zip code
COUNTRIES_NOT_REQUIRING_ZIP
. If not included in the list then a zip code with the minimum length of 1 character is required. If the country isUnited States
then validate against regexUNITED_STATES_ZIP_REGEX
. If country isUnited Kingdom
then validate against regexUNITED_KINGDOM_ZIP_REGEX
.
More Parameters
Responses
- 200
successful response
Show response object
curl -X PUT 'https://api.rechargeapps.com/addresses/38700614' \
-H 'X-Recharge-Version: 2021-11' \
-H 'Content-Type: application/json' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d '{"address1": "1776 Washington Street"}'
{
"address": {
"id": 42171447,
"payment_method_id": 17874235,
"address1": "1030 Barnum Ave",
"address2": "Suite 101",
"city": "Stratford",
"company": "Fake Company",
"country_code": "US",
"created_at": "2022-10-12T20:16:09+00:00",
"customer_id": 37657002,
"discounts": [],
"first_name": "Fake First",
"last_name": "Fake Last",
"order_attributes": [
{
"name": "custom name",
"value": "custom value"
}
],
"order_note": "My recurring order note",
"phone": "999-999-9999",
"presentment_currency": "USD",
"province": "Connecticut",
"shipping_lines_conserved": [],
"shipping_lines_override": [],
"updated_at": "2022-10-12T20:16:09+00:00",
"zip": "06614"
}
}
Delete an address
It is possible to delete certain addresses from the store using API.
Only Addresses
with no active Subscriptions
can be deleted.
Responses
- 204
Content Deleted: The server has successfully fulfilled the request and deleted the desired object and there is no content to send in the response body.
Show response object
curl -X DELETE 'https://api.rechargeapps.com/addresses/38700614'\
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token'
{}
List addresses
Returns all addresses from the store, or addresses for the user given in the parameter.
HTTP examples
GET /addresses
GET /addresses?customer_id=<customer_id>
GET /addresses?discount_id=<discount_id>
GET /addresses?discount_code=<discount_code>
You can combine created_at_min
and created_at_max
to return all addresses created in the given timespan. This also applies to updated_at_min
and updated_at_max
parameters.
Query Parameters
- created_at_maxdatetime
Returns addresses created before the given time.
- created_at_mindatetime
Returns addresses created after the given time.
- customer_idinteger
Unique identifier of the customer.
- discount_codestring
Returns addresses that have the provided discount_code.
- discount_idstring
Returns addresses that have the provided discount_id.
- idsstring
Filter addresses by id. If passing multiple values, must be comma separated. Non-integer values will result in a 422 error
- limitstring
Default: 50
Max: 250
The amount of results. Default is 50 while the maximum is 250.
- pagestring*Deprecated
Default: 1
The page to show. Default is 1.
Page-based pagination has been deprecated but still available to use for pages up to a 100. If you need data past this point, use cursor pagination.
- updated_at_maxstring
Returns addresses updated before the given date.
- updated_at_minstring
Returns addresses updated after the given time.
- is_activeboolean
Returns active addresses.
More Parameters
Responses
- 200
successful response
Show response object
curl 'https://api.rechargeapps.com/addresses' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d limit=3 -G
{
"addresses": [
{
"id": 42171447,
"payment_method_id": 17874235,
"address1": "1030 Barnum Ave",
"address2": "Suite 101",
"city": "Stratford",
"company": "Fake Company",
"country_code": "US",
"created_at": "2022-10-12T20:16:09+00:00",
"customer_id": 37657002,
"discounts": [
{
"id": 123456
}
],
"first_name": "Fake First",
"last_name": "Fake Last",
"order_attributes": [
{
"name": "custom name",
"value": "custom value"
}
],
"order_note": "My recurring order note",
"phone": "999-999-9999",
"presentment_currency": "USD",
"province": "Connecticut",
"shipping_lines_conserved": [],
"shipping_lines_override": [
{
"code": "Standard Shipping",
"price": "0.00",
"title": "Standard Shipping"
}
],
"updated_at": "2022-10-12T20:16:09+00:00",
"zip": "06614"
}
]
}
Merge addresses
Merges up to 10 source addresses into 1 target address.
If one of the Addresses
being merged has a different presentment currency, the entire merge will fail and throw an error.
Body Parameters
- delete_source_addressesboolean
Indicates whether source addresses should be deleted.
- next_charge_datestring
Specifies the next charge date of the associated subscriptions on the target address.
- target_addressobject* Required
The address all of the subscriptions should be moved to.
Show object attributes - source_addressesarray* Required
The list of addresses that the subscriptions should move from.
More Parameters
Responses
- 200
successful response
Show response object
curl -X POST 'https://api.rechargeapps.com/addresses/merge' \
-H 'X-Recharge-Version: 2021-11' \
-H 'Content-Type: application/json' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d '{"target_address": {"id": 42171447}, "source_addresses": [{"id": 42171446}, {"id": 42171445}]}'
{
"address": {
"id": 42171447,
"payment_method_id": 17874235,
"address1": "1030 Barnum Ave",
"address2": "Suite 101",
"city": "Stratford",
"company": "Fake Company",
"country_code": "US",
"created_at": "2022-10-12T20:16:09+00:00",
"customer_id": 37657002,
"discounts": [],
"first_name": "Fake First",
"last_name": "Fake Last",
"order_attributes": [
{
"name": "custom name",
"value": "custom value"
}
],
"order_note": "My recurring order note",
"phone": "999-999-9999",
"presentment_currency": "USD",
"province": "Connecticut",
"shipping_lines_conserved": [],
"shipping_lines_override": [],
"updated_at": "2022-10-12T20:16:09+00:00",
"zip": "06614"
}
}
Skip future charge
Skip a Charge
in the future for one or multiple Subscriptions
associated with the Address
.
If the Charge
does not exist on the date, it will be created with a SKIPPED
status.
Body Parameters
- datedatetime* Required
The date in the future of the
Charge
to be skipped.This date must be within the delivery schedules of the
Customer
. - subscription_idsarray* Required
A list containing the
Subscription
IDs to be skipped.
More Parameters
Responses
- 200
successful response
Show response object - 404
the
Address
withid
does not existShow response object - 422
invalid data passed to the endpoint
Show response object
curl 'https://api.rechargeapps.com/addresses/91977136/charges/skip' \
-H 'X-Recharge-Version: 2021-11' \
-H 'Content-Type: application/json' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d '{"date": "2022-09-15", "purchase_item_ids": [27363808, 27363809]}'
{
"charge": {
"id": 377749210,
"address_id": 42171447,
"analytics_data": {
"utm_params": [
{
"utm_source": "facebook",
"utm_medium": "cpc"
}
]
},
"billing_address": {
"address1": "601 SW Washing St.",
"address2": "Suite 101",
"city": "Portland",
"company": "Acme Corp",
"country_code": "US",
"first_name": "Jane",
"last_name": "Doe",
"phone": "888-888-8888",
"province": "Oregon",
"zip": "97205"
},
"client_details": {
"browser_ip": "192.168.0.1",
"user_agent": "safari webkit"
},
"created_at": "2021-11-09T19:22:13+00:00",
"currency": "USD",
"customer": {
"id": 37657002,
"email": "test@test.com",
"external_customer_id": {
"ecommerce": "2879413682227"
},
"hash": "7e706455cbd13e40"
},
"discounts": [
{
"id": 12345,
"code": "TESTCODE10",
"value": 10,
"value_type": "fixed_amount"
}
],
"error": null,
"error_type": null,
"external_order_id": {
"ecommerce": "2541635698739"
},
"external_transaction_id": {
"payment_processor": "ch_1HzWElJ2zqHvZRd1TWKFFqDR"
},
"has_uncommitted_changes": false,
"line_items": [
{
"purchase_item_id": 63898947,
"external_product_id": {
"ecommerce": "4381728735283"
},
"external_variant_id": {
"ecommerce": "999999999999"
},
"grams": 454,
"handle": "shirt-package",
"images": {
"large": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h_large.jpg",
"medium": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h_medium.jpg",
"original": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h.jpg",
"small": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h_small.jpg"
},
"original_price": "10.00",
"properties": [
{
"name": "Color",
"value": "Blue"
}
],
"purchase_item_type": "subscription",
"quantity": 1,
"sku": "TOM0001",
"tax_due": "1.30",
"tax_lines": [
{
"price": "0.9931",
"rate": "0.0725",
"title": "CA State Tax",
"unit_price": "0.3310"
},
{
"price": "0.3082",
"rate": "0.0225",
"title": "LA County Tax",
"unit_price": "0.1027"
}
],
"taxable": true,
"taxable_amount": "10.00",
"title": "Shirt package",
"total_price": "11.30",
"unit_price": "10.00",
"unit_price_includes_tax": false,
"variant_title": "Blue T-shirt"
}
],
"note": "next order in sequence 3",
"order_attributes": [
{
"name": "Checkout-Method",
"value": "delivery"
}
],
"orders_count": 1,
"payment_processor": "stripe",
"processed_at": null,
"retry_date": null,
"scheduled_at": "2021-12-09",
"shipping_address": {
"address1": "1030 Barnum Ave",
"address2": "Suite 101",
"city": "Stratford",
"company": "Fake Company",
"country_code": "US",
"first_name": "Fake First",
"last_name": "Fake Last",
"phone": "999-999-9999",
"province": "Connecticut",
"zip": "06614"
},
"shipping_lines": [
{
"code": "Standard",
"price": "4.90",
"source": "shopify",
"taxable": true,
"tax_lines": [
{
"price": "0.355",
"rate": 0.0725,
"title": "CA State Tax"
},
{
"price": "0.110",
"rate": 0.0225,
"title": "Los Angeles County Tax"
}
],
"title": "Standard"
}
],
"status": "skipped",
"subtotal_price": "10.00",
"tags": "Subscription, Subscription Recurring Order",
"tax_lines": [
{
"price": "0.950",
"rate": 0.0725,
"title": "CA State Tax"
},
{
"price": "0.335",
"rate": 0.0225,
"title": "Los Angeles County Tax"
}
],
"taxable": true,
"total_discounts": "10.0",
"total_line_items_price": "10.00",
"total_price": "11.29",
"total_refunds": "0.00",
"total_tax": "1.29",
"total_weight_grams": 454,
"type": "recurring",
"updated_at": "2021-11-09T23:59:32+00:00"
}
}
A bundle selection represents the contents within a Bundle linked to an individual Subscription. It can represent the selection for upcoming orders or past orders. A BundleSelection
is associated with a corresponding Subscription
and a BundleVariant
(the BundleVariant
is used to validate contents in the selection). When a new order for the associated Subscription
occurs, it will extract the current contents of the BundleSelection
for the Bundle item in the order.
A bundle selection represents the contents within a Bundle linked to an individual Subscription. It can represent the selection for upcoming orders or past orders. A BundleSelection
is associated with a corresponding Subscription
and a BundleVariant
(the BundleVariant
is used to validate contents in the selection).
Attributes
- idinteger
The unique numeric identifier for the
BundleSelection
. - bundle_variant_idinteger
The ID of the
BundleVariant
associated with theBundleSelection
. - purchase_item_idinteger
The ID of the
PurchaseItem
associated with theBundleSelection
. - created_atdatetime
The date and time when the contents were selected.
- external_product_idstring
The product id as it appears in the external e-commerce platform. The
external_product_id
of theProduct
record in Recharge, linking theBundleSelection
to aProduct
associated with a Bundle. - external_variant_idstring
The variant id as it appears in the external e-commerce platform. The
external_variant_id
of theProduct
record in Recharge, linking theBundleSelection
to aProduct
associated with a Bundle. - itemsarray
A list of
item
objects, each containing information about a distinct product selected as part of the Bundle.Show object attributes - updated_atdatetime
The date and time at which the
BundleSelection
was most recently updated.
Error related attributes
More Attributes
{
"bundle_selection": {
"id": 100714428,
"bundle_variant": 382417,
"purchase_item_id": 199820883,
"created_at": "2022-08-26T18:31:28+00:00",
"external_product_id": "7121697210548",
"external_variant_id": "41450875650228",
"items": [
{
"id": 541,
"collection_id": "287569608884",
"collection_source": "shopify",
"created_at": "2022-08-26T18:31:28+00:00",
"external_product_id": "7121693671604",
"external_variant_id": "41450853105844",
"quantity": 3,
"updated_at": "2022-08-26T18:52:41+00:00"
}
],
"updated_at": "2022-08-26T18:31:28+00:00"
}
}
Returns a list of BundleSelections
.
HTTP request examples
GET /bundle_selections?purchase_item_ids=:purchase_item_id_1,:purchase_item_id_2
GET /bundle_selections?bundle_variant_ids=:bundle_variant_id_1,:bundle_variant_id_2
GET /bundle_selections?limit=1
GET /bundle_selections?limit=1&page=2
GET /bundle_selections?sort_by=updated_at-desc
BundleSelections
are sorted descending by ID value by default.BundleSelections
endpoints are available for Recharge Pro merchants. If you’re interested in leveraging the Recharge bundle_selections
endpoints, reach out to your account manager or our Support team.
Learn more about Recharge Pro.
Query Parameters
- purchase_item_idsstring
Filter
BundleSelections
bySubscription
orOnetime
ID.If passing multiple values, must be comma separated. Non-integer values will result in a
422
error. - bundle_variant_idsstring
Filter
BundleSelections
byBundleVariants
.If passing multiple values, must be comma separated. Non-integer values will result in a
422
error. - item_external_variant_idsstring
Filter
BundleSelections
by contents. If the Variant ID exists as a content of theBundleSelection
it will appear as a result.If passing multiple values, must be comma separated. Non-integer values will result in a
422
error. - item_external_product_idsstring
Filter
BundleSelections
by contents. If the Product ID exists as a content of theBundleSelection
it will appear as a result.If passing multiple values, must be comma separated. Non-integer values will result in a
422
error. - limitstring
Default: 50
Max: 250
The amount of results.
- pagestring*Deprecated
Default: 1
The page to show.
Page-based pagination has been deprecated but still available to use for pages up to a 100. If you need data past this point, use cursor pagination.
- sort_bystring
Sort listed
BundleSelections
in a specific order.
Available sort options:id-asc
,id-desc
,updated_at-asc
,updated_at-desc
. - active_purchase_itemsboolean
Bundle selections are not directly linked to Subscriptions. By default, the endpoint returns Bundle Selections, even those associated with cancelled subscriptions, unless the Bundle Selection has been deleted.
To exclude cancelled subscriptions from your results, includeactive_purchase_items=true
in your query.
More Parameters
Responses
- 200
successful response
Show response object
curl 'https://api.rechargeapps.com/bundle_selections' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d limit=3 -G
{
"next_cursor": null,
"previous_cursor": null,
"bundle_selections": [
{
"id": 100714428,
"bundle_variant": 382417,
"purchase_item_id": 199820883,
"created_at": "2022-08-26T18:31:28+00:00",
"external_product_id": "7121697210548",
"external_variant_id": "41450875650228",
"items": [
{
"id": 541,
"collection_id": "287569608884",
"collection_source": "shopify",
"created_at": "2022-08-26T18:31:28+00:00",
"external_product_id": "7121693671604",
"external_variant_id": "41450853105844",
"quantity": 3,
"updated_at": "2022-08-26T18:52:41+00:00"
}
],
"updated_at": "2022-08-26T18:31:28+00:00"
}
]
}
Retrieve a BundleSelection
using the bundle_selection_id
.
BundleSelections
endpoints are available for Recharge Pro merchants. If you’re interested in leveraging the Recharge bundle_selections
endpoints, reach out to your account manager or our Support team.
Learn more about Recharge Pro.
Responses
- 200
successful response
Show response object - 404
the
BundleSelection
withbundle_selection_id
does not existShow response object
curl 'https://api.rechargeapps.com/bundle_selections/100714428' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token'
{
"bundle_selection": {
"id": 100714428,
"bundle_variant": 382417,
"purchase_item_id": 199820883,
"created_at": "2022-08-26T18:31:28+00:00",
"external_product_id": "7121697210548",
"external_variant_id": "41450875650228",
"items": [
{
"id": 541,
"collection_id": "287569608884",
"collection_source": "shopify",
"created_at": "2022-08-26T18:31:28+00:00",
"external_product_id": "7121693671604",
"external_variant_id": "41450853105844",
"quantity": 3,
"updated_at": "2022-08-26T18:52:41+00:00"
}
],
"updated_at": "2022-08-26T18:31:28+00:00"
}
}
Create a BundleSelection
.
BundleSelections
endpoints are available for Recharge Pro merchants. If you’re interested in leveraging the Recharge bundle_selections
endpoints, reach out to your account manager or our Support team.
Learn more about Recharge Pro.
Body Parameters
- purchase_item_idinteger* Required
The ID of the
PurchaseItem
associated with theBundleSelection
. - plan_idinteger
A Recharge Plan ID. If provided in a dynamically-priced Bundle, the unit price of each product variant will be inferred from the plan associated with the ID; otherwise, it will be inferred from the plan with the greatest discount for each product in the selection. This is not used in fixed-priced Bundles.
- itemsarray* Required
A list of
item
objects, each containing information about a distinct product selected as part of the Bundle.Show object attributes
More Parameters
Responses
- 200
successful response
Show response object - 422
missing required field or invalid items passed
Show response object
curl 'https://api.rechargeapps.com/bundle_selections' \
-H 'X-Recharge-Version: 2021-11' \
-H 'Content-Type: application/json' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d '{
"purchase_item_id": 199820883,
"items": [
{
"collection_id": "287569608884",
"collection_source": "shopify",
"external_product_id": "7121693671604",
"external_variant_id": "41450853105844",
"quantity": 3
}
]
}'
{
"bundle_selection": {
"id": 100714428,
"bundle_variant": 382417,
"purchase_item_id": 199820883,
"created_at": "2022-08-26T18:31:28+00:00",
"external_product_id": "7121697210548",
"external_variant_id": "41450875650228",
"items": [
{
"id": 541,
"collection_id": "287569608884",
"collection_source": "shopify",
"created_at": "2022-08-26T18:31:28+00:00",
"external_product_id": "7121693671604",
"external_variant_id": "41450853105844",
"quantity": 3,
"updated_at": "2022-08-26T18:52:41+00:00"
}
],
"updated_at": "2022-08-26T18:31:28+00:00"
}
}
Modify an existing BundleSelection
to match the specified parameters.
BundleSelections
endpoints are available for Recharge Pro merchants. If you’re interested in leveraging the Recharge bundle_selections
endpoints, reach out to your account manager or our Support team.
Learn more about Recharge Pro.
Body Parameters
- purchase_item_idinteger
The ID of the
PurchaseItem
associated with theBundleSelection
. - plan_idinteger
A Recharge Plan ID. If provided in a dynamically-priced Bundle, the unit price of each product variant will be inferred from the plan associated with the ID; otherwise, it will be inferred from the plan with the greatest discount for each product in the selection. This is not used in fixed-priced Bundles.
- itemsarray
A list of
item
objects, each containing information about a distinct product selected as part of the Bundle.
More Parameters
Responses
- 200
successful response
Show response object - 404
the
BundleSelection
withbundle_selection_id
does not existShow response object - 422
invalid items passed
Show response object
curl -X PUT 'https://api.rechargeapps.com/bundle_selections/100714428' \
-H 'X-Recharge-Version: 2021-11' \
-H 'Content-Type: application/json' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d '{
"purchase_item_id": 199820883,
"items": [
{
"collection_id": "287569608884",
"collection_source": "shopify",
"external_product_id": "7121693671604",
"external_variant_id": "41450853105844",
"quantity": 3
}
]
}'
{
"bundle_selection": {
"id": 100714428,
"bundle_variant": 382417,
"purchase_item_id": 199820883,
"created_at": "2022-08-26T18:31:28+00:00",
"external_product_id": "7121697210548",
"external_variant_id": "41450875650228",
"items": [
{
"id": 541,
"collection_id": "287569608884",
"collection_source": "shopify",
"created_at": "2022-08-26T18:31:28+00:00",
"external_product_id": "7121693671604",
"external_variant_id": "41450853105844",
"quantity": 3,
"updated_at": "2022-08-26T18:52:41+00:00"
}
],
"updated_at": "2022-08-26T18:31:28+00:00"
}
}
Delete a BundleSelection
BundleSelections
endpoints are available for Recharge Pro merchants. If you’re interested in leveraging the Recharge bundle_selections
endpoints, reach out to your account manager or our Support team.
Learn more about Recharge Pro.
Responses
- 204
the
BundleSelection
has been removed and there is no content to send back in the response body.Show response object - 404
the
BundleSelection
withid
does not existShow response object
curl -X DELETE 'https://api.rechargeapps.com/bundle_selections/100714428' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token'
{}
Charges
A charge is the representation of a financial transaction linked to the purchase of an item (past or future). It can be a transaction that was processed already or the representation of an upcoming transaction. A Charge
is linked to its corresponding Orders
(one Order
for pay as you go subscriptions and several for pre-paid). Orders
are created once the corresponding Charge
is successful. After successful payment, the first Order
will be immediately submitted to the external platform if applicable (e.g. Shopify, BigCommerce).
The charge object
A Charge
is the representation of the financial transaction linked to a purchase (past or future). It can be a transaction that was processed already or the representation of an upcoming transaction. A charge is linked to its corresponding Orders
(one for pay as you go subscriptions and several for pre-paid).
A Charge
can have many parent subscriptions. All subscriptions on a given Address
with the same next_charge_date
date will be merged into one Charge
and that charge will contain one line_item
per Subscription
.
Attributes
- idinteger
The unique numeric identifier for the
Charge
. - address_idinteger
The ID of the shipping
Address
tied to theCharge
. - analytics_dataobject
An object containing analytics data associated with the
Charge
.Show object attributes - billing_addressobject
All the billing information related to the charge.
Show object attributes - client_detailsobject
Details of the access method used by the purchaser.
Show object attributes - created_atdatetime
The date and time when the transaction was created.
- currencystring
The code of the currency for this
Charge
, such asUSD
.Related guides: Supported currencies
- customerobject
An object containing
Customer
information associated with thisCharge
.Show object attributes - discountsarray
An array of
Discounts
associated with theCharge
.Show object attributes - external_order_idobject
An object containing the associated external order ID.
Show object attributes - external_transaction_idobject
An object containing the associated external transaction ID.
Show object attributes - line_itemsarray
A list of
line_item
objects, each containing information about a distinct purchase item.Show object attributes - notestring
Notes associated with the
Charge
. - order_attributesarray
An array of name-value pairs of order attributes on the
Charge
.Show object attributes - orders_countinteger
The number of
Orders
generated from thisCharge
(>1 for prepaidSubscriptions
). - payment_processorstring
The payment processor used for this
Charge
. - processed_atdatetime
The date and time when the transaction was processed.
- scheduled_atdate
The date time of when the
Charge
is/was scheduled to process. - shipping_addressobject
The shipping
Address
of theCharge
.Show object attributes - shipping_linesarray
An array of shipping lines associated with the
Charge
.Show object attributes - statusstring
Possible values: success, error, queued, skipped, refunded, partially_refunded, pending_manual_payment, pending
The status of the
Charge
. - subtotal_pricestring
The combined price of all
line_items
without taxes and shipping. - tagsstring
A comma-separated list of tags on the
Charge
. - tax_linesarray
An array of tax lines that apply to the
Charge
.Show object attributes - taxableboolean
A boolean indicator of the taxability of the
Charge
. - taxes_includedboolean
Whether taxes are included in the order subtotal.
- total_discountsstring
The sum of the
Discounts
applied to theCharge
. - total_line_items_pricestring
The total price of all line items of the
Charge
. - total_pricestring
The sum of all the prices of all the items in the
Charge
, taxes and discounts included (must be positive). - total_refundsstring
The sum of all refunds that were applied to the
Charge
. - total_taxstring
The total tax due associated with the
Charge
. - total_weight_gramsinteger
The total weight of the
Charge
’s line items in grams. - typestring
Possible values: checkout, recurring
An indicator of the
Charge
’s type, either checkout or recurring. - updated_atdatetime
The date time at which the
Charge
was most recently updated.
Error related attributes
- errorstring
Error reason as sentence text (typically returned direct from the payment processor). e.g.
"error": "Customer needs to update credit card"
- error_typestring
Structured reason why the charge failed such as
CUSTOMER_NEEDS_TO_UPDATE_CARD
. - charge_attemptsinteger
Shows how many times an attempt to charge was placed.
- external_variant_id_not_foundboolean
Indicates if Recharge was able to find the
external_variant_id
from theCharge
. - retry_datedate
The date when the next attempt will be placed.
More Attributes
- has_uncommited_changesboolean
Specifies whether the
Charge
is scheduled for a regeneration (if theSubscription
related to the charge was updated in the last 5 seconds usingcommit=false
).
{
"charge": {
"id": 100714428,
"address_id": 21317826,
"analytics_data": {
"utm_params": [
{
"utm_campaign": "spring_sale",
"utm_content": "differentiate-content",
"utm_data_source": "cookie",
"utm_medium": "email",
"utm_source": "newsletter",
"utm_term": "test-term",
"utm_time_stamp": "2019-12-16T23:57:28.752Z"
}
]
},
"billing_address": {
"address1": "3030 Nebraska Avenue",
"address2": null,
"city": "Los Angeles",
"company": null,
"country_code": "US",
"first_name": "Mike",
"last_name": "Flynn",
"phone": "3103843698",
"province": "California",
"zip": "90404"
},
"client_details": {
"browser_ip": "192.168.0.1",
"user_agent": "safari webkit"
},
"created_at": "2018-11-14T09:45:44+00:00",
"currency": "USD",
"customer": {
"id": 12345,
"email": "test@test.com",
"external_customer_id": {
"ecommerce": "2879413682227"
},
"hash": "7e706455cbd13e40"
},
"discounts": [
{
"id": 12345,
"code": "10DOLLAROFF",
"value": 10,
"value_type": "fixed_amount"
}
],
"error": null,
"error_type": null,
"external_order_id": {
"ecommerce": "2541635698739"
},
"external_transaction_id": {
"payment_processor": "ch_XXXXXXXXXXXXXXX"
},
"has_uncommitted_changes": false,
"line_items": [
{
"purchase_item_id": 63898947,
"external_product_id": {
"ecommerce": "4381728735283"
},
"external_variant_id": {
"ecommerce": "99999999999"
},
"grams": 4536,
"images": {
"large": "https://cdn.shopify.com/s/files/1/0175/0695/9460/products/Sumatra_Coffee_large.png",
"medium": "https://cdn.shopify.com/s/files/1/0175/0695/9460/products/Sumatra_Coffee__medium.png",
"original": "https://cdn.shopify.com/s/files/1/0175/0695/9460/products/Sumatra_Coffee_.png",
"small": "https://cdn.shopify.com/s/files/1/0175/0695/9460/products/Sumatra_Coffee__small.png"
},
"original_price": "12.00",
"properties": [
{
"name": "grind",
"value": "drip"
}
],
"purchase_item_type": "subscription",
"quantity": 1,
"sku": "MILK-1",
"tax_due": "1.14",
"tax_lines": [
{
"price": "0.870",
"rate": 0.0725,
"title": "CA State Tax"
},
{
"price": "0.270",
"rate": 0.0225,
"title": "Los Angeles County Tax"
}
],
"taxable": true,
"taxable_amount": "12.00",
"title": "Sumatra Coffee",
"total_price": "13.14",
"unit_price": "12.00",
"variant_title": "Milk - a / b"
}
],
"note": "next order #1",
"order_attributes": [
{
"name": "custom name",
"value": "custom value"
}
],
"processor_name": "stripe",
"scheduled_at": "2018-12-12",
"shipping_address": {
"address1": "3030 Nebraska Avenue",
"address2": "",
"city": "Los Angeles",
"company": "Recharge",
"country": "United States",
"first_name": "Mike",
"last_name": "Flynn",
"phone": "3103843698",
"province": "California",
"zip": "90404"
},
"shipping_lines": [
{
"code": "Standard Shipping",
"price": "0.00",
"title": "Standard Shipping"
}
],
"status": "queued",
"subtotal_price": "12.00",
"tags": "Subscription",
"tax_lines": [
{
"price": "0.950",
"rate": 0.0725,
"title": "CA State Tax"
},
{
"price": "0.335",
"rate": 0.0225,
"title": "Los Angeles County Tax"
}
],
"taxes_included": true,
"total_discounts": "0.00",
"total_line_items_price": "12.00",
"total_price": "13.14",
"total_refunds": null,
"total_tax": "1.14",
"total_weight_grams": 4536,
"type": "recurring",
"updated_at": "2018-11-14T09:45:44+00:00"
}
}
Retrieve a charge
Retrieve a Charge
using the charge_id
.
Starting March 19th, 2025, processed charges (those where status equals success, refunded, or partially-refunded) that have a value for processed_at greater than 90 days in the past will no longer appear in responses. As a result, you may receive an error.
Charge data processed over 90 days ago will remain available through the Exports tool in the Recharge merchant portal and within the Merchant portal UI.
Example of unaffected API calls:
[RETRIEVE A CHARGE] /charges/{id}
(if it’s a charge that does not have a processed_at date or the processed_at date is within the last 90 days)
Example of API calls that will result in an error:
[RETRIEVE A CHARGE] /charges/{id}
(if it’s a charge with a processed_at date older than 90 days)
Responses
- 200
successful response
Show response object
curl 'https://api.rechargeapps.com/charges/377749210' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token'
{
"charge": {
"id": 377749210,
"address_id": 42171447,
"analytics_data": {
"utm_params": [
{
"utm_source": "facebook",
"utm_medium": "cpc"
}
]
},
"billing_address": {
"address1": "601 SW Washing St.",
"address2": "Suite 101",
"city": "Portland",
"company": "Acme Corp",
"country_code": "US",
"first_name": "Jane",
"last_name": "Doe",
"phone": "888-888-8888",
"province": "Oregon",
"zip": "97205"
},
"client_details": {
"browser_ip": "192.168.0.1",
"user_agent": "safari webkit"
},
"created_at": "2021-11-09T19:22:13+00:00",
"currency": "USD",
"customer": {
"id": 37657002,
"email": "test@test.com",
"external_customer_id": {
"ecommerce": "2879413682227"
},
"hash": "7e706455cbd13e40"
},
"discounts": [
{
"id": 12345,
"code": "TESTCODE10",
"value": 10,
"value_type": "fixed_amount"
}
],
"error": null,
"error_type": null,
"external_order_id": {
"ecommerce": "2541635698739"
},
"external_transaction_id": {
"payment_processor": "ch_1HzWElJ2zqHvZRd1TWKFFqDR"
},
"has_uncommitted_changes": false,
"line_items": [
{
"purchase_item_id": 63898947,
"external_product_id": {
"ecommerce": "4381728735283"
},
"external_variant_id": {
"ecommerce": "999999999999"
},
"grams": 454,
"handle": "shirt-package",
"images": {
"large": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h_large.jpg",
"medium": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h_medium.jpg",
"original": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h.jpg",
"small": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h_small.jpg"
},
"original_price": "10.00",
"properties": [
{
"name": "Color",
"value": "Blue"
}
],
"purchase_item_type": "subscription",
"quantity": 1,
"sku": "TOM0001",
"tax_due": "1.30",
"tax_lines": [
{
"price": "0.9931",
"rate": "0.0725",
"title": "CA State Tax",
"unit_price": "0.3310"
},
{
"price": "0.3082",
"rate": "0.0225",
"title": "LA County Tax",
"unit_price": "0.1027"
}
],
"taxable": true,
"taxable_amount": "10.00",
"title": "Shirt package",
"total_price": "11.30",
"unit_price": "10.00",
"unit_price_includes_tax": false,
"variant_title": "Blue T-shirt"
}
],
"note": "next order in sequence 3",
"order_attributes": [
{
"name": "Checkout-Method",
"value": "delivery"
}
],
"orders_count": 1,
"payment_processor": "stripe",
"processed_at": "2021-11-09T23:59:31+00:00",
"retry_date": "2021-01-01",
"scheduled_at": "2021-12-09",
"shipping_address": {
"address1": "1030 Barnum Ave",
"address2": "Suite 101",
"city": "Stratford",
"company": "Fake Company",
"country_code": "US",
"first_name": "Fake First",
"last_name": "Fake Last",
"phone": "999-999-9999",
"province": "Connecticut",
"zip": "06614"
},
"shipping_lines": [
{
"code": "Standard",
"price": "4.90",
"source": "shopify",
"taxable": true,
"tax_lines": [
{
"price": "0.355",
"rate": 0.0725,
"title": "CA State Tax"
},
{
"price": "0.110",
"rate": 0.0225,
"title": "Los Angeles County Tax"
}
],
"title": "Standard"
}
],
"status": "success",
"subtotal_price": "10.00",
"tags": "Subscription, Subscription Recurring Order",
"tax_lines": [
{
"price": "0.950",
"rate": 0.0725,
"title": "CA State Tax"
},
{
"price": "0.335",
"rate": 0.0225,
"title": "Los Angeles County Tax"
}
],
"taxable": true,
"taxes_included": true,
"total_discounts": "10.0",
"total_line_items_price": "10.00",
"total_price": "11.29",
"total_refunds": "0.00",
"total_tax": "1.29",
"total_weight_grams": 454,
"type": "recurring",
"updated_at": "2021-11-09T23:59:32+00:00"
}
}
List charges
Returns a list of Charges
.
HTTP request examples
GET /charges?address_id=:address_id
GET /charges?created_at_min=2016-05-18&created_at_max=2016-06-18
GET /charges?customer_id=:customer_id
GET /charges?discount_code=10PERCENTOFF
GET /charges?discount_id=:discount_id
GET /charges?external_order_id=:external_order_id
GET /charges?ids=1123551,262667345,12341535
GET /charges?purchase_item_id=:purchase_item_id
GET /charges?scheduled_at=2016-06-18
GET /charges?scheduled_at_min=2016-05-18&scheduled_at_max=2016-06-18
GET /charges?sort_by=id-desc
GET /charges?status=queued
GET /charges?status=queued,refunded,partially_refunded
GET /charges?updated_at_min=2016-05-18&updated_at_max=2016-06-18
GET /charges?processed_at_min=2022-01-18&processed_at_max=2022-02-18
Starting March 19th, 2025, processed charges (those where status equals success, refunded, or partially- refunded) that have a value for processed_at greater than 90 days in the past will no longer appear in responses. As a result, you may receive a partial data set or an empty list.
Charge data processed over 90 days ago will remain available through the Exports tool in the Recharge merchant portal and within the Merchant portal UI.
Examples of unaffected API calls:
[LIST CHARGES] /charges?status=queued
[LIST CHARGES] /charges?status=error
Examples of API calls that may return partial results:
[LIST CHARGES] /charges?processed_at_min=2024-01-01
(this would only return results that have a processed_at date in the last 90 days)
[LIST CHARGES] /charges?status=success,queued
(this would return all queued charges but only return success charges that have a processed_at date in the last 90 days)
Example of API calls that will result in an empty list:
[LIST CHARGES] /charges?processed_at_max=2024-01-01
(any date over 90 days old)
Reminder: returned charges are sorted ascending by id value by default.
Query Parameters
- address_idstring
Filter
Charges
byAddress
. - created_at_maxstring
Show
Charges
created before the given date. - created_at_minstring
Show
Charges
created after the given date. - customer_idstring
Filter
Charges
byCustomer
. - discount_codestring
List
Charges
that contain the givendiscount_code
. - discount_idstring
List
Charges
that contain the givendiscount_id
. - external_order_idstring
Filter
Charges
by the associated order ID in the external e-commerce platform. - idsstring
Filter
Charges
by ID.If passing multiple values, must be comma separated. Non-integer values will result in a
422
error. - limitstring
Default: 50
Max: 250
The amount of results.
- pagestring*Deprecated
Default: 1
The page to show.
Page-based pagination has been deprecated but still available to use for pages up to a 100. If you need data past this point, use cursor pagination.
- purchase_item_idstring
Filter
Charges
by aSubscription
orOnetime
ID. - purchase_item_idsstring
Filter
Charges
by a comma-separated list ofSubscription
orOnetime
IDs. - scheduled_atstring
Filter
Charges
by specific scheduled charge date. - scheduled_at_maxstring
Show
Charges
scheduled to be processed before the given date. - scheduled_at_minstring
Show
Charges
scheduled to be processed after the given date. - sort_bystring
Sort listed
Charges
in a specific order.
Available sort options:id-asc
,id-desc
,updated_at-asc
,updated_at-desc
,scheduled_at-asc
,scheduled_at-desc
. - statusstring
Filter charges by status.
Available status:success
,queued
,error
,refunded
,partially_refunded
,skipped
,pending_manual_payment
,pending
. - updated_at_maxstring
Show charges updated before the given date.
- updated_at_minstring
Show charges updated after the given date.
- processed_at_minstring
Show charges processed after, and including, the given date.
- processed_at_maxstring
Show charges processed before, and including, the given date.
More Parameters
Responses
- 200
successful response
Show response object
curl 'https://api.rechargeapps.com/charges' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d limit=3 -G
{
"next": "next_cursor",
"previous": "previous_cursor",
"charges": [
{
"id": 377749210,
"address_id": 42171447,
"analytics_data": {
"utm_params": [
{
"utm_source": "facebook",
"utm_medium": "cpc"
}
]
},
"billing_address": {
"address1": "601 SW Washing St.",
"address2": "Suite 101",
"city": "Portland",
"company": "Acme Corp",
"country_code": "US",
"first_name": "Jane",
"last_name": "Doe",
"phone": "888-888-8888",
"province": "Oregon",
"zip": "97205"
},
"client_details": {
"browser_ip": "192.168.0.1",
"user_agent": "safari webkit"
},
"created_at": "2021-11-09T19:22:13+00:00",
"currency": "USD",
"customer": {
"id": 37657002,
"email": "test@test.com",
"external_customer_id": {
"ecommerce": "2879413682227"
},
"hash": "7e706455cbd13e40"
},
"discounts": [
{
"id": 12345,
"code": "TESTCODE10",
"value": 10,
"value_type": "fixed_amount"
}
],
"error": null,
"error_type": null,
"external_order_id": {
"ecommerce": "2541635698739"
},
"external_transaction_id": {
"payment_processor": "ch_1HzWElJ2zqHvZRd1TWKFFqDR"
},
"has_uncommitted_changes": false,
"line_items": [
{
"purchase_item_id": 63898947,
"external_product_id": {
"ecommerce": "4381728735283"
},
"external_variant_id": {
"ecommerce": "999999999999"
},
"grams": 454,
"handle": "shirt-package",
"images": {
"large": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h_large.jpg",
"medium": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h_medium.jpg",
"original": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h.jpg",
"small": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h_small.jpg"
},
"original_price": "10.00",
"properties": [
{
"name": "Color",
"value": "Blue"
}
],
"purchase_item_type": "subscription",
"quantity": 1,
"sku": "TOM0001",
"tax_due": "1.30",
"tax_lines": [
{
"price": "0.9931",
"rate": "0.0725",
"title": "CA State Tax",
"unit_price": "0.3310"
},
{
"price": "0.3082",
"rate": "0.0225",
"title": "LA County Tax",
"unit_price": "0.1027"
}
],
"taxable": true,
"taxable_amount": "10.00",
"title": "Shirt package",
"total_price": "11.30",
"unit_price": "10.00",
"unit_price_includes_tax": false,
"variant_title": "Blue T-shirt"
}
],
"note": "next order in sequence 3",
"order_attributes": [
{
"name": "Checkout-Method",
"value": "delivery"
}
],
"orders_count": 1,
"payment_processor": "stripe",
"processed_at": "2021-11-09T23:59:31+00:00",
"retry_date": "2021-01-01",
"scheduled_at": "2021-12-09",
"shipping_address": {
"address1": "1030 Barnum Ave",
"address2": "Suite 101",
"city": "Stratford",
"company": "Fake Company",
"country_code": "US",
"first_name": "Fake First",
"last_name": "Fake Last",
"phone": "999-999-9999",
"province": "Connecticut",
"zip": "06614"
},
"shipping_lines": [
{
"code": "Standard",
"price": "4.90",
"source": "shopify",
"taxable": true,
"tax_lines": [
{
"price": "0.355",
"rate": 0.0725,
"title": "CA State Tax"
},
{
"price": "0.110",
"rate": 0.0225,
"title": "Los Angeles County Tax"
}
],
"title": "Standard"
}
],
"status": "success",
"subtotal_price": "10.00",
"tags": "Subscription, Subscription Recurring Order",
"tax_lines": [
{
"price": "0.950",
"rate": 0.0725,
"title": "CA State Tax"
},
{
"price": "0.335",
"rate": 0.0225,
"title": "Los Angeles County Tax"
}
],
"taxable": true,
"taxes_included": true,
"total_discounts": "10.0",
"total_line_items_price": "10.00",
"total_price": "11.29",
"total_refunds": "0.00",
"total_tax": "1.29",
"total_weight_grams": 454,
"type": "recurring",
"updated_at": "2021-11-09T23:59:32+00:00"
}
]
}
Apply a discount
Endpoint for adding Discount
to an existing queued Charge
.
You cannot add a Discount
to an existing queued Charge
if the Charge
or the associated Address
already has one.
You can provide either discount_id
or discount_code
. If both parameters are passed, the value for discount_id
will take precedence.
If a Charge
has a Discount
and it gets updated, or a regeneration occurs, the Discount
will be lost. Regeneration is a process that refreshes the Charge
JSON with new data in the case of the Subscription
or Address
being updated.
Body Parameters
- discount_codestring
Code of the
Discount
you want to apply to aCharge
. - discount_idinteger
ID of the
Discount
you want to apply to aCharge
.
More Parameters
Responses
- 200
successful response
Show response object
curl 'https://api.rechargeapps.com/charges/105805051/apply_discount' \
-H 'Content-Type: application/json' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d '{"discount_code": "test"}'
{
"charge": {
"id": 377749210,
"address_id": 42171447,
"analytics_data": {
"utm_params": [
{
"utm_source": "facebook",
"utm_medium": "cpc"
}
]
},
"billing_address": {
"address1": "601 SW Washing St.",
"address2": "Suite 101",
"city": "Portland",
"company": "Acme Corp",
"country_code": "US",
"first_name": "Jane",
"last_name": "Doe",
"phone": "888-888-8888",
"province": "Oregon",
"zip": "97205"
},
"client_details": {
"browser_ip": "192.168.0.1",
"user_agent": "safari webkit"
},
"created_at": "2021-11-09T19:22:13+00:00",
"currency": "USD",
"customer": {
"id": 37657002,
"email": "test@test.com",
"external_customer_id": {
"ecommerce": "2879413682227"
},
"hash": "7e706455cbd13e40"
},
"discounts": [
{
"id": 12345,
"code": "TESTCODE10",
"value": 10,
"value_type": "fixed_amount"
}
],
"error": null,
"error_type": null,
"external_order_id": {
"ecommerce": "2541635698739"
},
"external_transaction_id": {
"payment_processor": "ch_1HzWElJ2zqHvZRd1TWKFFqDR"
},
"has_uncommitted_changes": false,
"line_items": [
{
"purchase_item_id": 63898947,
"external_product_id": {
"ecommerce": "4381728735283"
},
"external_variant_id": {
"ecommerce": "999999999999"
},
"grams": 454,
"handle": "shirt-package",
"images": {
"large": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h_large.jpg",
"medium": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h_medium.jpg",
"original": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h.jpg",
"small": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h_small.jpg"
},
"original_price": "10.00",
"properties": [
{
"name": "Color",
"value": "Blue"
}
],
"purchase_item_type": "subscription",
"quantity": 1,
"sku": "TOM0001",
"tax_due": "1.30",
"tax_lines": [
{
"price": "0.9931",
"rate": "0.0725",
"title": "CA State Tax",
"unit_price": "0.3310"
},
{
"price": "0.3082",
"rate": "0.0225",
"title": "LA County Tax",
"unit_price": "0.1027"
}
],
"taxable": true,
"taxable_amount": "10.00",
"title": "Shirt package",
"total_price": "11.30",
"unit_price": "10.00",
"unit_price_includes_tax": false,
"variant_title": "Blue T-shirt"
}
],
"note": "next order in sequence 3",
"order_attributes": [
{
"name": "Checkout-Method",
"value": "delivery"
}
],
"orders_count": 1,
"payment_processor": "stripe",
"processed_at": "2021-11-09T23:59:31+00:00",
"retry_date": "2021-01-01",
"scheduled_at": "2021-12-09",
"shipping_address": {
"address1": "1030 Barnum Ave",
"address2": "Suite 101",
"city": "Stratford",
"company": "Fake Company",
"country_code": "US",
"first_name": "Fake First",
"last_name": "Fake Last",
"phone": "999-999-9999",
"province": "Connecticut",
"zip": "06614"
},
"shipping_lines": [
{
"code": "Standard",
"price": "4.90",
"source": "shopify",
"taxable": true,
"tax_lines": [
{
"price": "0.355",
"rate": 0.0725,
"title": "CA State Tax"
},
{
"price": "0.110",
"rate": 0.0225,
"title": "Los Angeles County Tax"
}
],
"title": "Standard"
}
],
"status": "success",
"subtotal_price": "10.00",
"tags": "Subscription, Subscription Recurring Order",
"tax_lines": [
{
"price": "0.950",
"rate": 0.0725,
"title": "CA State Tax"
},
{
"price": "0.335",
"rate": 0.0225,
"title": "Los Angeles County Tax"
}
],
"taxable": true,
"total_discounts": "10.0",
"total_line_items_price": "10.00",
"total_price": "11.29",
"total_refunds": "0.00",
"total_tax": "1.29",
"total_weight_grams": 454,
"type": "recurring",
"updated_at": "2021-11-09T23:59:32+00:00"
}
}
Remove a discount
Remove a Discount
from a Charge
without destroying the Discount
.
HTTP request examples
POST /charges/<charge_id>/remove_discount/
In most cases the Discount
should be removed from the Address
. When the Discount
is removed from the Address
, the Discount
is also removed from any future Charges
.
If the Discount
is on the parent Address
, you cannot remove it using charge_id
. When removing your Discount
, it is preferable to pass the address_id
so that the Discount
stays removed if the Charge
is regenerated. Only pass charge_id
in edge cases in which there are two or more Charges
on a parent Address
and you only want to remove the Discount
from one Charge
. If you pass both parameters, it will remove the Discount
from the Address
.
Responses
- 200
successful response
Show response object
curl 'https://api.rechargeapps.com/charges/459904607/remove_discount' \
-H 'Content-Type: application/json' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d '{}'
{
"charge": {
"id": 377749210,
"address_id": 42171447,
"analytics_data": {
"utm_params": [
{
"utm_source": "facebook",
"utm_medium": "cpc"
}
]
},
"billing_address": {
"address1": "601 SW Washing St.",
"address2": "Suite 101",
"city": "Portland",
"company": "Acme Corp",
"country_code": "US",
"first_name": "Jane",
"last_name": "Doe",
"phone": "888-888-8888",
"province": "Oregon",
"zip": "97205"
},
"client_details": {
"browser_ip": "192.168.0.1",
"user_agent": "safari webkit"
},
"created_at": "2021-11-09T19:22:13+00:00",
"currency": "USD",
"customer": {
"id": 37657002,
"email": "test@test.com",
"external_customer_id": {
"ecommerce": "2879413682227"
},
"hash": "7e706455cbd13e40"
},
"discounts": [],
"error": null,
"error_type": null,
"external_order_id": {
"ecommerce": "2541635698739"
},
"external_transaction_id": {
"payment_processor": "ch_1HzWElJ2zqHvZRd1TWKFFqDR"
},
"has_uncommitted_changes": false,
"line_items": [
{
"purchase_item_id": 63898947,
"external_product_id": {
"ecommerce": "4381728735283"
},
"external_variant_id": {
"ecommerce": "999999999999"
},
"grams": 454,
"handle": "shirt-package",
"images": {
"large": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h_large.jpg",
"medium": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h_medium.jpg",
"original": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h.jpg",
"small": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h_small.jpg"
},
"original_price": "10.00",
"properties": [
{
"name": "Color",
"value": "Blue"
}
],
"purchase_item_type": "subscription",
"quantity": 1,
"sku": "TOM0001",
"tax_due": "1.30",
"tax_lines": [
{
"price": "0.9931",
"rate": "0.0725",
"title": "CA State Tax",
"unit_price": "0.3310"
},
{
"price": "0.3082",
"rate": "0.0225",
"title": "LA County Tax",
"unit_price": "0.1027"
}
],
"taxable": true,
"taxable_amount": "10.00",
"title": "Shirt package",
"total_price": "11.30",
"unit_price": "10.00",
"unit_price_includes_tax": false,
"variant_title": "Blue T-shirt"
}
],
"note": "next order in sequence 3",
"order_attributes": [
{
"name": "Checkout-Method",
"value": "delivery"
}
],
"orders_count": 1,
"payment_processor": "stripe",
"processed_at": "2021-11-09T23:59:31+00:00",
"retry_date": "2021-01-01",
"scheduled_at": "2021-12-09",
"shipping_address": {
"address1": "1030 Barnum Ave",
"address2": "Suite 101",
"city": "Stratford",
"company": "Fake Company",
"country_code": "US",
"first_name": "Fake First",
"last_name": "Fake Last",
"phone": "999-999-9999",
"province": "Connecticut",
"zip": "06614"
},
"shipping_lines": [
{
"code": "Standard",
"price": "4.90",
"source": "shopify",
"taxable": true,
"tax_lines": [
{
"price": "0.355",
"rate": 0.0725,
"title": "CA State Tax"
},
{
"price": "0.110",
"rate": 0.0225,
"title": "Los Angeles County Tax"
}
],
"title": "Standard"
}
],
"status": "success",
"subtotal_price": "10.00",
"tags": "Subscription, Subscription Recurring Order",
"tax_lines": [
{
"price": "0.950",
"rate": 0.0725,
"title": "CA State Tax"
},
{
"price": "0.335",
"rate": 0.0225,
"title": "Los Angeles County Tax"
}
],
"taxable": true,
"total_discounts": "10.0",
"total_line_items_price": "10.00",
"total_price": "11.29",
"total_refunds": "0.00",
"total_tax": "1.29",
"total_weight_grams": 454,
"type": "recurring",
"updated_at": "2021-11-09T23:59:32+00:00"
}
}
Skip a charge
Skip a Charge
.
Responses
- 200
successful response
Show response object
curl 'https://api.rechargeapps.com/charges/377749210/skip' \
-H 'X-Recharge-Version: 2021-11' \
-H 'Content-Type: application/json' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d '{"purchase_item_ids": [27363808, 27363809]}'
{
"charge": {
"id": 377749210,
"address_id": 42171447,
"analytics_data": {
"utm_params": [
{
"utm_source": "facebook",
"utm_medium": "cpc"
}
]
},
"billing_address": {
"address1": "601 SW Washing St.",
"address2": "Suite 101",
"city": "Portland",
"company": "Acme Corp",
"country_code": "US",
"first_name": "Jane",
"last_name": "Doe",
"phone": "888-888-8888",
"province": "Oregon",
"zip": "97205"
},
"client_details": {
"browser_ip": "192.168.0.1",
"user_agent": "safari webkit"
},
"created_at": "2021-11-09T19:22:13+00:00",
"currency": "USD",
"customer": {
"id": 37657002,
"email": "test@test.com",
"external_customer_id": {
"ecommerce": "2879413682227"
},
"hash": "7e706455cbd13e40"
},
"discounts": [
{
"id": 12345,
"code": "TESTCODE10",
"value": 10,
"value_type": "fixed_amount"
}
],
"error": null,
"error_type": null,
"external_order_id": {
"ecommerce": "2541635698739"
},
"external_transaction_id": {
"payment_processor": "ch_1HzWElJ2zqHvZRd1TWKFFqDR"
},
"has_uncommitted_changes": false,
"line_items": [
{
"purchase_item_id": 63898947,
"external_product_id": {
"ecommerce": "4381728735283"
},
"external_variant_id": {
"ecommerce": "999999999999"
},
"grams": 454,
"handle": "shirt-package",
"images": {
"large": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h_large.jpg",
"medium": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h_medium.jpg",
"original": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h.jpg",
"small": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h_small.jpg"
},
"original_price": "10.00",
"properties": [
{
"name": "Color",
"value": "Blue"
}
],
"purchase_item_type": "subscription",
"quantity": 1,
"sku": "TOM0001",
"tax_due": "1.30",
"tax_lines": [
{
"price": "0.9931",
"rate": "0.0725",
"title": "CA State Tax",
"unit_price": "0.3310"
},
{
"price": "0.3082",
"rate": "0.0225",
"title": "LA County Tax",
"unit_price": "0.1027"
}
],
"taxable": true,
"taxable_amount": "10.00",
"title": "Shirt package",
"total_price": "11.30",
"unit_price": "10.00",
"unit_price_includes_tax": false,
"variant_title": "Blue T-shirt"
}
],
"note": "next order in sequence 3",
"order_attributes": [
{
"name": "Checkout-Method",
"value": "delivery"
}
],
"orders_count": 1,
"payment_processor": "stripe",
"processed_at": null,
"retry_date": null,
"scheduled_at": "2021-12-09",
"shipping_address": {
"address1": "1030 Barnum Ave",
"address2": "Suite 101",
"city": "Stratford",
"company": "Fake Company",
"country_code": "US",
"first_name": "Fake First",
"last_name": "Fake Last",
"phone": "999-999-9999",
"province": "Connecticut",
"zip": "06614"
},
"shipping_lines": [
{
"code": "Standard",
"price": "4.90",
"source": "shopify",
"taxable": true,
"tax_lines": [
{
"price": "0.355",
"rate": 0.0725,
"title": "CA State Tax"
},
{
"price": "0.110",
"rate": 0.0225,
"title": "Los Angeles County Tax"
}
],
"title": "Standard"
}
],
"status": "skipped",
"subtotal_price": "10.00",
"tags": "Subscription, Subscription Recurring Order",
"tax_lines": [
{
"price": "0.950",
"rate": 0.0725,
"title": "CA State Tax"
},
{
"price": "0.335",
"rate": 0.0225,
"title": "Los Angeles County Tax"
}
],
"taxable": true,
"total_discounts": "10.0",
"total_line_items_price": "10.00",
"total_price": "11.29",
"total_refunds": "0.00",
"total_tax": "1.29",
"total_weight_grams": 454,
"type": "recurring",
"updated_at": "2021-11-09T23:59:32+00:00"
}
}
Unskip a charge
Unskip a Charge
.
Responses
- 200
successful response
Show response object - 422
Unprocessable
Show response object
curl 'https://api.rechargeapps.com/charges/377749210/unskip' \
-H 'X-Recharge-Version: 2021-11' \
-H 'Content-Type: application/json' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d '{"purchase_item_ids": [27363808]}'
{
"charge": {
"id": 377749210,
"address_id": 42171447,
"analytics_data": {
"utm_params": [
{
"utm_source": "facebook",
"utm_medium": "cpc"
}
]
},
"billing_address": {
"address1": "601 SW Washing St.",
"address2": "Suite 101",
"city": "Portland",
"company": "Acme Corp",
"country_code": "US",
"first_name": "Jane",
"last_name": "Doe",
"phone": "888-888-8888",
"province": "Oregon",
"zip": "97205"
},
"client_details": {
"browser_ip": "192.168.0.1",
"user_agent": "safari webkit"
},
"created_at": "2021-11-09T19:22:13+00:00",
"currency": "USD",
"customer": {
"id": 37657002,
"email": "test@test.com",
"external_customer_id": {
"ecommerce": "2879413682227"
},
"hash": "7e706455cbd13e40"
},
"discounts": [
{
"id": 12345,
"code": "TESTCODE10",
"value": 10,
"value_type": "fixed_amount"
}
],
"error": null,
"error_type": null,
"external_order_id": {
"ecommerce": "2541635698739"
},
"external_transaction_id": {
"payment_processor": "ch_1HzWElJ2zqHvZRd1TWKFFqDR"
},
"has_uncommitted_changes": false,
"line_items": [
{
"purchase_item_id": 63898947,
"external_product_id": {
"ecommerce": "4381728735283"
},
"external_variant_id": {
"ecommerce": "999999999999"
},
"grams": 454,
"handle": "shirt-package",
"images": {
"large": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h_large.jpg",
"medium": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h_medium.jpg",
"original": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h.jpg",
"small": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h_small.jpg"
},
"original_price": "10.00",
"properties": [
{
"name": "Color",
"value": "Blue"
}
],
"purchase_item_type": "subscription",
"quantity": 1,
"sku": "TOM0001",
"tax_due": "1.30",
"tax_lines": [
{
"price": "0.9931",
"rate": "0.0725",
"title": "CA State Tax",
"unit_price": "0.3310"
},
{
"price": "0.3082",
"rate": "0.0225",
"title": "LA County Tax",
"unit_price": "0.1027"
}
],
"taxable": true,
"taxable_amount": "10.00",
"title": "Shirt package",
"total_price": "11.30",
"unit_price": "10.00",
"unit_price_includes_tax": false,
"variant_title": "Blue T-shirt"
}
],
"note": "next order in sequence 3",
"order_attributes": [
{
"name": "Checkout-Method",
"value": "delivery"
}
],
"orders_count": 1,
"payment_processor": "stripe",
"processed_at": null,
"retry_date": null,
"scheduled_at": "2021-12-09",
"shipping_address": {
"address1": "1030 Barnum Ave",
"address2": "Suite 101",
"city": "Stratford",
"company": "Fake Company",
"country_code": "US",
"first_name": "Fake First",
"last_name": "Fake Last",
"phone": "999-999-9999",
"province": "Connecticut",
"zip": "06614"
},
"shipping_lines": [
{
"code": "Standard",
"price": "4.90",
"source": "shopify",
"taxable": true,
"tax_lines": [
{
"price": "0.355",
"rate": 0.0725,
"title": "CA State Tax"
},
{
"price": "0.110",
"rate": 0.0225,
"title": "Los Angeles County Tax"
}
],
"title": "Standard"
}
],
"status": "queued",
"subtotal_price": "10.00",
"tags": "Subscription, Subscription Recurring Order",
"tax_lines": [
{
"price": "0.950",
"rate": 0.0725,
"title": "CA State Tax"
},
{
"price": "0.335",
"rate": 0.0225,
"title": "Los Angeles County Tax"
}
],
"taxable": true,
"total_discounts": "10.0",
"total_line_items_price": "10.00",
"total_price": "11.29",
"total_refunds": "0.00",
"total_tax": "1.29",
"total_weight_grams": 454,
"type": "recurring",
"updated_at": "2021-11-09T23:59:32+00:00"
}
}
Refund a charge
Refund a Charge
.
After the POST
request, that particular Charge
will have status
parameter updated to refunded
or partially_refunded
depending on the value of the amount
parameter. If retry
is true
, error
and error_type
are required, the Charge
will have status
parameter updated to error
. This means a new transaction would occur if the charge dunning process succeeds again. This functionality is used when the order submission attempt on the remote platform failed after the transaction succeeded.
Body Parameters
- amountstring* Required
Amount of money that will be refunded. It can be fully or partially refunded.
- full_refundboolean
If this parameter has value
true
, theCharge
will be totally refunded. - retryboolean
If this parameter has value
true
andfull_refund
has valuetrue
, theCharge
will be retried. Thestatus
on theCharge
will be returned as “error”. - errorstring
If the
retry
parameter has valuetrue
, this value is required. Valid values are “insufficient_inventory”. - error_typestring
If the
retry
parameter has valuetrue
, this value is required.
More Parameters
Responses
- 200
successful response
Show response object
curl 'https://api.rechargeapps.com/charges/377749210/refund' \
-H 'X-Recharge-Version: 2021-11' \
-H 'Content-Type: application/json' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d '{"amount": 11.00}'
{
"charge": {
"id": 377749210,
"address_id": 42171447,
"analytics_data": {
"utm_params": [
{
"utm_source": "facebook",
"utm_medium": "cpc"
}
]
},
"billing_address": {
"address1": "601 SW Washing St.",
"address2": "Suite 101",
"city": "Portland",
"company": "Acme Corp",
"country_code": "US",
"first_name": "Jane",
"last_name": "Doe",
"phone": "888-888-8888",
"province": "Oregon",
"zip": "97205"
},
"client_details": {
"browser_ip": "192.168.0.1",
"user_agent": "safari webkit"
},
"created_at": "2021-11-09T19:22:13+00:00",
"currency": "USD",
"customer": {
"id": 37657002,
"email": "test@test.com",
"external_customer_id": {
"ecommerce": "2879413682227"
},
"hash": "7e706455cbd13e40"
},
"discounts": [
{
"id": 12345,
"code": "TESTCODE10",
"value": 10,
"value_type": "fixed_amount"
}
],
"error": null,
"error_type": null,
"external_order_id": {
"ecommerce": "2541635698739"
},
"external_transaction_id": {
"payment_processor": "ch_1HzWElJ2zqHvZRd1TWKFFqDR"
},
"has_uncommitted_changes": false,
"line_items": [
{
"purchase_item_id": 63898947,
"external_product_id": {
"ecommerce": "4381728735283"
},
"external_variant_id": {
"ecommerce": "999999999999"
},
"grams": 454,
"handle": "shirt-package",
"images": {
"large": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h_large.jpg",
"medium": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h_medium.jpg",
"original": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h.jpg",
"small": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h_small.jpg"
},
"original_price": "10.00",
"properties": [
{
"name": "Color",
"value": "Blue"
}
],
"purchase_item_type": "subscription",
"quantity": 1,
"sku": "TOM0001",
"tax_due": "1.30",
"tax_lines": [
{
"price": "0.9931",
"rate": "0.0725",
"title": "CA State Tax",
"unit_price": "0.3310"
},
{
"price": "0.3082",
"rate": "0.0225",
"title": "LA County Tax",
"unit_price": "0.1027"
}
],
"taxable": true,
"taxable_amount": "10.00",
"title": "Shirt package",
"total_price": "11.30",
"unit_price": "10.00",
"unit_price_includes_tax": false,
"variant_title": "Blue T-shirt"
}
],
"note": "next order in sequence 3",
"order_attributes": [
{
"name": "Checkout-Method",
"value": "delivery"
}
],
"orders_count": 1,
"payment_processor": "stripe",
"processed_at": "2021-11-09T23:59:31+00:00",
"retry_date": null,
"scheduled_at": "2021-12-09",
"shipping_address": {
"address1": "1030 Barnum Ave",
"address2": "Suite 101",
"city": "Stratford",
"company": "Fake Company",
"country_code": "US",
"first_name": "Fake First",
"last_name": "Fake Last",
"phone": "999-999-9999",
"province": "Connecticut",
"zip": "06614"
},
"shipping_lines": [
{
"code": "Standard",
"price": "4.90",
"source": "shopify",
"taxable": true,
"tax_lines": [
{
"price": "0.355",
"rate": 0.0725,
"title": "CA State Tax"
},
{
"price": "0.110",
"rate": 0.0225,
"title": "Los Angeles County Tax"
}
],
"title": "Standard"
}
],
"status": "refunded",
"subtotal_price": "10.00",
"tags": "Subscription, Subscription Recurring Order",
"tax_lines": [
{
"price": "0.950",
"rate": 0.0725,
"title": "CA State Tax"
},
{
"price": "0.335",
"rate": 0.0225,
"title": "Los Angeles County Tax"
}
],
"taxable": true,
"total_discounts": "10.0",
"total_line_items_price": "10.00",
"total_price": "11.29",
"total_refunds": "11.29",
"total_tax": "1.29",
"total_weight_grams": 454,
"type": "recurring",
"updated_at": "2021-11-09T23:59:32+00:00"
}
}
Process a charge
Pro only
The charge processing route can be used to process Charges
that are in a queued
or error
status.
Related guides: Charges FAQ
The /charges/{id}/process
endpoint is available to Recharge Pro merchants on a request basis. If you’re interested in leveraging the Recharge Charge
processing API, reach out to your account manager or our Support team.
Learn more about Recharge Pro.
Responses
- 200
successful response
Show response object
curl 'https://api.rechargeapps.com/charges/100714428/process' \
-H 'X-Recharge-Version: 2021-11' \
-H 'Content-Type: application/json' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d '{}'
{
"charge": {
"id": 377749210,
"address_id": 42171447,
"analytics_data": {
"utm_params": [
{
"utm_source": "facebook",
"utm_medium": "cpc"
}
]
},
"billing_address": {
"address1": "601 SW Washing St.",
"address2": "Suite 101",
"city": "Portland",
"company": "Acme Corp",
"country_code": "US",
"first_name": "Jane",
"last_name": "Doe",
"phone": "888-888-8888",
"province": "Oregon",
"zip": "97205"
},
"client_details": {
"browser_ip": "192.168.0.1",
"user_agent": "safari webkit"
},
"created_at": "2021-11-09T19:22:13+00:00",
"currency": "USD",
"customer": {
"id": 37657002,
"email": "test@test.com",
"external_customer_id": {
"ecommerce": "2879413682227"
},
"hash": "7e706455cbd13e40"
},
"discounts": [
{
"id": 12345,
"code": "TESTCODE10",
"value": 10,
"value_type": "fixed_amount"
}
],
"error": null,
"error_type": null,
"external_order_id": {
"ecommerce": "2541635698739"
},
"external_transaction_id": {
"payment_processor": "ch_1HzWElJ2zqHvZRd1TWKFFqDR"
},
"has_uncommitted_changes": false,
"line_items": [
{
"purchase_item_id": 63898947,
"external_product_id": {
"ecommerce": "4381728735283"
},
"external_variant_id": {
"ecommerce": "999999999999"
},
"grams": 454,
"handle": "shirt-package",
"images": {
"large": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h_large.jpg",
"medium": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h_medium.jpg",
"original": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h.jpg",
"small": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h_small.jpg"
},
"original_price": "10.00",
"properties": [
{
"name": "Color",
"value": "Blue"
}
],
"purchase_item_type": "subscription",
"quantity": 1,
"sku": "TOM0001",
"tax_due": "1.30",
"tax_lines": [
{
"price": "0.9931",
"rate": "0.0725",
"title": "CA State Tax",
"unit_price": "0.3310"
},
{
"price": "0.3082",
"rate": "0.0225",
"title": "LA County Tax",
"unit_price": "0.1027"
}
],
"taxable": true,
"taxable_amount": "10.00",
"title": "Shirt package",
"total_price": "11.30",
"unit_price": "10.00",
"unit_price_includes_tax": false,
"variant_title": "Blue T-shirt"
}
],
"note": "next order in sequence 3",
"order_attributes": [
{
"name": "Checkout-Method",
"value": "delivery"
}
],
"orders_count": 1,
"payment_processor": "stripe",
"processed_at": "2021-11-09T23:59:31+00:00",
"retry_date": null,
"scheduled_at": "2021-12-09",
"shipping_address": {
"address1": "1030 Barnum Ave",
"address2": "Suite 101",
"city": "Stratford",
"company": "Fake Company",
"country_code": "US",
"first_name": "Fake First",
"last_name": "Fake Last",
"phone": "999-999-9999",
"province": "Connecticut",
"zip": "06614"
},
"shipping_lines": [
{
"code": "Standard",
"price": "4.90",
"source": "shopify",
"taxable": true,
"tax_lines": [
{
"price": "0.355",
"rate": 0.0725,
"title": "CA State Tax"
},
{
"price": "0.110",
"rate": 0.0225,
"title": "Los Angeles County Tax"
}
],
"title": "Standard"
}
],
"status": "success",
"subtotal_price": "10.00",
"tags": "Subscription, Subscription Recurring Order",
"tax_lines": [
{
"price": "0.950",
"rate": 0.0725,
"title": "CA State Tax"
},
{
"price": "0.335",
"rate": 0.0225,
"title": "Los Angeles County Tax"
}
],
"taxable": true,
"total_discounts": "10.0",
"total_line_items_price": "10.00",
"total_price": "11.29",
"total_refunds": "0.00",
"total_tax": "1.29",
"total_weight_grams": 454,
"type": "recurring",
"updated_at": "2021-11-09T23:59:32+00:00"
}
}
Capture a charge
If you are leveraging the authorize/capture workflow with Recharge, the charge/{id}/capture_payment
endpoint is how to capture the funds of a previously authorized Charge
.
Capture Window
You can only capture payment on
Charges
that have been authorized within the last 7 days. This is a limitation of payment providers/financial institutions and Recharge cannot configure or override this limit. Any Charges
that are attempted to be captured beyond that 7 day window may result in an error indicating the Charge
cannot be captured. As a result, Recharge provides a
charge/uncaptured
Webhook
. If subscribed, this Webhook
will notify you of any Charges
that are not captured 6 days after authorization. Please refer to the webhooks section for more information.
The /charges/{id}/capture_payment
endpoint is available to Recharge Pro merchants in the Recharge Closed Beta group. If you’re interested in leveraging the Recharge capture_payment
endpoint, reach out to your account manager or our Support team.
Learn more about Recharge Pro.
Responses
- 200
Charge captured successfully
Show response object - 400
Bad Request
Show response object - 404
Not Found
Show response object - 422
Unprocessable
Show response object
curl 'https://api.rechargeapps.com/charges/100714428/capture_payment' \
-H 'Content-Type: application/json' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d '{}'
{
"charge": {
"id": 377749210,
"address_id": 42171447,
"analytics_data": {
"utm_params": [
{
"utm_source": "facebook",
"utm_medium": "cpc"
}
]
},
"billing_address": {
"address1": "601 SW Washing St.",
"address2": "Suite 101",
"city": "Portland",
"company": "Acme Corp",
"country_code": "US",
"first_name": "Jane",
"last_name": "Doe",
"phone": "888-888-8888",
"province": "Oregon",
"zip": "97205"
},
"client_details": {
"browser_ip": "192.168.0.1",
"user_agent": "safari webkit"
},
"created_at": "2021-11-09T19:22:13+00:00",
"currency": "USD",
"customer": {
"id": 37657002,
"email": "test@test.com",
"external_customer_id": {
"ecommerce": "2879413682227"
},
"hash": "7e706455cbd13e40"
},
"discounts": [
{
"id": 12345,
"code": "TESTCODE10",
"value": 10,
"value_type": "fixed_amount"
}
],
"error": null,
"error_type": null,
"external_order_id": {
"ecommerce": "2541635698739"
},
"external_transaction_id": {
"payment_processor": "ch_1HzWElJ2zqHvZRd1TWKFFqDR"
},
"has_uncommitted_changes": false,
"line_items": [
{
"purchase_item_id": 63898947,
"external_product_id": {
"ecommerce": "4381728735283"
},
"external_variant_id": {
"ecommerce": "999999999999"
},
"grams": 454,
"handle": "shirt-package",
"images": {
"large": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h_large.jpg",
"medium": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h_medium.jpg",
"original": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h.jpg",
"small": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h_small.jpg"
},
"original_price": "10.00",
"properties": [
{
"name": "Color",
"value": "Blue"
}
],
"purchase_item_type": "subscription",
"quantity": 1,
"sku": "TOM0001",
"tax_due": "1.30",
"tax_lines": [
{
"price": "0.9931",
"rate": "0.0725",
"title": "CA State Tax",
"unit_price": "0.3310"
},
{
"price": "0.3082",
"rate": "0.0225",
"title": "LA County Tax",
"unit_price": "0.1027"
}
],
"taxable": true,
"taxable_amount": "10.00",
"title": "Shirt package",
"total_price": "11.30",
"unit_price": "10.00",
"unit_price_includes_tax": false,
"variant_title": "Blue T-shirt"
}
],
"note": "next order in sequence 3",
"order_attributes": [
{
"name": "Checkout-Method",
"value": "delivery"
}
],
"orders_count": 1,
"payment_processor": "stripe",
"processed_at": "2021-11-09T23:59:31+00:00",
"retry_date": null,
"scheduled_at": "2021-12-09",
"shipping_address": {
"address1": "1030 Barnum Ave",
"address2": "Suite 101",
"city": "Stratford",
"company": "Fake Company",
"country_code": "US",
"first_name": "Fake First",
"last_name": "Fake Last",
"phone": "999-999-9999",
"province": "Connecticut",
"zip": "06614"
},
"shipping_lines": [
{
"code": "Standard",
"price": "4.90",
"source": "shopify",
"taxable": true,
"tax_lines": [
{
"price": "0.355",
"rate": 0.0725,
"title": "CA State Tax"
},
{
"price": "0.110",
"rate": 0.0225,
"title": "Los Angeles County Tax"
}
],
"title": "Standard"
}
],
"status": "success",
"subtotal_price": "10.00",
"tags": "Subscription, Subscription Recurring Order",
"tax_lines": [
{
"price": "0.950",
"rate": 0.0725,
"title": "CA State Tax"
},
{
"price": "0.335",
"rate": 0.0225,
"title": "Los Angeles County Tax"
}
],
"taxable": true,
"total_discounts": "10.0",
"total_line_items_price": "10.00",
"total_price": "11.29",
"total_refunds": "0.00",
"total_tax": "1.29",
"total_weight_grams": 454,
"type": "recurring",
"updated_at": "2021-11-09T23:59:32+00:00"
}
}
Checkouts
Checkouts
allow you to create, update, and process a Checkout
programmatically. Shipping cost and sales tax determination are automatic functions of the Recharge Checkout resource.
Related guides: Recharge checkout integrations, How to use the Checkout resource
Important - The Checkout
endpoints are only available for BigCommerce and Custom. Checkouts on Shopify must go through Shopify.
The checkout object
Checkouts
resource allows you to create, update, and process a checkout programmatically. Shipping cost and sales tax determination are automatic functions of the Recharge Checkout resource.
The Checkout
endpoints are only available for BigCommerce and Custom. Checkouts
on Shopify must go through Shopify.
Attributes
- charge_idinteger
ID for the
Charge
resulting from processing theCheckout
. - analytics_dataobject
Urchin Tracking Module (UTM) parameters are used for online marketing campaigns.
Show object attributes - applied_discountsarray
Discount
details, populated once theDiscount
has been applied successfully to theCheckout
.Show object attributes - applied_shipping_rateobject
Shipping rates details, populated once the rates have been selected and applied successfully to the
Checkout
.Show object attributes - available_shipping_ratesarray
Shipping rates available for the shipping address provided in the
Checkout
.Checkout
object must contain theshipping_address
before theavailable_shipping_rates
are populated.Show object attributes - billing_addressobject
Billing
Address
for theCheckout
.Show object attributes - completed_atdatetime
Timestamp for when the
Checkout
was processed. - created_atdatetime
Timestamp for when the
Checkout
was created. - currencystring
Currency of the
Checkout
. - emailstring
Email address for the
Customer
. - external_checkout_idstring
External checkout reference, if one exists.
- external_checkout_sourcestring
External checkout platform, if one exists.
- external_customer_idobject
External customer reference, if one exists.
Show object attributes - external_transaction_idobject
The ID of the associated transaction in a payment processor system (like Stripe).
Show object attributes - line_itemsarray
A list of items included in the
Checkout
quantity
andexternal_variant_id
are required parameters inline_items
.Show object attributes - notestring
Custom note.
- notification_preferencesobject
Notification preferences for the
Customer
.Show object attributes - order_attributesarray
List of name-value pairs for custom attributes.
Show object attributes - payment_processorstring
Name of the payment processor.
- requires_shippingboolean
Whether or not the
Checkout
contains items that require shipping. - shipping_addressobject
Shipping
Address
for theCheckout
.Show object attributes - shipping_linesobject
Details of shipping rate, cost…
Show object attributes - subtotal_pricestring
Value of the
Checkout
minus shipping and tax. - tax_linesarray
Array of
tax_line
objects.Show object attributes - taxableboolean
Whether the
Checkout
contains items that are taxable. - taxes_includedboolean
Whether the tax is included in the price of the items.
- tokenstring
Unique token for the
Checkout
. - total_pricestring
Full price of the
Checkout
including shipping and tax. - total_taxstring
Tax charged on the
Checkout
. - updated_atdatetime
Timestamp for the latest
Checkout
update.
Error related attributes
More Attributes
{
"checkout": {
"charge_id": null,
"analytics_data": {
"utm_params": [
{
"utm_campaign": "spring_sale",
"utm_content": "textlink",
"utm_data_source": "cookie",
"utm_medium": "cpc",
"utm_source": "google",
"utm_term": "mleko",
"utm_timestamp": "2020-03-05T00:00:00+00:00"
}
]
},
"applied_discounts": [
{
"amount": "5.00",
"applicable": true,
"discount_code": "5_DOLLARS_OFF",
"non_redeemable_reason": null,
"value": "5.00",
"value_type": "fixed_amount"
}
],
"applied_shipping_rate": {
"checkout": {
"subtotal_price": null,
"total_price": null,
"total_tax": null
},
"code": "Flat rate (3 - 7 Business Days)",
"delivery_range": null,
"description": null,
"handle": "recharge-Flat%20rate%20%283%20-%207%20Business%20Days%29-7.49",
"name": "Flat rate (3 - 7 Business Days)",
"phone_required": null,
"price": "7.49",
"tax_lines": [],
"title": "Flat rate (3 - 7 Business Days)"
},
"available_shipping_rates": [
{
"checkout": {
"subtotal_price": null,
"total_price": null,
"total_tax": null
},
"code": "Flat rate (3 - 7 Business Days)",
"delivery_range": null,
"description": null,
"handle": "recharge-Flat%20rate%20%283%20-%207%20Business%20Days%29-7.49",
"name": "Flat rate (3 - 7 Business Days)",
"phone_required": null,
"price": "7.49",
"tax_lines": [],
"title": "Flat rate (3 - 7 Business Days)"
},
{
"checkout": {
"subtotal_price": null,
"total_price": null,
"total_tax": null
},
"code": "3 Days (3 Business Days)",
"delivery_range": null,
"description": null,
"handle": "recharge-3%20Days%20%283%20Business%20Days%29-11.75",
"name": "3 Days (3 Business Days)",
"phone_required": null,
"price": "11.75",
"tax_lines": [],
"title": "3 Days (3 Business Days)"
}
],
"billing_address": {
"address1": "6419 Ocean Front Walk",
"address2": "Apt 2",
"city": "Los Angeles",
"company": null,
"country_code": "US",
"first_name": "Novak",
"last_name": "Djokovic",
"phone": "1-800-800-8000",
"province": "California",
"zip": "90293"
},
"completed_at": null,
"created_at": "2021-11-16T00:51:28+00:00",
"currency": "USD",
"email": "somerandomemail@test.com",
"external_checkout_id": "<external_cart_id>",
"external_checkout_source": "custom",
"external_customer_id": {
"ecommerce": null,
"payment_processor": null
},
"external_transaction_id": {
"payment_processor": null
},
"line_items": [
{
"id": 0,
"external_inventory_policy": "decrement_obeying_policy",
"external_product_id": {
"ecommerce": "12345"
},
"external_variant_id": {
"ecommerce": "123456"
},
"handle": "shirt-with-design",
"images": {
"small": "https://veryniceimage.jpg"
},
"properties": [
{
"name": "size",
"value": "xl"
}
],
"purchase_item_type": "onetime",
"quantity": 3,
"recurring_unit_price": "12.00",
"requires_shipping": true,
"sku": null,
"subscription_preferences": {
"charge_delay": null,
"charge_interval_frequency": null,
"charge_on_day_of_month": null,
"charge_on_day_of_week": null,
"number_charges_until_expiration": null,
"shipping_interval_frequency": null,
"shipping_interval_unit_type": null
},
"tax_lines": [],
"taxable": false,
"title": "A Nice Shirt",
"total_price": "36.00",
"unit_price": "12.00",
"variant_title": null,
"weight": 340,
"weight_unit": "g"
}
],
"note": "flash sale",
"notification_preferences": {
"email": {
"promotional": {
"last_opt_in_at": null,
"last_opt_in_source": null,
"last_opt_out_at": null,
"last_opt_out_source": null,
"status": "unspecified"
},
"replenishment": {
"last_opt_in_at": null,
"last_opt_in_source": null,
"last_opt_out_at": null,
"last_opt_out_source": null,
"status": "unspecified"
},
"transactional": {
"last_opt_in_at": null,
"last_opt_in_source": null,
"last_opt_out_at": null,
"last_opt_out_source": null,
"status": "unspecified"
}
},
"sms": {
"promotional": {
"last_opt_in_at": null,
"last_opt_in_source": null,
"last_opt_out_at": null,
"last_opt_out_source": null,
"status": "unspecified"
},
"replenishment": {
"last_opt_in_at": null,
"last_opt_in_source": null,
"last_opt_out_at": null,
"last_opt_out_source": null,
"status": "unspecified"
},
"transactional": {
"last_opt_in_at": null,
"last_opt_in_source": null,
"last_opt_out_at": null,
"last_opt_out_source": null,
"status": "unspecified"
}
}
},
"order_attributes": {
"customer_niceness": "very"
},
"payment_processor": null,
"requires_shipping": true,
"shipping_address": {
"address1": "6419 Ocean Front Walk",
"address2": "Apt 2",
"city": "Los Angeles",
"company": null,
"country_code": "US",
"first_name": "Novak",
"last_name": "Djokovic",
"phone": "1-800-800-8000",
"province": "California",
"zip": "90293"
},
"shipping_lines": [
{
"code": "Flat rate (3 - 7 Business Days)",
"price": "7.49",
"tax_lines": [],
"taxable": false,
"title": "Flat rate (3 - 7 Business Days)"
}
],
"subtotal_price": "31.00",
"tax_lines": [],
"taxable": false,
"taxes_included": false,
"token": "efd5a6b12b984f439ec16ce2a00fb5c3",
"total_price": "31.00",
"total_tax": "0.00",
"updated_at": "2021-11-16T00:51:28+00:00"
}
}
Create a checkout
Create a Checkout
The Checkout
endpoints are only available for BigCommerce and Custom. Checkouts on Shopify must go through Shopify.
Query Parameters
- get_shipping_ratesboolean
If specified as a query parameter, shipping rates will be retrieved and will allow providing applied_shipping_rate upon checkout creation.
More Parameters
Body Parameters
- analytics_dataobject
Urchin Tracking Module (UTM) parameters are used for online marketing campaigns.
Show object attributes - applied_discountsarray
Discount
to apply to theCheckout
.Show object attributes - applied_shipping_rateobject
Shipping rates details, populated once the rates have been selected and applied successfully to the
Checkout
.Important: You can only create a checkout with
applied_shipping_rate
if you pass the query parameterget_shipping_rates=true
or if you supply a list of shipping rates usingcustom_shipping_rate_options
.Show object attributes - custom_shipping_rate_optionsarray
List of available shipping rates to use when selecting a shipping rate with
applied_shipping_rate
. Custom shipping rates will take precedence over any default rates fetched usingget_shipping_rates=true
.Show object attributes - billing_addressobject
Billing
Address
for theCheckout
.Show object attributes - currencystring
Currency of the
Checkout
. - emailstring
Email address for the
Customer
. - external_checkout_idstring
External checkout reference, if one exists.
- external_checkout_sourcestring
External checkout platform, if one exists.
- external_transaction_idobject
The ID of the associated transaction in a payment processor system (like Stripe).
Show object attributes - line_itemsarray* Required
A list of items included in the
Checkout
quantity
andexternal_variant_id
are required parameters inline_items
.Show object attributes - notestring
Custom note.
- order_attributesarray
List of name-value pairs for custom attributes.
Show object attributes - shipping_addressobject
Shipping
Address
for theCheckout
.Show object attributes
More Parameters
Responses
- 200
successful response
Show response object
curl 'https://api.rechargeapps.com/checkouts' \
-H 'Content-Type: application/json' \
-H 'X-Recharge-Access-Token: your_api_token' \
-H 'X-Recharge-Version: 2021-11' \
-d '{
"analytics_data": {
"utm_params": [
{
"utm_campaign": "spring_sale",
"utm_content": "textlink",
"utm_data_source": "shopify_cookie",
"utm_medium": "cpc",
"utm_source": "google",
"utm_term": "mleko",
"utm_timestamp": "2020-03-05T00:00:00"
}
]
},
"applied_discounts": [
{
"discount_code": "5_DOLLARS_OFF"
}
],
"billing_address": {
"address1": "6419 Ocean Front Walk",
"address2": "Apt 2",
"city": "Los Angeles",
"company": "",
"country_code": "US",
"first_name": "Novak",
"last_name": "Djokovic",
"phone": "1-800-800-8000",
"province": "California",
"zip": "90293"
},
"email": "somerandomemail@test.com",
"external_checkout_id": "<external_cart_id>",
"external_checkout_source": "custom",
"line_items": [
{
"external_product_id": {
"ecommerce": "12345"
},
"external_variant_id": {
"ecommerce": "123456"
},
"handle": "shirt-with-design",
"images": {
"small": "http://small.jpg"
},
"properties": [
{
"name": "size",
"value": "xl"
},
{
"name": "color",
"value": "fuchsia"
}
],
"quantity": 3,
"subscription_preferences": {
"charge_interval_frequency": null,
"cutoff_day_of_month": null,
"cutoff_day_of_week": null,
"expire_after_specific_number_of_charges": null,
"interval_unit": null,
"order_day_of_month": null,
"order_day_of_week": null,
"order_interval_frequency": null
},
"tax_lines": [
{
"price": "0.950",
"rate": 0.0725,
"title": "CA State Tax"
}
]
}
],
"note": "flash sale",
"order_attributes": {
"customer_niceness": "very"
},
"shipping_address": {
"address1": "6419 Ocean Front Walk",
"address2": "Apt 2",
"city": "Los Angeles",
"company": "",
"country_code": "US",
"first_name": "Novak",
"last_name": "Djokovic",
"phone": "1-800-800-8000",
"province": "California",
"zip": "90293"
}
}'
{
"checkout": {
"charge_id": null,
"analytics_data": {
"utm_params": [
{
"utm_campaign": "spring_sale",
"utm_content": "textlink",
"utm_data_source": "shopify_cookie",
"utm_medium": "cpc",
"utm_source": "google",
"utm_term": "mleko",
"utm_timestamp": "2020-03-05T00:00:00+00:00"
}
]
},
"applied_discounts": [
{
"amount": "5.00",
"applicable": true,
"discount_code": "5_DOLLARS_OFF",
"non_redeemable_reason": null,
"value": "5.00",
"value_type": "fixed_amount"
}
],
"billing_address": {
"address1": "6419 Ocean Front Walk",
"address2": "Apt 2",
"city": "Los Angeles",
"company": null,
"country_code": "US",
"first_name": "Novak",
"last_name": "Djokovic",
"phone": "1-800-800-8000",
"province": "California",
"zip": "90293"
},
"completed_at": null,
"created_at": "2021-11-16T00:51:28+00:00",
"currency": "USD",
"email": "somerandomemail@test.com",
"external_checkout_id": "f71848585658686-36f6-d9efg8125rogkfdaa",
"external_checkout_source": "custom",
"external_customer_id": {
"ecommerce": null,
"payment_processor": null
},
"external_transaction_id": {
"payment_processor": null
},
"line_items": [
{
"id": null,
"external_inventory_policy": "decrement_obeying_policy",
"external_product_id": {
"ecommerce": "12345"
},
"external_variant_id": {
"ecommerce": "123456"
},
"handle": "shirt-with-design",
"images": {
"small": "http://small.jpg"
},
"properties": [
{
"name": "size",
"value": "xl"
},
{
"name": "color",
"value": "fuchsia"
}
],
"purchase_item_type": "onetime",
"quantity": 3,
"requires_shipping": true,
"sku": null,
"subscription_preferences": {
"charge_interval_frequency": null,
"cutoff_day_of_month": null,
"cutoff_day_of_week": null,
"expire_after_specific_number_of_charges": null,
"interval_unit": null,
"order_day_of_month": null,
"order_day_of_week": null,
"order_interval_frequency": null
},
"tax_lines": [],
"taxable": false,
"title": "A Very Nice Shirt",
"total_price": "36.00",
"unit_price": "12.00",
"variant_title": null,
"weight": 340,
"weight_unit": "g"
}
],
"note": "flash sale",
"notification_preferences": {
"email": {
"promotional": {
"last_opt_in_at": null,
"last_opt_in_source": null,
"last_opt_out_at": null,
"last_opt_out_source": null,
"status": "unspecified"
},
"replenishment": {
"last_opt_in_at": null,
"last_opt_in_source": null,
"last_opt_out_at": null,
"last_opt_out_source": null,
"status": "unspecified"
},
"transactional": {
"last_opt_in_at": null,
"last_opt_in_source": null,
"last_opt_out_at": null,
"last_opt_out_source": null,
"status": "unspecified"
}
},
"sms": {
"promotional": {
"last_opt_in_at": null,
"last_opt_in_source": null,
"last_opt_out_at": null,
"last_opt_out_source": null,
"status": "unspecified"
},
"replenishment": {
"last_opt_in_at": null,
"last_opt_in_source": null,
"last_opt_out_at": null,
"last_opt_out_source": null,
"status": "unspecified"
},
"transactional": {
"last_opt_in_at": null,
"last_opt_in_source": null,
"last_opt_out_at": null,
"last_opt_out_source": null,
"status": "unspecified"
}
}
},
"order_attributes": {
"customer_niceness": "very"
},
"payment_processor": null,
"requires_shipping": true,
"shipping_address": {
"address1": "6419 Ocean Front Walk",
"address2": "Apt 2",
"city": "Los Angeles",
"company": null,
"country_code": "US",
"first_name": "Novak",
"last_name": "Djokovic",
"phone": "1-800-800-8000",
"province": "California",
"zip": "90293"
},
"subtotal_price": "31.00",
"tax_lines": [],
"taxable": false,
"taxes_included": false,
"token": "efd5a6b12b984f439ec16ce2a00fb5c3",
"total_price": "31.00",
"total_tax": "0.00",
"updated_at": "2021-11-16T00:51:28+00:00"
}
}
Retrieve a checkout
Retrieve a checkout.
The Checkout
endpoints are only available for BigCommerce and Custom. Checkouts on Shopify must go through Shopify.
Responses
- 200
successful response
Show response object
curl 'https://api.rechargeapps.com/checkouts/6a7c36a1213a4d7fb746e6588fa55005' \
-H 'X-Recharge-Access-Token: your_api_token' \
-H 'X-Recharge-Version: 2021-11'
{
"checkout": {
"charge_id": null,
"analytics_data": {
"utm_params": [
{
"utm_campaign": "spring_sale",
"utm_content": "textlink",
"utm_data_source": "shopify_cookie",
"utm_medium": "cpc",
"utm_source": "google",
"utm_term": "mleko",
"utm_timestamp": "2020-03-05T00:00:00+00:00"
}
]
},
"applied_discounts": [
{
"amount": "5.00",
"applicable": true,
"discount_code": "5_DOLLARS_OFF",
"non_redeemable_reason": null,
"value": "5.00",
"value_type": "fixed_amount"
}
],
"applied_shipping_rate": {
"checkout": {
"subtotal_price": null,
"total_price": null,
"total_tax": null
},
"code": "3 Days (3 Business Days)",
"delivery_range": null,
"description": null,
"handle": "recharge-3%20Days%20%283%20Business%20Days%29-11.75",
"name": "3 Days (3 Business Days)",
"phone_required": null,
"price": "11.75",
"tax_lines": [],
"title": "3 Days (3 Business Days)"
},
"available_shipping_rates": [
{
"checkout": {
"subtotal_price": null,
"total_price": null,
"total_tax": null
},
"code": "Flat rate (3 - 7 Business Days)",
"delivery_range": null,
"description": null,
"handle": "recharge-Flat%20rate%20%283%20-%207%20Business%20Days%29-7.49",
"name": "Flat rate (3 - 7 Business Days)",
"phone_required": null,
"price": "7.49",
"tax_lines": [],
"title": "Flat rate (3 - 7 Business Days)"
},
{
"checkout": {
"subtotal_price": null,
"total_price": null,
"total_tax": null
},
"code": "3 Days (3 Business Days)",
"delivery_range": null,
"description": null,
"handle": "recharge-3%20Days%20%283%20Business%20Days%29-11.75",
"name": "3 Days (3 Business Days)",
"phone_required": null,
"price": "11.75",
"tax_lines": [],
"title": "3 Days (3 Business Days)"
}
],
"billing_address": {
"address1": "6419 Ocean Front Walk",
"address2": "Apt 2",
"city": "Los Angeles",
"company": null,
"country_code": "US",
"first_name": "Novak",
"last_name": "Djokovic",
"phone": "1-800-800-8000",
"province": "California",
"zip": "90293"
},
"completed_at": null,
"created_at": "2021-11-16T00:51:28+00:00",
"currency": "USD",
"email": "somerandomemail@test.com",
"external_checkout_id": "<external_cart_id>",
"external_checkout_source": "headless",
"external_customer_id": {
"ecommerce": null,
"payment_processor": null
},
"external_transaction_id": {
"payment_processor": null
},
"line_items": [
{
"id": null,
"external_inventory_policy": "decrement_obeying_policy",
"external_product_id": {
"ecommerce": "12345"
},
"external_variant_id": {
"ecommerce": "123456"
},
"handle": "shirt-with-design",
"images": {
"small": "http://small.jpg"
},
"properties": [
{
"name": "size",
"value": "xl"
}
],
"purchase_item_type": "onetime",
"quantity": 3,
"requires_shipping": true,
"sku": null,
"subscription_preferences": {
"charge_interval_frequency": null,
"cutoff_day_of_month": null,
"cutoff_day_of_week": null,
"expire_after_specific_number_of_charges": null,
"interval_unit": null,
"order_day_of_month": null,
"order_day_of_week": null,
"order_interval_frequency": null
},
"tax_lines": [],
"taxable": false,
"title": "A Very Nice Shirt",
"total_price": "36.00",
"unit_price": "12.00",
"variant_title": null,
"weight": 340,
"weight_unit": "g"
}
],
"note": "flash sale",
"notification_preferences": {
"email": {
"promotional": {
"last_opt_in_at": null,
"last_opt_in_source": null,
"last_opt_out_at": null,
"last_opt_out_source": null,
"status": "unspecified"
},
"replenishment": {
"last_opt_in_at": null,
"last_opt_in_source": null,
"last_opt_out_at": null,
"last_opt_out_source": null,
"status": "unspecified"
},
"transactional": {
"last_opt_in_at": null,
"last_opt_in_source": null,
"last_opt_out_at": null,
"last_opt_out_source": null,
"status": "unspecified"
}
},
"sms": {
"promotional": {
"last_opt_in_at": null,
"last_opt_in_source": null,
"last_opt_out_at": null,
"last_opt_out_source": null,
"status": "unspecified"
},
"replenishment": {
"last_opt_in_at": null,
"last_opt_in_source": null,
"last_opt_out_at": null,
"last_opt_out_source": null,
"status": "unspecified"
},
"transactional": {
"last_opt_in_at": null,
"last_opt_in_source": null,
"last_opt_out_at": null,
"last_opt_out_source": null,
"status": "unspecified"
}
}
},
"order_attributes": {
"customer_niceness": "very"
},
"payment_processor": null,
"requires_shipping": true,
"shipping_address": {
"address1": "6419 Ocean Front Walk",
"address2": "Apt 2",
"city": "Los Angeles",
"company": null,
"country_code": "US",
"first_name": "Novak",
"last_name": "Djokovic",
"phone": "1-800-800-8000",
"province": "California",
"zip": "90293"
},
"subtotal_price": "31.00",
"tax_lines": [],
"taxable": false,
"taxes_included": false,
"token": "efd5a6b12b984f439ec16ce2a00fb5c3",
"total_price": "31.00",
"total_tax": "0.00",
"updated_at": "2021-11-16T00:51:28+00:00"
}
}
Update a checkout
You can modify an existing checkout to match the specified parameters.
The Checkout
endpoints are only available for BigCommerce and Custom. Checkouts on Shopify must go through Shopify.
Query Parameters
- get_shipping_ratesboolean
If specified as a query parameter, shipping rates will be retrieved and will allow providing applied_shipping_rate upon checkout update.
More Parameters
Body Parameters
- analytics_dataarray
Urchin Tracking Module (UTM) parameters are used for online marketing campaigns.
Show object attributes - applied_shipping_rateobject
Shipping rates details, populated once the rates have been selected and applied successfully to the
Checkout
.Important: You can only set
applied_shipping_rate
if you have already collected theavailable_shipping_rates
by callingGET /checkout/<checkout_token>/shipping_rates
or if you pass the query parameterget_shipping_rates=true
when calling this route.code
,price
andtitle
are required when setting a custom shipping rateShow object attributes - billing_addressobject
Billing address for the checkout.
Show object attributes - buyer_accepts_marketingboolean
Does the buyer accept marketing, newsletters etc.
- discount_codestring
Discount code to be used on the checkout, e.g. “DISCOUNT20”.
- currencystring
Currency of the
Checkout
. - emailstring
Email address for the customer.
- external_checkout_idstring
Represents the external cart token.
- external_checkout_sourcestring
Represents the source for
external_checkout_id
. - line_itemsarray
quantity
,product_id
andvariant_id
are required parameters in line_items.Show object attributes - notestring
Note attribute used to store custom notes.
- partial_shippingboolean
When set to true, shipping address validations are reduced to only require country and zip when creating/updating a checkout. The full shipping address including address line 1 must be added to the checkout before processing associated charges. This is helpful for mobile payments.
Related guides: Mobile payments - order_attributesarray
Structured custom notes.
Show object attributes - phonestring
Customer phone number.
- shipping_addressobject
Shipping address for the checkout.
When using mobile payment options, insufficient shipping address data is available until payment intent, which causes validation errors when updating the checkout object.
Related guides: Checkout mobile paymentShow object attributes - shipping_linearray
Shipping lines.
Show object attributes
More Parameters
Responses
- 200
successful response
Show response object
curl -X PUT 'https://api.rechargeapps.com/checkouts/b706eecfd66c45329d3886a02d7515d6' \
-H 'Content-Type: application/json' \
-H 'X-Recharge-Access-Token: your_api_token' \
-H 'X-Recharge-Version: 2021-11' \
-d '{
"line_items": [
{
"product_id": 4546063663207,
"quantity": 6,
"variant_id": 3844924611
}
]
}'
{
"checkout": {
"analytics_data": {
"utm_params": [
{
"utm_campaign": "spring_sale",
"utm_content": "textlink",
"utm_data_source": "shopify_cookie",
"utm_medium": "cpc",
"utm_source": "google",
"utm_term": "mleko",
"utm_timestamp": "2020-03-05T00:00:00+00:00"
}
]
},
"applied_discount": {
"amount": "7.50",
"applicable": true,
"non_applicable_reason": "",
"value": "7.50",
"value_type": "fixed_amount"
},
"billing_address": null,
"buyer_accepts_marketing": false,
"charge_id": null,
"completed_at": null,
"created_at": "2020-07-10T09:21:46.259352+00:00",
"currency": "USD",
"discount_code": "POPUS_25",
"email": "somerandomemail@test.com",
"external_checkout_id": "<shopify_cart_token>",
"external_checkout_source": "shopify",
"line_items": [
{
"charge_interval_frequency": 5,
"cutoff_day_of_month": null,
"cutoff_day_of_week": null,
"expire_after_specific_number_of_charges": null,
"first_recurring_charge_delay": null,
"fulfillment_service": "manual",
"image": "//cdn.shopify.com/s/files/1/0279/8387/2103/products/kazan_small.jpg?v=1586451337",
"line_price": "30.00",
"order_day_of_month": null,
"order_day_of_week": null,
"order_interval_frequency": 5,
"order_interval_unit": "day",
"order_interval_unit_type": "day",
"original_price": "5.00",
"price": "5.00",
"product_id": 4546063663207,
"product_type": "Milk",
"properties": null,
"quantity": 6,
"recurring_price": "5.00",
"requires_shipping": true,
"sku": "kRaViCah-1",
"tax_code": null,
"taxable": false,
"title": "Powder Milk",
"type": "SUBSCRIPTION",
"variant_id": 32165284380775,
"variant_title": "1 / Powder",
"vendor": "Imlek"
}
],
"note": null,
"order_attributes": null,
"payment_processor": null,
"payment_processor_customer_id": null,
"payment_processor_transaction_id": null,
"phone": null,
"requires_shipping": true,
"shipping_address": {
"address1": "6419 Ocean Front Walk",
"address2": "Apt 2",
"city": "Los Angeles",
"company": "",
"country_code": "US",
"first_name": "Novak",
"last_name": "Djokovic",
"phone": "1-800-800-8000",
"province": "California",
"zip": "90293"
},
"shipping_address_validations": {
"country_is_supported": true,
"ups": true
},
"shipping_line": null,
"shipping_rate": null,
"subtotal_price": "22.50",
"tax_lines": [
{
"price": "0.950",
"rate": 0.0725,
"title": "CA State Tax"
},
{
"price": "0.335",
"rate": 0.0225,
"title": "Los Angeles County Tax"
}
],
"taxes_included": false,
"token": "b706eecfd66c45329d3886a02d7515d6",
"total_price": "22.50",
"total_tax": "0.00",
"updated_at": "2020-07-10T09:21:46.284703+00:00"
}
}
Retrieve shipping rates
You can retrieve all shipping rates for a specific checkout.
Responses
- 200
successful response
Show response object
curl 'https://api.rechargeapps.com/checkouts/6a7c36a1213a4d7fb746e6588fa55005/shipping_rates' \
-H 'X-Recharge-Access-Token: your_api_token' \
-H 'X-Recharge-Version: 2021-11'
{
"shipping_rates": [
{
"checkout": {
"subtotal_price": "22.50",
"total_price": "42.40",
"total_tax": "0.00"
},
"code": "Standard",
"delivery_range": null,
"description": null,
"handle": "shopify-Standard-19.90",
"name": "Standard",
"phone_required": false,
"price": "19.90",
"title": "Standard"
}
]
}
Process a checkout
You can process and charge checkout using our API.
The Checkout
endpoints are only available for BigCommerce and Custom. Checkouts on Shopify must go through Shopify.
We support Stripe, Apple Pay, Google Pay, and Braintree as payment processor.
If you are using test stripe keys, you can use tok_visa
as your token.
Related guides: Mobile payments
Body Parameters
- payment_processorstring* Required
Possible values: stripe, braintree, authorize
The name of payment processor.
- payment_tokenstring* Required
Payment token that will be used in transaction.
For Stripe this field needs to be populated with a payment method.
For Braintree this field needs to be populated with a payment nonce. - payment_typestring
Possible values: CREDIT_CARD, PAYPAL, APPLE_PAY, GOOGLE_PAY, SEPA_DEBIT
The payment type used for the
Checkout
.
More Parameters
Responses
- 200
successful response
Show response object
curl 'https://api.rechargeapps.com/checkouts/5a5c19ea31c44641855017f1276db959/process' \
-H 'Content-Type: application/json' \
-H 'X-Recharge-Access-Token: your_api_token' \
-H 'X-Recharge-Version: 2021-11' \
-d '{
"payment_processor": "stripe",
"payment_token": "<payment_token>",
"payment_type": "CREDIT_CARD"
}'
{
"checkout_charge": {
"authorization_token": null,
"charge_id": 258065996,
"free": false,
"payment_processor": "stripe",
"processor_customer_token": "cus_HcbgqiS49fABBg62E",
"processor_payment_method_token": "pm_lkj3lkl4lk34",
"payment_processor_transaction_id": "ch_1H3McXJ2zdqHvZaRd191xV2idRt",
"payment_token": "tok_visa",
"payment_type": "CREDIT_CARD",
"status": "successful"
}
}
Collections
Collections
allow to create and manage a logical list of Products
called a Collection
. A Collection
contains an ordered list of Products
and can be used for selective display of Products
on chosen interfaces or for business logic automation (e.g. Discounts
definition…).
The collection object
Attributes
- idinteger
Unique numeric identifier for the
Collection
. - created_atdatetime
The date and time when the
Collection
was created. - descriptionstring
The
Collection
description. Used to store a detailed explanation of theCollection
content and its purpose. - sort_orderstring
Possible values: id-asc, id-desc, title-asc, title-desc, created_at-asc, created_at-desc
The order in which the
Products
in the collection should appear. - titlestring
The title of the
Collection
. A short descriptive definition of theCollection
. - typestring
Possible values: manual
The type of the
Collection
.manual
stands forCollections
which were manually created ie. when the products where explicitly selected to be part of theCollection
. - updated_atdatetime
The date and time when the
Collection
was last updated.
Error related attributes
More Attributes
{
"id": 4021,
"created_at": "2021-08-30T17:25:57+00:00",
"description": "August 2021 cat products new release.",
"sort_order": "id-desc",
"title": "Cats Summer Collection",
"type": "manual",
"updated_at": "2021-08-30T17:25:57+00:00"
}
Create a collection
Create a Collection
in Recharge.
Body Parameters
- descriptionstring* Required
The
Collection
description. Used to store a detailed explanation of theCollection
content and its purpose. - sort_orderstring
Default: id-desc
Possible values: id-asc, id-desc, title-asc, title-desc, created-asc, created-desc
The order in which the
Products
in the collection should appear. - titlestring* Required
The title of the
Collection
. A short descriptive definition of theCollection
.
More Parameters
Responses
- 200
successful response
Show response object
curl 'https://api.rechargeapps.com/collections' \
-H 'X-Recharge-Version: 2021-11' \
-H 'Content-Type: application/json' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d '{
"description": "cat products new release",
"title": "Cats Spring 2022"
}
{
"collection": {
"id": 134157,
"created_at": "2022-03-29T04:57:36+00:00",
"description": "cat products new release",
"sort_order": "id-desc",
"title": "Cats Spring 2022",
"type": "manual",
"updated_at": "2020-12-17T18:50:39+00:00"
}
}
Retrieve a collection
Retrieve one Collection
using collection_id
.
Responses
- 200
successful response
Show response object - 404
Not found
Show response object
curl 'https://api.rechargeapps.com/collections/134157' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token'
{
"collection": {
"id": 134157,
"created_at": "2022-03-29T04:57:36+00:00",
"description": "cat products new release",
"sort_order": "id-desc",
"title": "Cats Spring 2022",
"type": "manual",
"updated_at": "2022-03-29T04:57:36+00:00"
}
}
Update a collection
Modify an existing Collection
to match the specified parameters.
Body Parameters
- descriptionstring
The
Collection
description. Used to store a detailed explanation of theCollection
content and its purpose. - sort_orderstring
Possible values: id-asc, id-desc, title-asc, title-desc, created-asc, created-desc
The order in which the
Products
in the collection should appear. - titlestring
The title of the
Collection
. A short descriptive definition of theCollection
.
More Parameters
Responses
- 200
successful response
Show response object - 404
Not found
Show response object - 415
Unprocessable entity
Show response object
curl -X PUT 'https://api.rechargeapps.com/collections/134157' \
-H 'X-Recharge-Version: 2021-11' \
-H 'Content-Type: application/json' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d '{"sort_order": "title-asc"}'
{
"collection": {
"id": 134157,
"created_at": "2022-03-29T04:57:36+00:00",
"description": "cats products new release",
"sort_order": "title-asc",
"title": "Cats Spring 2022",
"type": "manual",
"updated_at": "2022-03-29T05:27:39+00:00"
}
}
Delete a collection
Delete a Collection
For safety and good UX, you can only delete a Collection
if it is not in use in the Customer Portal
Responses
- 204
Content Deleted: The server has successfully fulfilled the request and deleted the desired object and there is no content to send in the response body.
Show response object - 404
Not found
Show response object
curl -X DELETE 'https://api.rechargeapps.com/collections/134129' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token'
{}
List collections
Return a list of Collections
in your store.
HTTP request examples
GET /collections
GET /collections?title=Cats
Query Parameters
- titlestring
The title of the
Collection
. A short descriptive definition of theCollection
.
More Parameters
Responses
- 200
successful response
Show response object
curl 'https://api.rechargeapps.com/collections' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d limit=3 -G
{
"next_cursor": null,
"previous_cursor": null,
"collections": [
{
"id": 134136,
"created_at": "2022-03-28T15:38:27+00:00",
"description": "cat products august 2022",
"sort_order": "title-asc",
"title": "Cats",
"type": "manual",
"updated_at": "2022-03-28T15:38:27+00:00"
},
{
"id": 134129,
"created_at": "2022-03-28T12:27:03+00:00",
"description": "kitten accessories soft.",
"sort_order": "id-asc",
"title": "Soft Kitty",
"type": "manual",
"updated_at": "2022-02-23T11:27:34+00:00"
}
]
}
List collections products
Return a list of the product ids included in Collections
in your store.
HTTP request examples
GET /collection_products
GET /collection_products?collection_id=134157
Query Parameters
- collection_idinteger
The id of the
Collection
for which you want to see the products.
More Parameters
Responses
- 200
successful response
Show response object - 422
Errors. Unprocessable entity.
Show response object
curl 'https://api.rechargeapps.com/collection_products' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d limit=3 -G
{
"next_cursor": null,
"previous_cursor": null,
"collection_products": [
{
"collection_id": 111733,
"created_at": "2022-03-30T18:12:09+00:00",
"external_product_id": "5",
"updated_at": "2022-03-30T18:12:09+00:00"
},
{
"collection_id": 111733,
"created_at": "2022-02-12T12:53:33+00:00",
"external_product_id": "2",
"updated_at": "2022-02-12T12:53:33+00:00"
},
{
"collection_id": 111735,
"created_at": "2022-02-12T06:46:49+00:00",
"external_product_id": "3",
"updated_at": "2022-02-12T06:46:49+00:00"
}
]
}
Add collection products
Add products to a Collection
in Recharge.
Body Parameters
- collection_productsarray* Required
An array of products and their collection definition to be added to the
Collection
.Important: there is a set limit of 250 products that can be added per request.
Show object attributes
More Parameters
Responses
- 201
successful response
Show response object - 422
unprocessable entity
Show response object - 500
internal server error. (often the external_product_id entered does not exist)
Show response object
curl -X POST 'https://api.rechargeapps.com/collections/134129/collection_products-bulk' \
-H 'X-Recharge-Version: 2021-11' \
-H 'Content-Type: application/json' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d '{
"collection_products": [
{
"external_product_id": "7"
}
]
}'
{
"collection_products": [
{
"collection_id": 111733,
"created_at": "2022-03-29T04:57:36+00:00",
"external_product_id": "7",
"updated_at": "2022-03-30T18:32:35+00:00"
}
]
}
Delete collection products
Removes products from a Collection
in Recharge.
Body Parameters
- collection_productsarray* Required
The array of products to be removed from the
Collection
identified by theirexternal_product_id
.Important: there is a set limit of 250 products that can be added per request.
Show object attributes
More Parameters
Responses
- 204
Content Deleted: The server has successfully fulfilled the request and deleted the desired object and there is no content to send in the response body.
Show response object - 404
Not found
Show response object
curl '-X DELETE https://api.rechargeapps.com/collections/134129/collection_products-bulk' \
-H 'X-Recharge-Version: 2021-11' \
-H 'Content-Type: application/json' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d '{
"collection_products": [
{
"external_product_id": "7"
}
]
}'
{}
Credits
Retain
The Credit Account object represents a set of positive and negative credit adjustments that when summed equal an available balance. Credit accounts may not have negative balances. A credit account may be associated with a customer, via the customer_id attribute. A customer may be associated with many credit accounts.
A credit adjustment represents a financial adjustment to the balance of a Credit Account. An adjustment of type ‘credit’ results in an increase in the available balance of a credit account, where an adjustment of type ‘debit’ results in a decrease.
Access to the Credits endpoints are only available for merchants using Retain.
The credit account object
Attributes
- idinteger
Unique numeric identifier for the Credit Account
- customer_idinteger
Unique numeric identifier for the
customer
associated with thecredit_account
. - available_balancestring
The dollar amount of available credit in the credit account, in the associated Store’s default currency as configured in Recharge
- created_atdatetime
The date and time at which the credit account was created.
- currency_codestring
The currency code for the credit account.
- expires_atdatetime
The date and time at which the credit account expires, and can no longer be adjusted.
- initial_valuestring
The initial value of the credit account at the time of creation.
- namestring
The name of the credit account
- typestring
The type of the credit account. Accepted values are
rewards
andmanual
. - updated_atdatetime
The date and time at which the credit account was last updated.
Error related attributes
More Attributes
{
"credit_account": {
"id": 697470,
"customer_id": 36885098,
"available_balance": "10.00",
"created_at": "2024-07-08T20:40:30+00:00",
"currency_code": "USD",
"expires_at": null,
"initial_value": "1.55",
"name": "Reward Credit",
"type": "reward",
"updated_at": "2024-07-08T20:40:30+00:00"
}
}
List credit accounts
Return a list of credit accounts.
Query Parameters
- customer_idstring
Return the list of credit accounts linked to the given Recharge
customer_id
- limitstring
The amount of results. Default is 50 while the maximum is 250.
More Parameters
Responses
- 200
successful response
Show response object
curl 'https://api.rechargeapps.com/credit_accounts' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token'
{
"next_cursor": "eyJzdGFydGluZ19iZWZvcmVfaWQiOjQ5ODg2NywibGFzdF92YWx1ZSI6NDk4ODY3LCJzb3J0X2J5IjoiaWQtZGVzYyIsImN1cnNvcl9kaXIiOiJuZXh0In0",
"previous_cursor": null,
"credit_accounts": [
{
"id": 697470,
"customer_id": 36885098,
"available_balance": "0.00",
"created_at": "2024-07-08T20:40:30+00:00",
"currency_code": "USD",
"expires_at": null,
"initial_value": "0.45",
"name": "Reward Credit",
"type": "reward",
"updated_at": "2024-07-08T20:40:30+00:00"
}
]
}
Retrieve a credit account
Retrieve one credit account using credit_account_id
.
Responses
- 200
successful response
Show response object
curl 'https://api.rechargeapps.com/credit_accounts/697470' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token'
{
"credit_account": {
"id": 697470,
"customer_id": 36885098,
"available_balance": "0.00",
"created_at": "2024-07-08T20:40:30+00:00",
"currency_code": "USD",
"expires_at": null,
"initial_value": "0.45",
"name": "Reward Credit",
"type": "reward",
"updated_at": "2024-07-08T20:40:30+00:00"
}
}
List credit adjustments
Retrieve a list of credit adjustments.
Query Parameters
- limitstring
The amount of results. Default is 50 while the maximum is 250.
More Parameters
Responses
- 200
successful response
Show response object
curl 'https://api.rechargeapps.com/credit_adjustments' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token'
{
"next_cursor": "eyJzdGFydGluZ19iZWZvcmVfaWQiOjQ4NjY4NDksImxhc3RfdmFsdWUiOjQ4NjY4NDksInNvcnRfYnkiOiJpZC1kZXNjIiwiY3Vyc29yX2RpciI6Im5leHQifQ",
"previous_cursor": null,
"credit_adjustments": [
{
"id": 4866849,
"credit_account_id": 697470,
"amount": "0.45",
"created_at": "2024-08-08T04:06:37+00:00",
"currency_code": "USD",
"ending_balance": "0.00",
"note": "Amount related with shop order shop.myshopify.com. Address Id: 169902120 Charge Id: 1125729345",
"type": "debit",
"updated_at": "2024-08-08T04:06:37+00:00"
}
]
}
Retrieve a credit adjustment
Retrieve one credit adjustment using credit_adjustment_id
.
Responses
- 200
successful response
Show response object
curl 'api.rechargeapps.com/credit_adjustments/4866849' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token'
{
"next_cursor": "eyJzdGFydGluZ19iZWZvcmVfaWQiOjQ4NjY4NDksImxhc3RfdmFsdWUiOjQ4NjY4NDksInNvcnRfYnkiOiJpZC1kZXNjIiwiY3Vyc29yX2RpciI6Im5leHQifQ",
"previous_cursor": null,
"credit_adjustments": [
{
"id": 4866849,
"credit_account_id": 697470,
"amount": "0.45",
"created_at": "2024-08-08T04:06:37+00:00",
"currency_code": "USD",
"ending_balance": "0.00",
"note": "Amount related with shop order jordans-curious-tomatoes.myshopify.com Address Id: 169902120 Charge Id: 1125729345",
"type": "debit",
"updated_at": "2024-08-08T04:06:37+00:00"
}
]
}
List Credit Adjustments for a Credit Account
Retrieve credit adjustments associated with specific credit account using credit_account_id
.
Query Parameters
- limitstring
The amount of results. Default is 50 while the maximum is 250.
More Parameters
Responses
- 200
successful response
Show response object
curl 'https://api.rechargeapps.com/credit_accounts/697470/credit_adjustments' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token'
{
"next_cursor": null,
"previous_cursor": null,
"credit_adjustments": [
{
"id": 4866849,
"credit_account_id": 697470,
"amount": "0.45",
"created_at": "2024-08-08T04:06:37+00:00",
"currency_code": "USD",
"ending_balance": "0.00",
"note": "Amount related with shop order store.myshopify.com Address Id: 169902120 Charge Id: 1125729345",
"type": "debit",
"updated_at": "2024-08-08T04:06:37+00:00"
},
{
"id": 4719708,
"credit_account_id": 697470,
"amount": "0.45",
"created_at": "2024-07-08T20:40:31+00:00",
"currency_code": "USD",
"ending_balance": "0.45",
"note": "3% Cash back on curious tomatoes",
"type": "credit",
"updated_at": "2024-07-08T20:40:31+00:00"
}
]
}
Customers
The Customer
object holds account information. Email is unique on the Customer
; no two customers for a store can have the same email. Address
is a child of the Customer
object. There can be many child Addresses
on a customer, but only one parent Customer
per Address
.
The customer object
Attributes
- idinteger
Unique numeric identifier for the
Customer
. - analytics_dataobject
An object containing analytics data associated with the customer.
Show object attributes - apply_credit_to_next_recurring_chargeboolean
A boolean that indicates whether Recharge credits will be applied to the next recurring charge.
- created_atdatetime
The date and time when the customer was created.
- emailstring
The email address of the customer.
- external_customer_idobject
An object containing external ids for the customer record.
Show object attributes - first_charge_processed_atdatetime
Date when first charge was processed for the customer.
- first_namestring
The customer’s first name.
- has_payment_method_in_dunningboolean
A boolean that indicates if the customer has a payment method that is in dunning (failed charge).
- has_valid_payment_methodboolean
Is the payment method valid or not.
- hashstring
The unique string identifier used in a customers portal link.
- last_namestring
The customer’s last name.
- phonestring
The customer’s phone number.
- subscriptions_active_countinteger
The number of active subscriptions on addresses associated with the customer.
- subscriptions_total_countinteger
The total number of subscriptions created on addresses associated with the customer.
- subscription_related_charge_streakinteger
The number of consecutive subscription-related charges processed for this customer, while they retain at least one active subscription. When all subscriptions for this customer are cancelled or expired, this value will reset to zero.
- tax_exemptboolean
Whether the customer tax exempt or not.
- updated_atdatetime
The date and time when the customer was last updated.
Error related attributes
More Attributes
{
"id": 18819267,
"analytics_data": {
"utm_params": [
{
"utm_source": "facebook",
"utm_medium": "cpc"
}
]
},
"created_at": "2018-11-14T08:40:38+00:00",
"email": "example_mail@gmail.com",
"external_customer_id": {
"ecommerce": "126576412310131454"
},
"first_charge_processed_at": null,
"first_name": "Jacob",
"has_payment_method_in_dunning": false,
"has_valid_payment_method": false,
"hash": "18819267b1f9095be98f13a8",
"last_name": "Bronowski",
"phone": "+16175551212",
"subscriptions_active_count": 0,
"subscriptions_total_count": 1,
"tax_exempt": false,
"updated_at": "2020-11-14T08:40:38+00:00"
}
Create a customer in Recharge.
If you plan to add payment information, it must be the tokenized customer representation. We do not accept card data directly. For Stripe users, please make sure it starts with ‘cus’ and not with a 'tok’, as the 'cus’ is prefix for customer token, and 'tok’ is prefix for payment token.
Creating a customer in Recharge will not create the customer on any other platform at this time.
write_payment_methods
permission is only required when creating customers with payment token information, or updating payment token information on a customer.phone
must be in E.164 format, such as +16175551212
.
Body Parameters
- emailstring* Required
The customer’s email address.
- external_customer_idobject
An object containing external ids for the customer record.
Show object attributes - first_namestring* Required
The customer’s first name.
- last_namestring* Required
The customer’s last name.
- phonestring
The customer’s phone number.
- tax_exemptboolean
Whether or not the customer is tax exempt.
More Parameters
Responses
- 200
successful response
Show response object
curl 'https://api.rechargeapps.com/customers' \
-H 'X-Recharge-Version: 2021-11' \
-H 'Content-Type: application/json' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d '{
"first_name": "Niels",
"last_name": "Bohr",
"email": "fake@example.com"
}
{
"customer": {
"id": 37657002,
"analytics_data": {
"utm_params": [
{
"utm_source": "facebook",
"utm_medium": "cpc"
}
]
},
"created_at": "2020-02-19T17:40:07+00:00",
"email": "fake@example.com",
"external_customer_id": {
"ecommerce": "2879413682227"
},
"first_charge_processed_at": "2020-02-19T17:40:11+00:00",
"first_name": "Jane",
"has_payment_method_in_dunning": false,
"has_valid_payment_method": true,
"hash": "7e706455cbd13e40",
"last_name": "Doe",
"phone": null,
"subscriptions_active_count": 0,
"subscriptions_total_count": 0,
"subscription_related_charge_streak": 0,
"tax_exempt": false,
"updated_at": "2020-12-17T18:50:39+00:00"
}
}
Retrieve a customer
Retrieve one customer using customer_id
.
Responses
- 200
successful response
Show response object
curl 'https://api.rechargeapps.com/customers/37657002' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token'
{
"customer": {
"id": 37657002,
"analytics_data": {
"utm_params": [
{
"utm_source": "facebook",
"utm_medium": "cpc"
}
]
},
"created_at": "2020-02-19T17:40:07+00:00",
"email": "fake@example.com",
"external_customer_id": {
"ecommerce": "2879413682227"
},
"first_charge_processed_at": "2020-02-19T17:40:11+00:00",
"first_name": "Jane",
"has_payment_method_in_dunning": false,
"has_valid_payment_method": true,
"hash": "7e706455cbd13e40",
"last_name": "Doe",
"phone": "+16175551212",
"subscriptions_active_count": 0,
"subscriptions_total_count": 1,
"subscription_related_charge_streak": 0,
"tax_exempt": false,
"updated_at": "2020-12-17T18:50:39+00:00"
}
}
Update a customer
Modify an existing Customer
to match the specified parameters.
For US citizens, parameter billing_zip
is required when updating customer’s billing_country
parameter.phone
must be in E.164 format, such as +16175551212
.
Body Parameters
- apply_credit_to_next_recurring_chargeboolean
A boolean that indicates whether Recharge credits will be applied to the next recurring charge.
This property is dependent upon the store’s credit settings. If a call is made attempting to update this and the store has recurring redemption turned off or set to automatically redeem, the API will return a 422 response.
- emailstring
Email address of the customer.
- external_customer_idobject
An object containing external ids for the customer record.
Show object attributes - first_namestring
Customer’s first name.
- last_namestring
Customer’s last name.
- phonestring
The customer’s phone number.
- tax_exemptboolean
Whether or not the customer is tax exempt.
More Parameters
Responses
- 200
successful response
Show response object
curl -X PUT 'https://api.rechargeapps.com/customers/37657002' \
-H 'X-Recharge-Version: 2021-11' \
-H 'Content-Type: application/json' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d '{"last_name": "Doe"}'
{
"customer": {
"id": 37657002,
"analytics_data": {
"utm_params": [
{
"utm_source": "facebook",
"utm_medium": "cpc"
}
]
},
"created_at": "2020-02-19T17:40:07+00:00",
"email": "fake@example.com",
"external_customer_id": {
"ecommerce": "2879413682227"
},
"first_charge_processed_at": "2020-02-19T17:40:11+00:00",
"first_name": "Jane",
"has_payment_method_in_dunning": false,
"has_valid_payment_method": true,
"hash": "7e706455cbd13e40",
"last_name": "Doe",
"phone": "+16175551212",
"subscriptions_active_count": 0,
"subscriptions_total_count": 1,
"tax_exempt": false,
"updated_at": "2020-12-17T18:50:39+00:00"
}
}
Delete a customer
Customer deletion will automatically cancel and delete all child Address, Subscription, Onetime, etc. resources of that customer to eliminate orphaned child data.
To delete a certain address without deleting the customer you can use the Delete Address endpoint.
Responses
- 204
Content Deleted: The server has successfully fulfilled the request and deleted the desired object and there is no content to send in the response body.
Show response object
curl -X DELETE 'https://api.rechargeapps.com/customers/37657002' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token'
{}
List customers
Return a list of customers in your store.
HTTP request examples
GET /customers
GET /customers?email=example@email.com
GET /customers?hash=143806234a9ff87a8d9e
GET /customers?limit=250
GET /customers?page=2
GET /customers?external_customer_id=98273498
If emails contain special characters such as ‘john+doe@example.com’, you will need to encode them in the URLs, i.e. ?email=john%2Bdoe@example.com
Query Parameters
- emailstring
Returns the user linked to the email address provided.
- created_at_maxstring
Gets all customers created before this date.
- created_at_minstring
Gets all customers created after this date.
- hashstring
Returns the user linked to the given recharge customer hash.
- idsstring
Filter customers by id. If passing multiple values, must be comma separated. Non-integer values will result in a 422 error
- limitstring
Default: 50
Max: 250
Number of results.
- pagestring*Deprecated
Default: 1
Page to show.
Page-based pagination has been deprecated but still available to use for pages up to a 100. If you need data past this point, use cursor pagination.
- external_customer_idstring
Returns the user linked to the given
external_customer_id
. - updated_at_maxstring
Gets all customers updated before this date.
- updated_at_minstring
Gets all customers updated after this date.
More Parameters
Responses
- 200
successful response
Show response object
curl 'https://api.rechargeapps.com/customers' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d limit=3 -G
{
"next_cursor": "next_cursor",
"previous_cursor": "previous_cursor",
"customers": [
{
"id": 37657002,
"analytics_data": {
"utm_params": [
{
"utm_source": "facebook",
"utm_medium": "cpc"
}
]
},
"created_at": "2020-02-19T17:40:07+00:00",
"email": "fake@example.com",
"external_customer_id": {
"ecommerce": "2879413682227"
},
"first_charge_processed_at": "2020-02-19T17:40:11+00:00",
"first_name": "Jane",
"has_payment_method_in_dunning": false,
"has_valid_payment_method": true,
"hash": "7e706455cbd13e40",
"last_name": "Doe",
"phone": "+16175551212",
"subscriptions_active_count": 0,
"subscriptions_total_count": 1,
"tax_exempt": false,
"updated_at": "2020-12-17T18:50:39+00:00"
}
]
}
Retrieve a customer delivery schedule
Returns the list of projected deliveries in a specific interval.
If several filters are passed in parameters, the most restrictive will dominate.
The maximum length of the future delivery interval is 365 days.
Query Parameters
- delivery_count_futureinteger
The count of delivery objects that should be included that reference projected orders.
Will not return deliveries past 365 days in the future.
- future_intervalinteger
The count of days forward to report deliveries on.
Defaults to store setting or 90. Maximum of 365 days.
- date_maxstring
The end date of the requested delivery schedule.
Cannot be in the past. Cannot exceed 365 days in the future.
More Parameters
Responses
- 200
successful response
Show response object
curl -X GET 'https://api.rechargeapps.com/customers/37657002/delivery_schedule' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token'
{
"deliverySchedule": {
"customer": {
"id": 73720164,
"email": "test@example.com",
"first_name": "Aria",
"last_name": "Beciu"
},
"deliveries": [
{
"date": "2022-02-07",
"orders": [
{
"id": null,
"address_id": 79342717,
"charge_id": 507503288,
"line_items": [
{
"subscription_id": 186930285,
"external_product_id": {
"ecommerce": "1642443555555"
},
"external_variant_id": {
"ecommerce": null
},
"images": {},
"is_skippable": true,
"is_skipped": false,
"is_prepaid": false,
"original_price": "17.00",
"plan_type": "subscription",
"product_title": "Organic coffee beans",
"properties": [
{
"name": "shipping_interval_unit_type",
"value": "month"
},
{
"name": "shipping_interval_frequency",
"value": "1"
},
{
"name": "subscription_id",
"value": null
}
],
"quantity": 1,
"subtotal_price": "17.00",
"unit_price": "17.00",
"variant_title": "Refill large"
}
],
"order_subtotal": "17.00",
"payment_method": {
"id": 769874,
"billing_address": {
"address1": "90 avenue du Rouergue",
"address2": null,
"city": "Rodez",
"company": null,
"country_code": "FR",
"first_name": "Diane",
"last_name": "Farley",
"phone": null,
"province": null,
"zip": "12000"
},
"payment_details": {}
},
"shipping_address": {
"address1": "149 Forest Avenue",
"address2": null,
"city": "New York City",
"company": null,
"country_code": "US",
"first_name": "Aria",
"last_name": "Beciu",
"phone": "1234567890",
"province": "New York",
"zip": "10019"
}
}
]
}
]
}
}
Retrieve a customer's credit summary
Beta
Returns a credit summary for the customer.
Responses
- 200
successful response
Show response object
curl -X GET 'https://api.rechargeapps.com/customers/37657002/credit_summary' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token'
{
"credit_summary": {
"customer_id": 37657002,
"total_available_balance": "0.00",
"currency_code": "USD"
}
}
Discounts
Discounts can be applied to a Checkout
, or can be applied directly to an Address
. Depending on configuration they allow for single use, or recurring discounts. More details on how to add or remove discounts from an Address
can be found at Update an address.
Often discounts can be used in combination with webhooks, such that when a specific event occurs, it can apply a discount dependent on custom business logic.
There are various options that can be utilized for discounts such as minimum price, single use, recurring for a set number of charges, or ongoing. You can also set the date from which time the discount will become applicable and when it can no longer be applied to a new subscription.
The discount object
Attributes
- idinteger
Unique numeric identifier for the discount in Recharge.
- applies_toobject
An object encompassing data that limits discount application on the basis of an associated record (such as customer, products, etc.).
Show object attributes - channel_settingsobject
A list of channel objects containing information regarding discount behaviors for each.
If you’re on the SCI Platform, checkout channel will not apply during Shopify checkouts.Show object attributes - codestring
The code used to apply the discount.
- created_atdatetime
The date and time when the discount was created.
- ends_atdatetime
The expiration timestamp of the discount. Past this time the
Discount
can no longer be redeemed. Afterends_at
thestatus
of theDiscount
will go fromactive
todisabled
.Recurring discounts applied prior to their
ends_at
time will still apply. - external_discount_idobject
An object containing external ids of the discount.
Show object attributes - first_time_customer_restrictionboolean
Discount can be used on checkout for customer that still don’t exist in Recharge database.
- prerequisite_subtotal_minstring
The minimum cart subtotal needed for the discount to be applicable.
- starts_atdatetime
The date when the discount becomes active.
When not specified on creation
starts_at
will default tonull
which translates into no restrictions. - statusstring
Possible values: enabled, disabled, fully_disabled
The status of the discount. Value can be:
*enabled
discount is active to be applied
*disabled
discount can’t be applied on new purchases. Discount will remain on existing charges to which it has already been applied.
*fully_disabled
discount can no longer be applied. In addition,Discount
is removed from every queued charge.disabled
andfully_disabled
can both be reverted toenabled
. However once aDiscount
has been updated tofully_disabled
it will be removed from allCharges
it had been applied to. This removal fromCharges
is irreversible. - usage_limitsobject
An object containing limitations on a discount based on usage_counts results
Show object attributes - updated_atdatetime
The date and time when the discount was last updated.
- valuestring
The discounted value to be applied.
- value_typestring
Possible values: fixed_amount, percentage, shipping
Type of discount mechanic.
Error related attributes
More Attributes
{
"discount": {
"applies_to": {
"ids": [],
"purchase_item_type": "ALL",
"resource": null
},
"channel_settings": {
"api": {
"can_apply": true
},
"checkout_page": {
"can_apply": true
},
"customer_portal": {
"can_apply": true
},
"merchant_portal": {
"can_apply": true
}
},
"code": "TEST_DISCOUNT",
"created_at": "2021-04-01T16:13:53+00:00",
"ends_at": null,
"external_discount_id": {
"ecommerce": "1642443555555"
},
"external_discount_source": null,
"id": 25463058,
"prerequisite_subtotal_min": null,
"starts_at": null,
"status": "enabled",
"updated_at": "2021-04-13T22:24:59+00:00",
"usage_limits": {
"first_time_customer_restriction": false,
"max_subsequent_redemptions": null,
"one_application_per_customer": false
},
"value": "20.00",
"value_type": "percent"
}
}
Create a discount
Create a new discount.
Body Parameters
- applies_toobject
An object encompassing data that limits discount application on the basis of an associated record (such as customer, products, etc.)
Show object attributes - channel_settingsobject
A list of channel objects containing information regarding discount behaviors for each.
* can_apply: a boolean to indicate if the discount may be applied using the associated channel (defaults to true for all channels).Show object attributes - codestring* Required
The code used to apply the discount.
- ends_atdatetime
The expiration timestamp of the discount. Past this time the
Discount
can no longer be redeemed. Afterends_at
thestatus
of theDiscount
will go fromactive
todisabled
.Recurring discounts applied prior to their
ends_at
time will still apply. - prerequisite_subtotal_mininteger
The minimum cart subtotal needed for the discount to be applicable.
- starts_atdatetime
The date when the discount becomes
ACTIVE
.When not specified on creation
starts_at
will default tonull
which translates into no restrictions. - statusstring
Possible values: enabled, disabled, fully_disabled
The status of the discount.
- usage_limitsobject
Sets the limit on the number of times a discount can be used by all customers.
Show object attributes - valuestring* Required
The discounted value to be applied.
- value_typestring* Required
Possible values: fixed_amount, percentage, shipping
The discount type.
More Parameters
Responses
- 201
successful response
Show response object
curl 'https://api.rechargeapps.com/discounts' \
-H 'Content-Type: application/json' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d '{
"applies_to": {
"purchase_item_type": "ALL"
},
"channel_settings": {
"api": {
"can_apply": true
},
"checkout_page": {
"can_apply": true
},
"customer_portal": {
"can_apply": true
},
"merchant_portal": {
"can_apply": true
}
},
"code": "Discount1",
"status": "enabled",
"usage_limits": {
"first_time_customer_restriction": false,
"one_application_per_customer": false
},
"value": "100.00",
"value_type": "percentage"
}'
{
"discount": {
"id": 59568555,
"applies_to": {
"ids": [],
"purchase_item_type": "ALL",
"resource": null
},
"channel_settings": {
"api": {
"can_apply": true
},
"checkout_page": {
"can_apply": true
},
"customer_portal": {
"can_apply": true
},
"merchant_portal": {
"can_apply": true
}
},
"code": "Discount1",
"created_at": "2021-07-26T19:16:17+00:00",
"ends_at": null,
"external_discount_id": {
"ecommerce": null
},
"external_discount_source": null,
"prerequisite_subtotal_min": null,
"starts_at": null,
"status": "enabled",
"updated_at": "2021-07-26T19:16:17+00:00",
"usage_limits": {
"first_time_customer_restriction": false,
"max_subsequent_redemptions": null,
"one_application_per_customer": false
},
"value": "100.00",
"value_type": "percentage"
}
}
Retrieve a discount
Retrieve a single Recharge Discount
Responses
- 200
successful response
Show response object
curl 'https://api.rechargeapps.com/discounts/1234567' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token'
{
"discount": {
"id": 59568555,
"applies_to": {
"ids": [],
"purchase_item_type": "ALL",
"resource": null
},
"channel_settings": {
"api": {
"can_apply": true
},
"checkout_page": {
"can_apply": true
},
"customer_portal": {
"can_apply": true
},
"merchant_portal": {
"can_apply": true
}
},
"code": "Discount1",
"created_at": "2021-07-26T19:16:17+00:00",
"ends_at": null,
"external_discount_id": {
"ecommerce": null
},
"external_discount_source": null,
"prerequisite_subtotal_min": null,
"starts_at": null,
"status": "enabled",
"updated_at": "2021-07-26T19:16:17+00:00",
"usage_limits": {
"first_time_customer_restriction": false,
"max_subsequent_redemptions": null,
"one_application_per_customer": false
},
"value": "100.00",
"value_type": "percentage"
}
}
Update a discount
You can modify an existing discount to match the specified parameters.
Body Parameters
- applies_toobject
An object encompassing data that limits discount application on the basis of an associated record (such as customer, products, etc.)
Show object attributes - channel_settingsobject
A list of channel objects containing information regarding discount behaviors for each.
* can_apply: a boolean to indicate if the discount may be applied using the associated channel (defaults to true for all channels).Show object attributes - codestring
The code used to apply the discount…
- ends_atdatetime
The expiration timestamp of the discount. Past this time the
Discount
can no longer be redeemed. Afterends_at
thestatus
of theDiscount
will go fromactive
todisabled
.Recurring discounts applied prior to their
ends_at
time will still apply. - prerequisite_subtotal_mininteger
The minimum cart subtotal needed for the discount to be applicable.
- starts_atdatetime
The date when the discount becomes active.
- statusstring
Possible values: enabled, disabled, fully_disabled
The status of the discount. Value can be:
*enabled
discount is active to be applied
*disabled
discount can’t be applied on new purchases. Discount will remain on existing charges to which it has already been applied.
*fully_disabled
discount can no longer be applied. In addition,Discount
is removed from every queued charge.disabled
andfully_disabled
can both be reverted toenabled
. However once aDiscount
has been updated tofully_disabled
it will be removed from allCharges
it had been applied to. This removal fromCharges
is irreversible. - usage_limitsobject
Sets the limit on the number of times a discount can be used by all customers.
Show object attributes - valuestring
The discounted value to be applied.
- value_typestring
Possible values: fixed_amount, percentage, shipping
The discount type.
More Parameters
Responses
- 200
successful response
Show response object
curl -X PUT 'https://api.rechargeapps.com/discounts/11127406' \
-H 'Content-Type: application/json' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d '{
"usage_limits": {},
"starts_at": "2021-11-01T00:00:00+00:00"
}'
{
"discount": {
"id": 59568555,
"applies_to": {
"ids": [],
"purchase_item_type": "ALL",
"resource": null
},
"channel_settings": {
"api": {
"can_apply": true
},
"checkout_page": {
"can_apply": true
},
"customer_portal": {
"can_apply": true
},
"merchant_portal": {
"can_apply": true
}
},
"code": "Discount1",
"created_at": "2021-07-26T19:16:17+00:00",
"ends_at": null,
"external_discount_id": {
"ecommerce": null
},
"external_discount_source": null,
"prerequisite_subtotal_min": null,
"starts_at": null,
"status": "enabled",
"updated_at": "2021-07-26T19:16:17+00:00",
"usage_limits": {
"first_time_customer_restriction": false,
"max_subsequent_redemptions": null,
"one_application_per_customer": false
},
"value": "100.00",
"value_type": "percentage"
}
}
Delete a discount
Delete a discount
For safety reasons, to delete a discount you will need to set its status to fully_disabled
.
Responses
- 204
Content Deleted: The server has successfully fulfilled the request and deleted the desired object and there is no content to send in the response body.
Show response object
curl -X DELETE 'https://api.rechargeapps.com/discounts/12081717' \
-H 'X-Recharge-Access-Token: your_api_token' \
-H 'X-Recharge-Version: 2021-11'
{}
List discounts
Return a list of discounts in your store.
HTTP request examples
GET /discounts
GET /discounts?created_at_max=2017-10-01
GET /discounts?created_at_max=2017-10-01
GET /discounts?created_at_min=2017-10-01
GET /discounts?discount_code=Discount
GET /discounts?discount_type=percentage
GET /discounts?updated_at_min=2017-10-01
Query Parameters
- created_at_maxstring
Get all discounts created before a specified date.
- created_at_minstring
Get all discounts created after a specified date.
- discount_codestring
Search for a particular discount code.
- value_typestring
Possible values: percentage, fixed_amount, shipping
Filter based on type of discount.
- idsstring
Filter discounts by id. If passing multiple values, must be comma separated. Non-integer values will result in a 422 error
- limitstring
Default: 50
Max: 250
Number of results to return.
- pagestring*Deprecated
Default: 1
Page to show.
Page-based pagination has been deprecated but still available to use for pages up to a 100. If you need data past this point, use cursor pagination.
- statusstring
Possible values: enabled, disabled, fully_disabled
Returns all discounts with status
enabled
,disabled
orfully_disabled
. - updated_at_maxstring
Get all discounts updated before a specified date.
- updated_at_minstring
Get all discounts updated after a specified date.
More Parameters
Responses
- 200
successful response
Show response object
curl -X GET 'https://api.rechargeapps.com/discounts' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token'
{
"next_cursor": null,
"previous_cursor": null,
"discounts": [
{
"id": 59568555,
"applies_to": {
"ids": [],
"purchase_item_type": "ALL",
"resource": null
},
"channel_settings": {
"api": {
"can_apply": true
},
"checkout_page": {
"can_apply": true
},
"customer_portal": {
"can_apply": true
},
"merchant_portal": {
"can_apply": true
}
},
"code": "Discount1",
"created_at": "2021-07-26T19:16:17+00:00",
"ends_at": null,
"external_discount_id": {
"ecommerce": null
},
"external_discount_source": null,
"prerequisite_subtotal_min": null,
"starts_at": null,
"status": "enabled",
"updated_at": "2021-07-26T19:16:17+00:00",
"usage_limits": {
"first_time_customer_restriction": false,
"max_subsequent_redemptions": null,
"one_application_per_customer": false
},
"value": "100.00",
"value_type": "percentage"
}
]
}
Metafields
Metafields allow users to add additional information to other resources. They can be used for adding custom fields to objects, and are useful for storing specialized information.
The metafield object
Metafields feature allows to add additional information to other resources. They can be used for adding custom fields to objects, and are useful for storing specialized information.
You can extend the responses of some of these objects by appending the include?=metafields
as part of your GET
request.
cf. Extending responses for more info.
Attributes
- idinteger
Unique numeric identifier for the metafield.
- created_atdatetime
The date and time when the metafield was created.
- descriptionstring
Description of the metafield.
- keystring
The name of the metafield.
- namespacestring
A category or container that differentiates your metadata from other metafields.
- owner_idstring
Unique numeric identifier of the
owner_resource
. - owner_resourcestring
Possible values: address, store, customer, subscription, order, charge
Objects which support
Metafields
- updated_atdatetime
The date and time when the metafield was last updated.
- valuestring
The content of the metafield.
- value_typestring
Possible values: string, integer, json_string
The type of the value parameter.
Error related attributes
More Attributes
{
"metafield": {
"id": 33,
"created_at": "2018-11-07T14:00:10",
"description": "customer_phone_number",
"key": "phone_number",
"namespace": "personal_info",
"owner_id": 18301938,
"owner_resource": "customer",
"updated_at": "2018-11-07T14:00:10",
"value": "3103103101",
"value_type": "integer"
}
}
Create a metafield
Creates a metafield for a specific object.
There is a limit of 50 metafields per owner_id
.
You need to have WRITE
rights to the resource you want to create a Metafield
for.
Body Parameters
- descriptionstring
Description of the metafield.
- keystring* Required
The name of the metafield.
- namespacestring* Required
A category or container that differentiates your metadata from other metafields.
- owner_idstring* Required
Unique numeric identifier of the owner resource.
- owner_resourcestring* Required
Possible values: address, store, customer, subscription, order, charge
The owner of the resource.
- valuestring* Required
The content of the metafield.
- value_typestring* Required
Possible values: string, integer, json_string
The type of the value parameter.
More Parameters
Responses
- 200
successful response
Show response object
curl 'https://api.rechargeapps.com/metafields' \
-H 'Content-Type: application/json' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d '{
"metafield": {
"description": "customer_phone_number",
"key": "phone_number",
"namespace": "personal_info",
"owner_resource": "customer",
"owner_id": 18301938,
"value_type": "integer",
"value": "3103103101"
}
}'
{
"metafield": {
"id": 33,
"created_at": "2018-11-07T14:00:10",
"description": "customer_phone_number",
"key": "phone_number",
"namespace": "personal_info",
"owner_id": 18301938,
"owner_resource": "customer",
"updated_at": "2018-11-07T14:00:10",
"value": "3103103101",
"value_type": "integer"
}
}
Retrieve a metafield
Retrieves a single metafield based on a specified id.
HTTP request examples
GET /metafields/<metafield_id>
In addition to that, you can retrieve them by
subscription_id
by using: GET /metafields?owner_resource=subscription&owner_id=<subscription_id>
Responses
- 200
successful response
Show response object
curl 'https://api.rechargeapps.com/metafields/33' \
-H 'X-Recharge-Access-Token: your_api_token' \
-H 'X-Recharge-Version: 2021-11'
{
"metafield": {
"id": 33,
"created_at": "2018-11-07T14:00:10",
"description": "customer_phone_number",
"key": "phone_number",
"namespace": "personal_info",
"owner_id": 18301938,
"owner_resource": "customer",
"updated_at": "2018-11-07T14:00:10",
"value": "3103103101",
"value_type": "integer"
}
}
Update a metafield
Updates a metafield based on a specified owner_resource
.
Body Parameters
- descriptionstring
Description of the metafield.
- owner_idstring
Unique numeric identifier of the resource.
- owner_resourcestring
Possible values: address, store, customer, subscription, order, charge
The owner of the resource can be one of the following values:
address
,store
,customer
,subscription
,order
,charge
. There may be more objects added in the future. - valuestring
The content of the metafield.
- value_typestring
Possible values: string, integer, json_string
The type of the value parameter.
More Parameters
Responses
- 201
OK: The request was successful, created a new resource, and resource created is in the body.
Show response object
curl -X PUT 'https://api.rechargeapps.com/metafields/33' \
-H 'Content-Type: application/json' \
-H 'X-Recharge-Access-Token: your_api_token' \
-H 'X-Recharge-Version: 2021-11' \
-d '{
"metafield": {
"description": "phone_number_of_customer",
"owner_id": 18293088,
"owner_resource": "customer",
"value": "0333103133",
"value_type": "integer"
}
}'
{
"metafield": {
"id": 33,
"created_at": "2018-11-07T14:00:10",
"description": "customer_phone_number",
"key": "phone_number",
"namespace": "personal_info",
"owner_id": 18301938,
"owner_resource": "customer",
"updated_at": "2018-11-07T14:00:10",
"value": "3103103101",
"value_type": "integer"
}
}
Delete a metafield
Delete a metafield based on a specified id.
Responses
- 204
Content Deleted: The server has successfully fulfilled the request and deleted the desired object and there is no content to send in the response body.
Show response object
curl -X DELETE 'https://api.rechargeapps.com/metafields/6' \
-H 'X-Recharge-Access-Token: your_api_token' \
-H 'X-Recharge-Version: 2021-11'
{}
List metafields
Retrieves a list of metafields.
HTTP request examples
GET /metafields?owner_resource=<owner_resource>
GET /metafields?owner_resource=subscription&owner_id=<subscription_id>
GET /metafields?owner_resource=customer&owner_id=<customer_id>
GET /metafields?owner_resource=store&owner_id=<store_id>
When getting metafields, you must specify owner_resource in the URL. It can be
address
, store
, customer
, subscription
, order
, charge
You can list metafields that are created for all subscription objects using:
GET /metafields?owner_resource=subscription
- **owner_id**
in the response will be the ID of the subscription.
Query Parameters
- limitstring
Default: 50
Max: 250
Amount of results.
(default:50) (maximum: 250). - namespacestring
A category or container that differentiates your metadata from other metafields.
- owner_idstring
Unique numeric identifier of the owner resource.
- owner_resourcestring
Possible values: address, store, customer, subscription, order, charge
The owner of the resource can be one of the following values:
address
,store
,customer
,subscription
,order
,charge
. There may be more objects added in the future. - pagestring*Deprecated
Default: 1
Page to show.
Page-based pagination has been deprecated but still available to use for pages up to a 100. If you need data past this point, use cursor pagination.
More Parameters
Responses
- 200
successful response
Show response object
curl 'https://api.rechargeapps.com/metafields' \
-H 'X-Recharge-Access-Token: your_api_token' \
-H 'X-Recharge-Version: 2021-11' \
-d owner_resource=address \
-d limit=3 -G
{
"next_cursor": "next_cursor",
"previous_cursor": "previous_cursor",
"metafields": [
{
"id": 33,
"created_at": "2018-11-07T14:00:10",
"description": "customer_phone_number",
"key": "phone_number",
"namespace": "personal_info",
"owner_id": 18301938,
"owner_resource": "customer",
"updated_at": "2018-11-07T14:00:10",
"value": "3103103101",
"value_type": "integer"
},
{
"id": 15,
"created_at": "2018-11-05T12:59:30",
"description": "desc lorem ipsum",
"key": "marjan",
"namespace": "nmsp2c",
"owner_id": 17868054,
"owner_resource": "customer",
"updated_at": "2018-11-05T15:48:42",
"value": "5",
"value_type": "integer"
},
{
"id": 9,
"created_at": "2018-11-05T12:47:27",
"description": "desc lorem ipsum",
"key": "marjan",
"namespace": "nmspc",
"owner_id": 17868054,
"owner_resource": "customer",
"updated_at": "2018-11-05T12:47:27",
"value": "5",
"value_type": "integer"
}
]
}
Notifications
The Customer Notifications resource is used to dispatch email notifications to customers within Recharge. The endpoint uses your configured Recharge email templates, and will dispatch an email to the address associated with the indicated customer_id
. Some email templates require variable values, which are sent through the Customer Notifications resource via the template_vars attribute. See below examples for all available template types.
Send email notification
Sends a notification email to the customer with the customer_id
indicated in the route. To view the upcoming charge email template, visit the Notifications section in your Recharge Merchant Admin Portal.
Body Parameters
- typeinteger* Required
Identifies the type of the notifications. Value can be email only.
- template_typestring* Required
Possible values: upcoming_charge, get_account_access, shopify_update_payment_information
Identifies the type of the email notifications.
- template_varsobject
An object containing the necessary template variables for this email template type.
Show object attributes
More Parameters
Responses
- 200
successful response
Show response object
curl 'https://api.rechargeapps.com/customers/18819267/notifications' \
-H 'X-Recharge-Version: 2021-11' \
-H 'Content-Type: application/json' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d '{
"type": "email",
"template_type": "upcoming_charge",
"template_vars":{
"address_id":1234567890,
"charge_id":9876543210
}
}'
{}
Onetimes
Onetimes represent non-recurring line items on a QUEUED
Charge
.
The onetime object
Attributes
- idinteger
Unique numeric identifier for the
Onetime
purchase. - address_idinteger
Unique numeric identifier for the address the
Onetime
Purchase is associated with (cannot be used withnext_charge_scheduled_at
) - created_atdatetime
The time the
Onetime
item was first created. - customer_idinteger
Unique numeric identifier for the customer the
Onetime
purchase is tied to. - external_product_idstring
An object containing the product id as it appears in external platforms.
Show object attributes - external_variant_idstring
An object containing the variant id as it appears in external platforms.
Show object attributes - is_cancelledboolean
Flag indicating if the the onetime is cancelled.
- next_charge_scheduled_atdatetime
Date of the
Onetime
purchase execution.Cannot be used with
add_to_next_charge
- pricestring
The price of the item before discounts, taxes, or shipping have been applied.
- product_titlestring
The name of the product in a shop’s catalog.
- propertiesarray
An array containing key value pairs for any supplementary data.
Show object attributes - quantityinteger
The number of items in the
Onetime
purchase. - skustring
A unique identifier of the item in the fulfillment.
- sku_overrideboolean
Flag that is automatically updated to
true
when SKU is passed onPOST
orPUT
.When
sku_override
istrue
, thesku
on theOnetime
will be used to generateCharges
andOrders
.
Whensku_override
isfalse
, Recharge will dynamically fetch thesku
from the correspondingexternal_platform_variant
. - updated_atstring
The time the
Onetime
purchase was last updated. - variant_titlestring
The name of the variant in a shop’s catalog.
Error related attributes
More Attributes
{
"onetime": {
"id": 16909886,
"address_id": 21317826,
"customer_id": 18819267,
"created_at": "2018-11-14T11:20:05+00:00",
"external_product_id": {
"ecommerce": "4950280863846"
},
"external_variant_id": {
"ecommerce": "32139793137766"
},
"is_cancelled": false,
"next_charge_scheduled_at": "2018-12-17T00:00:00+00:00",
"price": null,
"product_title": "SuperKiwi ONETIME",
"properties": [
{
"name": "grind",
"value": "drip"
}
],
"quantity": 1,
"sku": "TOM0001",
"sku_override": false,
"updated_at": "2018-11-14T11:20:05+00:00",
"variant_title": "Blue star"
}
}
Create a onetime
Create a new Onetime
.
Body Parameters
- address_idinteger* Required
Unique numeric identifier for the address the one time purchase is associated with.
- add_to_next_chargeboolean
Instructs to add the
Onetime
to the next charge scheduled under thisAddress
. - external_product_idobject
Unique numeric identifier of the product in external platform.
Show object attributes - external_variant_idobject* Required
Unique numeric identifier of the product variant in external platform.
Show object attributes - next_charge_scheduled_atdatetime* Required
This will set the charge date of a new Onetime purchase.
Cannot be used with
add_to_next_charge
- pricestring* Required
The price of the product.
- product_titlestring* Required
The name of the product.
- propertiesstring
List of properties.
- quantityinteger* Required
The quantity of the product.
- skustring
A unique identifier of the item in the fulfillment.
More Parameters
Responses
- 201
OK: The request was successful, created a new resource, and resource created is in the body.
Show response object
curl 'https://api.rechargeapps.com/onetimes' \
-H 'X-Recharge-Version: 2021-11' \
-H 'Content-Type: application/json' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d '{
"address_id": 45154492,
"next_charge_scheduled_at": "2021-12-17",
"price": 6,
"product_title": "ABC Shirt",
"properties": [
{
"name": "Color",
"value": "Blue"
},
{
"name": "Size",
"value": "Medium"
}
],
"quantity": 1,
"external_variant_id": {
"ecommerce": "3844892483"
}
}'
{
"onetime": {
"id": 156155818,
"address_id": 45154492,
"customer_id": 40565990,
"created_at": "2021-05-24T19:14:25+00:00",
"external_product_id": {
"ecommerce": "4950280863846"
},
"external_variant_id": {
"ecommerce": "32139793137766"
},
"is_cancelled": false,
"next_charge_scheduled_at": "2021-12-17T05:00:00+00:00",
"price": "6.00",
"product_title": "ABC Shirt",
"properties": [
{
"name": "Color",
"value": "Red"
}
],
"quantity": 1,
"sku": "TOM0001",
"sku_override": false,
"updated_at": "2021-05-24T19:30:14+00:00",
"variant_title": "Blue star"
}
}
Retrieve a onetime
Retrieve a Onetime.
Responses
- 200
successful response
Show response object
curl 'https://api.rechargeapps.com/onetimes/16909886' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token'
{
"onetime": {
"id": 16909886,
"address_id": 45154492,
"created_at": "2021-05-24T19:14:25+00:00",
"customer_id": 40565990,
"external_product_id": {
"ecommerce": "4950280863846"
},
"external_variant_id": {
"ecommerce": "32139793137766"
},
"is_cancelled": false,
"next_charge_scheduled_at": "2021-12-17T05:00:00+00:00",
"price": "6.00",
"product_title": "ABC Shirt",
"properties": [
{
"name": "Color",
"value": "Blue"
}
],
"quantity": 1,
"sku": "TOM0001",
"sku_override": false,
"updated_at": "2021-05-24T19:30:14+00:00",
"variant_title": "Blue star"
}
}
Update a onetime
Update an existing Onetime
item.
Body Parameters
- address_idinteger
Unique numeric identifier for the address the
Onetime
Purchase is associated with (cannot be used withnext_charge_scheduled_at
) - next_charge_scheduled_atdatetime
This will set the charge date of a new
Onetime
purchase. - pricestring
The price of the product.
- product_titlestring
The name of the product.
- propertiesarray
List of properties.
Show object attributes - quantityinteger
The quantity of the product.
- external_variant_idobject
Unique numeric identifier of the product variant.
Show object attributes - skustring
A unique alphanumeric identifier of the item in the fulfillment.
- variant_titlestring
The name of the product variant.
More Parameters
Responses
- 200
successful response
Show response object
curl -x PUT 'https://api.rechargeapps.com/onetimes/16909886' \
-H 'X-Recharge-Version: 2021-11' \
-H 'Content-Type: application/json' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d '{"quantity": 1}'
{
"onetime": {
"id": 16909886,
"address_id": 45154492,
"created_at": "2021-05-24T19:14:25+00:00",
"customer_id": 40565990,
"external_product_id": {
"ecommerce": "4950280863846"
},
"external_variant_id": {
"ecommerce": "32139793137766"
},
"is_cancelled": false,
"next_charge_scheduled_at": "2021-12-17T05:00:00+00:00",
"price": "6.00",
"product_title": "ABC Shirt",
"properties": [
{
"name": "Color",
"value": "Blue"
}
],
"quantity": 1,
"sku": "TOM0001",
"sku_override": false,
"updated_at": "2021-05-24T19:30:14+00:00",
"variant_title": "Blue star"
}
}
Delete a onetime
Delete a Onetime
.
Responses
- 204
Content Deleted: The server has successfully fulfilled the request and deleted the desired object and there is no content to send in the response body.
Show response object
curl -X DELETE 'https://api.rechargeapps.com/onetimes/16665185' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token'
{}
List onetimes
Returns a list of all Onetime
products from store.
Query Parameters
- address_idstring
Return the onetimes linked to the given
address_id
. - address_idsstring
Return the onetimes linked to the given comma-separated list of
address_ids
. - created_at_maxdatetime
Return the onetimes created before the given date.
- created_at_mindatetime
Return the onetimes created after the given date.
- customer_idstring
Return the onetimes linked to the given Recharge
customer_id
. - include_cancelledboolean
If
true
the response will include the cancelledOnetimes
as well as the others. - limitstring
Default: 50
Max: 250
The number of results.
- pagestring*Deprecated
Default: 1
The page to show.
Page-based pagination has been deprecated but still available to use for pages up to a 100. If you need data past this point, use cursor pagination.
- external_variant_idstring
Return the one time purchases linked to the given external platform product
variant_id
. - updated_at_maxdatetime
Return the onetimes updated before the given date.
- updated_at_mindatetime
Return the onetimes updated after the given date.
- idsarray
Comma-separated list of one time purchase ids to filter.
More Parameters
Responses
- 200
successful response
Show response object
curl 'https://api.rechargeapps.com/onetimes' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d limit=3 -G
{
"next": "next_cursor",
"previous": "previous_cursor",
"onetimes": [
{
"id": 16909886,
"address_id": 45154492,
"created_at": "2021-05-24T19:14:25+00:00",
"customer_id": 40565990,
"external_product_id": {
"ecommerce": "4950280863846"
},
"external_variant_id": {
"ecommerce": "32139793137766"
},
"is_cancelled": false,
"next_charge_scheduled_at": "2021-12-17T05:00:00+00:00",
"price": "6.00",
"product_title": "ABC Shirt",
"properties": [
{
"name": "Color",
"value": "Blue"
}
],
"quantity": 1,
"sku": "TOM0001",
"sku_override": false,
"updated_at": "2021-05-24T19:30:14+00:00",
"variant_title": "Blue star"
}
]
}
Orders
An order is created after a Charge
is successfully processed. The Order
contains all the same json data as the Charge
. In case of a prepaid order creation, the order will be queued for a particular date and submitted on that date to the external platform.
The order object
Attributes
- idinteger
The unique numeric identifier for the order.
- address_idinteger
The id of the associated
Address
within Recharge. - billing_addressobject
The billing address at the time the order was created. See
Addresses
for detailed address information.Show object attributes - chargeobject
An object containing parameters of the
Charge
.Show object attributes - client_detailsobject
Details of the access method used by the purchase.
Show object attributes - created_atdatetime
The date when the order was created.
- currencystring
The currency of the payment used to create the order.
- customerobject
Object that contains information about the
Customer
.Show object attributes - discountsarray
An array of
Discounts
associated with theOrder
.Show object attributes - external_cart_tokenstring
The cart token as it appears in an external system.
- external_order_idobject
An object containing external order ids.
Show object attributes - external_order_number
An object containing the external order numbers.
Show object attributes - is_prepaidboolean
A boolean representing if this
Order
is generated from a prepaid purchase. - line_itemsarray
A list of
line_item
objects.Show object attributes - notestring
Notes associated with the
Order
. - order_attributesarray
An array of name value pairs of note attributes on the
Order
.Show object attributes - processed_atdatetime
The date time that the associated charge was processed at.
- scheduled_atdatetime
The date time of when the associated charge is/was scheduled to process.
- shipping_addressobject
The shipping address where the order will be shipped. See
Addresses
for detailedAddress
information.Show object attributes - shipping_linesarray
An array of shipping lines associated with the order.
Show object attributes - statusstring
Possible values: success, error, queued, cancelled
The status of creating the
Order
. - subtotal_pricestring
The subtotal price (sum of all line items * their quantity) of the order less discounts.
- tagsstring
A comma separated list of tags on the
Order
. - tax_linesarray
An array of tax lines that apply to the
Order
.Show object attributes - taxableboolean
A boolean indicator of the taxability of the
Order
. - total_discountsstring
The total discounted dollar value of the
Order
. - total_dutiesstring
The total cost of duties for the order.
- total_line_items_priceinteger
The total price of all line items of the
Order
. - total_pricestring
The total amount due of the
Order
. - total_refundsstring
The total dollar amount of refunds associated with the
Order
. - total_taxstring
The total tax due associated with the
Order
. - total_weight_gramsinteger
The total weight of the order in grams.
- typestring
Possible values: checkout, recurring
An indicator of the order’s type.
- updated_atdatetime
The date time at which the order was most recently updated.
Error related attributes
More Attributes
{
"order": {
"id": 70071255,
"address_id": 4029306,
"billing_address": {
"address1": "1933 Manning",
"address2": "204",
"city": "los angeles",
"company": "bootstrap",
"country_code": "US",
"first_name": "mike",
"last_name": "flynn",
"phone": "3103103101",
"province": "California",
"zip": "90025"
},
"charge": {
"id": 97376832,
"external_transaction_id": {
"payment_processor": "ch_1HzWElJ2zqHvZRd1TWKFFqDR"
}
},
"client_details": {
"browser_ip": "192.168.0.1",
"user_agent": "safari webkit"
},
"created_at": "2018-11-08T08:08:09+00:00",
"currency": "usd",
"customer": {
"id": 12354,
"email": "example@email.com",
"external_customer_id": {
"ecommerce": "382028302"
},
"hash": "7e706455cbd13e40"
},
"discounts": [
{
"id": 123456,
"code": "TESTCODE10",
"value": "10.00",
"value_type": "fixed_amount"
}
],
"external_cart_token": "aeic8101918fs0f8810",
"external_order_id": {
"ecommerce": "123496878536413"
},
"external_order_number": {
"ecommerce": "125"
},
"hash": "42039120ee0e6cfa5c97805",
"is_prepaid": false,
"line_items": [
{
"purchase_item_id": 365974856,
"external_inventory_policy": "decrement_obeying_policy",
"external_product_id": {
"ecommerce": "456123789"
},
"external_variant_id": {
"ecommerce": "4569876413"
},
"grams": 454,
"images": {
"large": "https://cdn.shopify.com/s/files/1/0683/1951/products/milk2_large.jpeg",
"medium": "https://cdn.shopify.com/s/files/1/0683/1951/products/milk2_medium.jpeg",
"original": "https://cdn.shopify.com/s/files/1/0683/1951/products/milk2.jpeg",
"small": "https://cdn.shopify.com/s/files/1/0683/1951/products/milk2_small.jpeg"
},
"original_price": "10.00",
"properties": [
{
"name": "grind",
"value": "drip"
},
{
"name": "size",
"value": "medium"
}
],
"purchase_item_type": "subscription",
"quantity": 4,
"sku": "TOM0001",
"tax_due": "3.80",
"tax_lines": [
{
"price": "0.993",
"rate": "0.0725",
"unit_price": "0.331",
"title": "CA State Tax"
},
{
"price": "0.308",
"rate": "0.0225",
"unit_price": "0.102",
"title": "LA County Tax"
}
],
"taxable": true,
"taxable_amount": "10.00",
"title": "Shirt bundle",
"total_price": "43.80",
"unit_price": "10.00",
"unit_price_includes_tax": false,
"variant_title": "Blue t-shirts"
}
],
"note": "next order #3 - Subscription Recurring Order",
"order_attributes": [
{
"name": "subscription_cycle_count_min",
"value": "3"
}
],
"processed_at": null,
"scheduled_at": "2022-11-16T00:00:00+00:00",
"shipping_address": {
"address1": "1933 Manning",
"address2": "204",
"city": "los angeles",
"company": "bootstrap",
"country_code": "US",
"first_name": "mike",
"last_name": "flynn",
"phone": "3103103101",
"province": "California",
"zip": "90025"
},
"shipping_lines": [
{
"code": "Standard Shipping",
"price": "4.90",
"taxable": true,
"tax_lines": [
{
"price": "0.360",
"rate": "0.0725",
"title": "CA State Tax"
},
{
"price": "0.110",
"rate": "0.0225",
"title": "LA County Tax"
}
],
"title": "Standard Shipping"
}
],
"status": "cancelled",
"subtotal_price": "40.00",
"tags": "Prepaid, Subscription, Subscription Recurring Order",
"tax_lines": [
{
"price": "0.950",
"rate": 0.0725,
"title": "CA State Tax"
},
{
"price": "0.335",
"rate": 0.0225,
"title": "Los Angeles County Tax"
}
],
"taxable": true,
"total_discounts": "10.00",
"total_duties": "0.0",
"total_line_items_price": "40.00",
"total_price": "38.22",
"total_refunds": "0.00",
"total_tax": "3.32",
"total_weight_grams": 454,
"type": "recurring",
"updated_at": "2020-12-20T13:25:52+00:00"
}
}
Retrieve an order
Retrieve one Order
using the Recharge order_id
.
Responses
- 200
successful response
Show response object
curl 'https://api.rechargeapps.com/orders/70071255' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token'
{
"order": {
"id": 210987092,
"address_id": 42171447,
"billing_address": {
"address1": "601 SW Washing St.",
"address2": "",
"city": "Portland",
"company": "Fake Company Billing",
"country_code": "US",
"first_name": "Fake Billing First",
"last_name": "Fake Billing Last",
"phone": "888-888-8888",
"province": "Oregon",
"zip": "97205"
},
"charge": {
"id": 272382456,
"external_transaction_id": {
"payment_processor": "ch_1HzWElJ2zqHvZRd1TWKFFqDR"
}
},
"client_details": {
"browser_ip": "192.168.0.1",
"user_agent": "safari webkit"
},
"created_at": "2020-12-17T18:50:25+00:00",
"currency": "usd",
"customer": {
"id": 37657002,
"email": "test@test.com",
"external_customer_id": {
"ecommerce": "382028302"
},
"hash": "7e706455cbd13e40"
},
"discounts": [
{
"id": 12345,
"code": "TESTCODE10",
"value": 10,
"value_type": "fixed_amount"
}
],
"error": null,
"external_cart_token": "aeic8101918fs0f8810",
"external_order_id": {
"ecommerce": "27117383938"
},
"external_order_number": {
"ecommerce": "1938"
},
"is_prepaid": false,
"line_items": [
{
"purchase_item_id": 63898947,
"external_inventory_policy": "decrement_obeying_policy",
"external_product_id": {
"ecommerce": "4381728735283"
},
"external_variant_id": {
"ecommerce": "999999999999"
},
"grams": 454,
"images": {
"large": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h_large.jpg",
"medium": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h_medium.jpg",
"original": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h.jpg",
"small": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h_small.jpg"
},
"original_price": "10.00",
"properties": [
{
"name": "Color",
"value": "Blue"
}
],
"purchase_item_type": "subscription",
"quantity": 1,
"sku": "TOM0001",
"tax_lines": [
{
"price": "0.993",
"rate": "0.0725",
"title": "CA State Tax",
"unit_price": "0.331"
},
{
"price": "0.308",
"rate": "0.0225",
"title": "LA County Tax",
"unit_price": "0.102"
}
],
"taxable": true,
"taxable_amount": "10.00",
"tax_due": "1.30",
"title": "Shirt bundle",
"total_price": "11.30",
"unit_price": "10.00",
"unit_price_includes_tax": false,
"variant_title": "Blue t-shirts"
}
],
"note": "next order in sequence 3",
"order_attributes": [
{
"name": "subscription_cycle_count_min",
"value": "3"
}
],
"processed_at": "2020-12-17T18:50:27+00:00",
"scheduled_at": "2020-12-17T18:50:27+00:00",
"shipping_address": {
"address1": "1030 Barnum Ave",
"address2": "Suite 101",
"city": "Stratford",
"company": "Fake Company",
"country_code": "US",
"first_name": "Fake First",
"last_name": "Fake Last",
"phone": "999-999-9999",
"province": "Connecticut",
"zip": "06614"
},
"shipping_lines": [
{
"code": "Standard",
"price": "4.90",
"taxable": true,
"tax_lines": [
{
"price": "0.355",
"rate": "0.0725",
"title": "CA State Tax"
},
{
"price": "0.110",
"rate": "0.0225",
"title": "Los Angeles County Tax"
}
],
"title": "Standard"
}
],
"status": "success",
"subtotal_price": "10.00",
"tags": "Subscription, Subscription Recurring Order",
"tax_lines": [
{
"price": "0.950",
"rate": "0.0725",
"title": "CA State Tax"
},
{
"price": "0.335",
"rate": "0.0225",
"title": "Los Angeles County Tax"
}
],
"taxable": true,
"total_discounts": "10.00",
"total_duties": "0.0",
"total_line_items_price": "10.00",
"total_price": "14.90",
"total_refunds": "0.00",
"total_tax": "10.00",
"total_weight_grams": 454,
"type": "recurring",
"updated_at": "2020-12-17T18:50:27+00:00"
}
}
Clone an order
You may add additional Orders
onto a success Charge
if it has existing prepaid Orders
by cloning an existing Order
.
Body Parameters
- scheduled_atdatetime* Required
Date in future when this order will be sent.
More Parameters
Responses
- 201
OK: The request was successful, created a new resource, and resource created is in the body.
Show response object
curl 'https://api.rechargeapps.com/orders/70071255/clone' \
-H 'Content-Type: application/json' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d '{"scheduled_at": "2022-11-16"}'
{
"order": {
"id": 210987092,
"address_id": 42171447,
"billing_address": {
"address1": "601 SW Washing St.",
"address2": "",
"city": "Portland",
"company": "Fake Company Billing",
"country_code": "US",
"first_name": "Fake Billing First",
"last_name": "Fake Billing Last",
"phone": "888-888-8888",
"province": "Oregon",
"zip": "97205"
},
"charge": {
"id": 272382456,
"external_transaction_id": {
"payment_processor": "ch_1HzWElJ2zqHvZRd1TWKFFqDR"
}
},
"client_details": {
"browser_ip": "192.168.0.1",
"user_agent": "safari webkit"
},
"created_at": "2020-12-17T18:50:25+00:00",
"currency": "usd",
"customer": {
"id": 37657002,
"email": "test@test.com",
"external_customer_id": {
"ecommerce": "382028302"
},
"hash": "7e706455cbd13e40"
},
"discounts": [
{
"id": 12345,
"code": "TESTCODE10",
"value": 10,
"value_type": "fixed_amount"
}
],
"error": null,
"external_cart_token": "aeic8101918fs0f8810",
"external_order_id": {
"ecommerce": "27117383938"
},
"external_order_number": {
"ecommerce": "1938"
},
"is_prepaid": false,
"line_items": [
{
"purchase_item_id": 63898947,
"external_inventory_policy": "decrement_obeying_policy",
"external_product_id": {
"ecommerce": "4381728735283"
},
"external_variant_id": {
"ecommerce": "999999999999"
},
"grams": 454,
"images": {
"large": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h_large.jpg",
"medium": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h_medium.jpg",
"original": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h.jpg",
"small": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h_small.jpg"
},
"original_price": "10.00",
"properties": [
{
"name": "Color",
"value": "Blue"
}
],
"purchase_item_type": "subscription",
"quantity": 1,
"sku": "TOM0001",
"tax_lines": [
{
"price": "0.993",
"rate": "0.0725",
"title": "CA State Tax",
"unit_price": "0.331"
},
{
"price": "0.308",
"rate": "0.0225",
"title": "LA County Tax",
"unit_price": "0.102"
}
],
"taxable": true,
"taxable_amount": "10.00",
"tax_due": "1.30",
"title": "Shirt bundle",
"total_price": "11.30",
"unit_price": "10.00",
"unit_price_includes_tax": false,
"variant_title": "Blue t-shirts"
}
],
"note": "next order in sequence 3",
"order_attributes": [
{
"name": "subscription_cycle_count_min",
"value": "3"
}
],
"processed_at": "2020-12-17T18:50:27+00:00",
"scheduled_at": "2020-12-17T18:50:27+00:00",
"shipping_address": {
"address1": "1030 Barnum Ave",
"address2": "Suite 101",
"city": "Stratford",
"company": "Fake Company",
"country_code": "US",
"first_name": "Fake First",
"last_name": "Fake Last",
"phone": "999-999-9999",
"province": "Connecticut",
"zip": "06614"
},
"shipping_lines": [
{
"code": "Standard",
"price": "4.90",
"taxable": true,
"tax_lines": [
{
"price": "0.355",
"rate": "0.0725",
"title": "CA State Tax"
},
{
"price": "0.110",
"rate": "0.0225",
"title": "Los Angeles County Tax"
}
],
"title": "Standard"
}
],
"status": "success",
"subtotal_price": "10.00",
"tags": "Subscription, Subscription Recurring Order",
"tax_lines": [
{
"price": "0.950",
"rate": "0.0725",
"title": "CA State Tax"
},
{
"price": "0.335",
"rate": "0.0225",
"title": "Los Angeles County Tax"
}
],
"taxable": true,
"total_discounts": "10.00",
"total_duties": "0.0",
"total_line_items_price": "10.00",
"total_price": "14.90",
"total_refunds": "0.00",
"total_tax": "10.00",
"total_weight_grams": 454,
"type": "recurring",
"updated_at": "2020-12-17T18:50:27+00:00"
}
}
Delay an order
Delay a prepaid Order
by one interval
The id
must be for the next queued Order
for a prepaid Subscription
. The Order
will be delayed by one interval, and all subsequent Orders
and Charges
will be shifted by the same amount.
Responses
- 200
successful response
Show response object
curl -X POST 'https://api.rechargeapps.com/orders/70071255/delay' \
-H 'Content-Type: application/json' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token'
{
"order": {
"id": 70071255,
"address_id": 4029306,
"created_at": "2022-02-04T23:19:52+00:00",
"currency": "usd",
"discounts": [],
"error": null,
"external_cart_token": null,
"is_prepaid": true,
"line_items": [
{
"purchase_item_id": 63898947,
"external_inventory_policy": "decrement_obeying_policy",
"external_product_id": {
"ecommerce": "4381728735283"
},
"external_variant_id": {
"ecommerce": "999999999999"
},
"grams": 454,
"images": {
"large": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h_large.jpg",
"medium": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h_medium.jpg",
"original": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h.jpg",
"small": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h_small.jpg"
},
"original_price": "10.00",
"properties": [
{
"name": "Color",
"value": "Blue"
}
],
"purchase_item_type": "subscription",
"quantity": 1,
"sku": "TOM0001",
"tax_lines": [
{
"price": "0.993",
"rate": "0.0725",
"title": "CA State Tax",
"unit_price": "0.331"
},
{
"price": "0.308",
"rate": "0.0225",
"title": "LA County Tax",
"unit_price": "0.102"
}
],
"taxable": true,
"taxable_amount": "10.00",
"tax_due": "1.30",
"title": "Shirt bundle",
"total_price": "11.30",
"unit_price": "10.00",
"unit_price_includes_tax": false,
"variant_title": "Blue t-shirts"
}
],
"note": null,
"order_attributes": [],
"processed_at": null,
"scheduled_at": "2023-02-07T05:00:00+00:00",
"shipping_lines": [
{
"code": "Standard",
"price": "4.90",
"taxable": true,
"tax_lines": [
{
"price": "0.355",
"rate": "0.0725",
"title": "CA State Tax"
},
{
"price": "0.110",
"rate": "0.0225",
"title": "Los Angeles County Tax"
}
],
"title": "Standard"
}
],
"status": "queued",
"subtotal_price": "10.00",
"tags": "Subscription, Subscription Recurring Order",
"tax_lines": [
{
"price": "0.950",
"rate": "0.0725",
"title": "CA State Tax"
},
{
"price": "0.335",
"rate": "0.0225",
"title": "Los Angeles County Tax"
}
],
"taxable": true,
"total_discounts": "0.00",
"total_duties": "0.0",
"total_line_items_price": "10.00",
"total_price": "14.90",
"total_refunds": "0.00",
"total_tax": "10.00",
"total_weight_grams": 454,
"type": "recurring",
"updated_at": "2023-01-05T22:11:46+00:00"
}
}
Update an order
Updating existing Order
using the order_id
.
Body Parameters
- billing_addressobject
Billing address details.
Show object attributes - customerobject
The key values related with customer.
Show object attributes - line_itemsarray
Line item properties can be updated on Prepaid Queued Orders. To change Queued Charges you must change the parent subscription(s) or address.
Important: When updating
line_items
, you must provide the entire json block that was inline_items
before, as the data provided overrides the entire block and only new parameters will remain.Show object attributes - external_order_idobject
An object containing external order ids.
Show object attributes - scheduled_atdatetime
The date when the order will be shipped.
- shipping_addressobject
Shipping address details.
Show object attributes - statusstring
Possible values: success, error, queued, cancelled
The status of the order
More Parameters
Responses
- 200
successful response
Show response object
curl -X PUT 'https://api.rechargeapps.com/orders/70071255' \
-H 'Content-Type: application/json' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d '{
"billing_address": {
"city": "Los Angeles",
"first_name": "mike",
"last_name": "flynn",
"zip": "90025"
}
}'
{
"order": {
"id": 210987092,
"address_id": 42171447,
"billing_address": {
"address1": "601 SW Washing St.",
"address2": "",
"city": "Portland",
"company": "Fake Company Billing",
"country_code": "US",
"first_name": "Fake Billing First",
"last_name": "Fake Billing Last",
"phone": "888-888-8888",
"province": "Oregon",
"zip": "97205"
},
"charge": {
"id": 272382456,
"external_transaction_id": {
"payment_processor": "ch_1HzWElJ2zqHvZRd1TWKFFqDR"
}
},
"client_details": {
"browser_ip": "192.168.0.1",
"user_agent": "safari webkit"
},
"created_at": "2020-12-17T18:50:25+00:00",
"currency": "usd",
"customer": {
"id": 37657002,
"email": "test@test.com",
"external_customer_id": {
"ecommerce": "382028302"
},
"hash": "7e706455cbd13e40"
},
"discounts": [
{
"id": 12345,
"code": "TESTCODE10",
"value": 10,
"value_type": "fixed_amount"
}
],
"error": null,
"external_cart_token": "aeic8101918fs0f8810",
"external_order_id": {
"ecommerce": "27117383938"
},
"external_order_number": {
"ecommerce": "1938"
},
"is_prepaid": false,
"line_items": [
{
"purchase_item_id": 63898947,
"external_inventory_policy": "decrement_obeying_policy",
"external_product_id": {
"ecommerce": "4381728735283"
},
"external_variant_id": {
"ecommerce": "999999999999"
},
"grams": 454,
"images": {
"large": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h_large.jpg",
"medium": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h_medium.jpg",
"original": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h.jpg",
"small": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h_small.jpg"
},
"original_price": "10.00",
"properties": [
{
"name": "Color",
"value": "Blue"
}
],
"purchase_item_type": "subscription",
"quantity": 1,
"sku": "TOM0001",
"tax_lines": [
{
"price": "0.725",
"rate": "0.0725",
"title": "CA State Tax",
"unit_price": "0.331"
},
{
"price": "0.225",
"rate": "0.0225",
"title": "LA County Tax",
"unit_price": "0.102"
}
],
"taxable": true,
"taxable_amount": "10.00",
"tax_due": "0.95",
"title": "Shirt bundle",
"total_price": "10.95",
"unit_price": "10.00",
"unit_price_includes_tax": false,
"variant_title": "Blue t-shirts"
}
],
"note": "next order in sequence 3",
"order_attributes": [
{
"name": "subscription_cycle_count_min",
"value": "3"
}
],
"processed_at": "2020-12-17T18:50:27+00:00",
"scheduled_at": "2020-12-17T18:50:27+00:00",
"shipping_address": {
"address1": "1030 Barnum Ave",
"address2": "Suite 101",
"city": "Stratford",
"company": "Fake Company",
"country_code": "US",
"first_name": "Fake First",
"last_name": "Fake Last",
"phone": "999-999-9999",
"province": "Connecticut",
"zip": "06614"
},
"shipping_lines": [
{
"code": "Standard",
"price": "4.90",
"taxable": true,
"tax_lines": [
{
"price": "0.355",
"rate": "0.0725",
"title": "CA State Tax"
},
{
"price": "0.110",
"rate": "0.0225",
"title": "Los Angeles County Tax"
}
],
"title": "Standard"
}
],
"status": "success",
"subtotal_price": "10.00",
"tags": "Subscription, Subscription Recurring Order",
"tax_lines": [
{
"price": "0.355",
"rate": "0.0725",
"title": "CA State Tax"
},
{
"price": "0.110",
"rate": "0.0225",
"title": "Los Angeles County Tax"
}
],
"taxable": true,
"total_discounts": "10.00",
"total_line_items_price": "10.00",
"total_price": "5.37",
"total_refunds": "0.00",
"total_tax": "0.47",
"total_weight_grams": 454,
"type": "recurring",
"updated_at": "2020-12-17T18:50:27+00:00"
}
}
Delete an order
You can delete a scheduled order.
Responses
- 204
Content Deleted: The server has successfully fulfilled the request and deleted the desired object and there is no content to send in the response body.
Show response object
curl -X DELETE 'https://api.rechargeapps.com/orders/70071255' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token'
{}
List orders
Returns a list of orders.
HTTP request examples
GET /orders
GET /orders?customer_id=123
GET /orders?address_id=4587216
GET /orders?charge_id=45678542
GET /orders?created_at_min=2016-05-18&created_at_max=2016-06-18
Query Parameters
- address_idstring
Filter orders by address.
GET /orders/?address_id=123
will return all orders for the givenaddress_id
. - charge_idstring
Filter orders by charge.
GET /orders/?charge_id=123
will return all orders for the givencharge_id
. - created_at_maxdatetime
Show orders created before the given date.
- created_at_mindatetime
Show orders created after the given date.
- customer_idstring
Filter orders by customer.
GET /orders/?customer_id=123
will return all orders for the givencustomer_id
- external_customer_idstring
Filter orders by external_customer_id.
- external_order_idstring
Filter orders by external_order_id.
- idsstring
Filter orders by id. If passing multiple values, must be comma separated. Non-integer values will result in a 422 error
- limitstring
Default: 50
Max: 250
The number of results.
- pagestring*Deprecated
Default: 1
The page to show. Default is 1.
Page-based pagination has been deprecated but still available to use for pages up to a 100. If you need data past this point, use cursor pagination.
- scheduled_at_maxdatetime
Show orders scheduled before the given date.
- scheduled_at_mindatetime
Show orders scheduled after the given date.
- has_external_orderstring
Filter orders with/without external_order_id.
- statusstring
Possible values: success, queued, error, refunded, skipped
Filter orders by status.
- typestring
Possible values: checkout, recurring
Filter orders by type.
GET /api/orders/?type=recurring
will return all orders for the given type. - purchase_item_idstring
Filter orders by subscription or onetime.
- updated_at_maxdatetime
Show orders updated before the given date.
- updated_at_mindatetime
Show orders updated after the given date.
More Parameters
Responses
- 200
successful response
Show response object
curl 'https://api.rechargeapps.com/orders' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d 'limit=3' -G
{
"next": "next_cursor",
"previous": "previous_cursor",
"orders": [
{
"id": 210987092,
"address_id": 42171447,
"billing_address": {
"address1": "601 SW Washing St.",
"address2": "",
"city": "Portland",
"company": "Fake Company Billing",
"country_code": "US",
"first_name": "Fake Billing First",
"last_name": "Fake Billing Last",
"phone": "888-888-8888",
"province": "Oregon",
"zip": "97205"
},
"charge": {
"id": 272382456,
"external_transaction_id": {
"payment_processor": "ch_1HzWElJ2zqHvZRd1TWKFFqDR"
}
},
"client_details": {
"browser_ip": "192.168.0.1",
"user_agent": "safari webkit"
},
"created_at": "2020-12-17T18:50:25+00:00",
"currency": "usd",
"customer": {
"id": 37657002,
"email": "test@test.com",
"external_customer_id": {
"ecommerce": "382028302"
},
"hash": "7e706455cbd13e40"
},
"discounts": [
{
"id": 12345,
"code": "TESTCODE10",
"value": 10,
"value_type": "fixed_amount"
}
],
"error": null,
"external_cart_token": "aeic8101918fs0f8810",
"external_order_id": {
"ecommerce": "27117383938"
},
"external_order_number": {
"ecommerce": "1938"
},
"is_prepaid": false,
"line_items": [
{
"purchase_item_id": 63898947,
"external_inventory_policy": "decrement_obeying_policy",
"external_product_id": {
"ecommerce": "4381728735283"
},
"external_variant_id": {
"ecommerce": "999999999999"
},
"grams": 454,
"images": {
"large": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h_large.jpg",
"medium": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h_medium.jpg",
"original": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h.jpg",
"small": "https://example-cdn.com/s/files/1/0257/0351/4163/products/il_570xN.1723312095_a43h_small.jpg"
},
"original_price": "10.00",
"properties": [
{
"name": "Color",
"value": "Blue"
}
],
"purchase_item_type": "subscription",
"quantity": 1,
"sku": "TOM0001",
"tax_lines": [
{
"price": "0.993",
"rate": "0.0725",
"title": "CA State Tax",
"unit_price": "0.331"
},
{
"price": "0.308",
"rate": "0.0225",
"title": "LA County Tax",
"unit_price": "0.102"
}
],
"taxable": true,
"taxable_amount": "10.00",
"tax_due": "1.30",
"title": "Shirt bundle",
"total_price": "11.30",
"unit_price": "10.00",
"unit_price_includes_tax": false,
"variant_title": "Blue t-shirts"
}
],
"note": "next order in sequence 3",
"order_attributes": [
{
"name": "subscription_cycle_count_min",
"value": "3"
}
],
"processed_at": "2020-12-17T18:50:27+00:00",
"scheduled_at": "2020-12-17T18:50:27+00:00",
"shipping_address": {
"address1": "1030 Barnum Ave",
"address2": "Suite 101",
"city": "Stratford",
"company": "Fake Company",
"country_code": "US",
"first_name": "Fake First",
"last_name": "Fake Last",
"phone": "999-999-9999",
"province": "Connecticut",
"zip": "06614"
},
"shipping_lines": [
{
"code": "Standard",
"price": "4.90",
"taxable": true,
"tax_lines": [
{
"price": "0.355",
"rate": "0.0725",
"title": "CA State Tax"
},
{
"price": "0.110",
"rate": "0.0225",
"title": "Los Angeles County Tax"
}
],
"title": "Standard"
}
],
"status": "success",
"subtotal_price": "10.00",
"tags": "Subscription, Subscription Recurring Order",
"tax_lines": [
{
"price": "0.950",
"rate": "0.0725",
"title": "CA State Tax"
},
{
"price": "0.335",
"rate": "0.0225",
"title": "Los Angeles County Tax"
}
],
"taxable": true,
"total_discounts": "10.00",
"total_duties": "0.0",
"total_line_items_price": "10.00",
"total_price": "14.90",
"total_refunds": "0.00",
"total_tax": "10.00",
"total_weight_grams": 454,
"type": "recurring",
"updated_at": "2020-12-17T18:50:27+00:00"
}
]
}
Payment methods
The Payment Method
object holds payment and billing information. A Customer
may be associated with many Payment Methods
, and an Addresses
record must be associated with at least one Payment_method
.
Important - If you see the following error when accessing Payment Methods endpoints: “You do not have sufficient permissions (scopes) for this object”, the token you are using has not been configured with correct access permissions. Update the token to have “Read access” or “Read and Write access” depending on your use case.
The payment method object
The Payment Method object holds payment and billing information. A Customer
may be associated with many payment methods, and an Addresses
record must be associated with at least one Payment Method
.
Attributes
- idinteger
The unique payment method id for a customer.
- customer_idinteger
The Recharge
customer_id
. - created_atdatetime
The date and time when the payment method was created.
- defaultboolean
If this is the default
Payment_method
for theCustomer
.Customer
must have 1 and only 1 default paymentPayment_method
. - payment_detailsobject
Details about the specific payment method
Those details will vary based on the
payment_type
Show object attributes - payment_typestring
Possible values: CREDIT_CARD, PAYPAL, APPLE_PAY, GOOGLE_PAY, SEPA_DEBIT
The type of payment this is.
If passed, must also be accompanied by one of
stripe_customer_token
,paypal_customer_token
orauthorizedotnet_customer_token
inprocessor_payment_method_token
. - processor_customer_tokenstring
The customer token at the processor.
- processor_namestring
Possible values: stripe, braintree, authorize, shopify_payments, mollie
This will impact validation on billing_details.
Currently,
shopify_payments
is in read-only mode and can only be managed by Shopify. - processor_payment_method_tokenstring
The payment token at the processor.
- statusstring
Possible values: unvalidated, valid, invalid, empty
State of the
Payment Method
. - status_reasonstring
The status reason for the payment method.
Often used when
invalid
to provide background details in invalidity. - updated_atdatetime
Last time the
Payment_method
was updated.
Error related attributes
More Attributes
- billing_addressobject
An object with the customer’s address information.
Show object attributes
{
"payment_method": {
"id": 1234567,
"customer_id": 62459147,
"billing_address": {
"address1": "123 Washington Street",
"address2": "Suite 101",
"city": "Los Angeles",
"company": "Recharge",
"country": "United States",
"country_code": "US",
"first_name": "Jane",
"last_name": "Doe",
"phone": "1234567890",
"province": "California",
"zip": "90210"
},
"created_at": "2018-11-14T09:00:01",
"default": true,
"payment_details": {
"brand": "visa",
"exp_month": 12,
"exp_year": 2021,
"last4": 1234
},
"payment_type": "CREDIT_CARD",
"processor_customer_token": "cus_AB3ebcBaL6pCx9",
"processor_name": "stripe",
"processor_payment_method_token": "pm_1LdKdfj2zqHvUId1uGheBEHy",
"retry_date": null,
"status": null,
"status_reason": null,
"taxable": true,
"updated_at": "2018-11-14T09:00:01"
}
}
Create a payment method
Create a Payment Method in Recharge.
Body Parameters
- customer_idinteger* Required
The Recharge
customer_id
. - defaultboolean
If this is the default
Payment Method
for the customer, customer must have 1 and only 1 defaultPayment Method
. - payment_typestring* Required
Possible values: CREDIT_CARD, PAYPAL, APPLE_PAY, GOOGLE_PAY, SEPA_DEBIT
The type of payment.
- processor_customer_tokenstring* Required
The customer token at the processor.
On Stripe this begins with
cus_
. - processor_namestring* Required
Possible values: stripe, braintree, authorize, shopify_payments, mollie
This will impact validation on billing_details.
Currently,
shopify_payments
is in read-only mode and can only be managed by Shopify. - processor_payment_method_tokenstring
The payment token at the processor.
More Parameters
- billing_addressobject
An object with the customer’s address information.
Show object attributes - retry_chargesboolean
Whether charges associated with this payment method should be retried. If
true
then charges with previous payment-related errors will be set to retry during the next automated processing.
Responses
- 201
OK: The request was successful, created a new resource, and resource created is in the body.
Show response object
curl 'https://api.rechargeapps.com/payment_methods' \
-H 'X-Recharge-Version: 2021-11' \
-H 'Content-Type: application/json' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d '{
"customer_id": 62824147,
"default": true,
"payment_type": "CREDIT_CARD",
"processor_customer_token": "cus_JGYtLWi2HS8ymD",
"processor_name": "stripe",
"processor_payment_method_token": "pm_34jdshjs"
}'
{
"payment_method": {
"id": 1234567,
"customer_id": 62459147,
"billing_address": {
"address1": "123 Washington Street",
"address2": "Suite 101",
"city": "Los Angeles",
"company": "Recharge",
"country_code": "US",
"first_name": "Jane",
"last_name": "Doe",
"phone": "1234567890",
"province": "California",
"zip": "90210"
},
"created_at": "2018-11-14T09:00:01",
"default": true,
"payment_details": {
"brand": "visa",
"exp_month": 12,
"exp_year": 2021,
"last4": "1234"
},
"payment_type": "CREDIT_CARD",
"processor_customer_token": "cus_AB3457BaL90Cx9",
"processor_name": "stripe",
"processor_payment_method_token": "pm_1LdKdfj2zqHvUId1uGheBEHy",
"status": null,
"status_reason": null,
"updated_at": "2018-11-14T09:00:01"
}
}
Retrieve a payment method
Retrieve an existing Payment Method
using the Recharge Payment Method
id
.
Responses
- 200
successful response
Show response object
curl 'https://api.rechargeapps.com/payment_methods/1234567' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token'
{
"payment_method": {
"id": 1234567,
"customer_id": 62459147,
"billing_address": {
"address1": "123 Washington Street",
"address2": "Suite 101",
"city": "Los Angeles",
"company": "Recharge",
"country_code": "US",
"first_name": "Jane",
"last_name": "Doe",
"phone": "1234567890",
"province": "California",
"zip": "90210"
},
"created_at": "2018-11-14T09:00:01",
"default": true,
"payment_details": {
"brand": "visa",
"exp_month": 12,
"exp_year": 2021,
"last4": "1234"
},
"payment_type": "CREDIT_CARD",
"processor_customer_token": "cus_AB3457BaL90Cx9",
"processor_name": "stripe",
"processor_payment_method_token": "pm_1LdKdfj2zqHvUId1uGheBEHy",
"status": null,
"status_reason": null,
"updated_at": "2018-11-14T09:00:01"
}
}
Update a payment method
Modify an existing Payment Method
.
Body Parameters
- defaultboolean
If this is the default
Payment Method
for theCustomer
.Customer
must have 1 and only 1 default paymentPayment_method
. - processor_namestring
Possible values: stripe, braintree, authorize, shopify_payments, mollie
This will impact validation on billing_details.
Currently,
shopify_payments
is in read-only mode and can only be managed by Shopify. - billing_addressobject
An object with the customer’s address information.
Show object attributes
More Parameters
Responses
- 200
successful response
Show response object
curl -X PUT 'https://api.rechargeapps.com/payment_methods/1234567' \
-H 'X-Recharge-Version: 2021-11' \
-H 'Content-Type: application/json' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d '{"default": true}'
{
"payment_method": {
"id": 1234567,
"customer_id": 62459147,
"billing_address": {
"address1": "123 Washington Street",
"address2": "Suite 101",
"city": "Los Angeles",
"company": "Recharge",
"country_code": "US",
"first_name": "Jane",
"last_name": "Doe",
"phone": "1234567890",
"province": "California",
"zip": "90210"
},
"created_at": "2018-11-14T09:00:01",
"default": true,
"payment_details": {
"brand": "visa",
"exp_month": 12,
"exp_year": 2021,
"last4": "1234"
},
"payment_type": "CREDIT_CARD",
"processor_customer_token": "cus_AB3457BaL90Cx9",
"processor_name": "stripe",
"processor_payment_method_token": "pm_1LdKdfj2zqHvUId1uGheBEHy",
"status": null,
"status_reason": null,
"updated_at": "2018-11-14T09:00:01"
}
}
Delete a payment method
Permanently delete a Payment Method
.
Responses
- 204
Content Deleted: The server has successfully fulfilled the request and deleted the desired object and there is no content to send in the response body.
Show response object
curl --X DELETE 'https://api.rechargeapps.com/payment_methods/1234567' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token'
{}
List payment methods
Return a list of payment methods in your store.
HTTP request examples
GET /payment_methods
Query Parameters
- customer_idstring
Gets all
Payment methods
for this customer.
More Parameters
Responses
- 200
successful response
Show response object
curl 'https://api.rechargeapps.com/payment_methods' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d limit=3 -G
{
"payment_methods": [
{
"id": 1234567,
"billing_address": {
"address1": "123 Washington Street",
"address2": "Suite 101",
"city": "Los Angeles",
"company_name": "Acme Corp",
"country_code": "US",
"name": "Jane Doe",
"phone": "1234567890",
"province": "California",
"zip": "90210"
},
"created_at": "2018-11-14T09:00:01",
"customer_id": 999123999,
"default": true,
"payment_details": [
{
"brand": "visa",
"exp_month": 12,
"exp_year": 2021,
"last4": 1234
}
],
"payment_type": "CREDIT_CARD",
"processor_customer_token": "cus_AB3ebcBaL6pCx9",
"processor_name": "stripe",
"processor_payment_method_token": "pm_123123",
"updated_at": "2018-11-14T09:00:01"
}
]
}
Plans
The Plans
resource is used to create and configure the transactional options for the Product
(subscription, prepaid, and onetime purchase options) in Recharge.
The plan object
The Plan
object is used to create and configure the transactional options for the Products
in Recharge.
Current transactional options are: subscription, prepaid and onetime.
Important - Updating/modifying plans in 2021-11 will impact /products in 2021-01. These are not cross compatible. Proceed with caution.
Attributes
- idinteger
Unique numeric identifier for the
Plan
. - channel_settingsobject
An object containing the availability of the plan through various supported channels.
Show object attributes - created_atdatetime
The time the plan was created.
- deleted_atdatetime
If deleted, the time the plan was deleted.
- discount_amountstring
The discount amount applied to the product
price
when purchased through thisPlan
.All
Plans
for a product must have the same discount amount. - discount_typestring
Possible values: percentage
Used in combination with discount amount to determine the discount applied on a product price when purchased through this
Plan
. - external_plan_group_idstring
The id of the selling plan group as it appears in external platforms.
- external_plan_idstring
The id of the selling plan as it appears in external platforms.
- external_plan_namestring
The name of the selling plan as it appears in external platforms.
- external_product_idobject
An object containing the product id as it appears in external platforms.
Show object attributes - external_variant_idsarray
An array of variant ids as they appear in external platforms.
- has_variant_restrictionsboolean
Default: false
Whether this plan applies to specific variants of the product
- sort_orderinteger
The number indicating the order which the plan will be in a list of related plans.
- subscription_preferencesobject
An object containing the various subscription preferences associated with this plan.
Show object attributes - titlestring
The title of the plan.
All
Plans
for a product must have the sametitle
. - typestring
Possible values: subscription, prepaid, onetime
The type of the plan.
Products with a
prepaid
plan cannot have plans of other types. - updated_atdatetime
The time the plan was last updated.
Error related attributes
More Attributes
{
"plan": {
"id": 88237,
"channel_settings": {
"api": {
"display": true
},
"checkout_page": {
"display": true
},
"customer_portal": {
"display": true
},
"merchant_portal": {
"display": true
}
},
"created_at": "2021-07-27T19:07:34+00:00",
"deleted_at": null,
"discount_amount": "30.00",
"discount_type": "percentage",
"external_plan_group_id": "77695091010",
"external_plan_id": "690103779650",
"external_plan_name": "1 week subscription",
"external_product_id": {
"ecommerce": "2103271587891"
},
"external_variant_ids": [],
"has_variant_restrictions": false,
"sort_order": 1,
"subscription_preferences": {
"apply_cutoff_date_to_checkout": false,
"charge_interval_frequency": 30,
"cutoff_day_of_month": null,
"cutoff_day_of_week": null,
"expire_after_specific_number_of_charges": null,
"interval_unit": "day",
"order_day_of_month": 1,
"order_day_of_week": null,
"order_interval_frequency": 30
},
"title": "Jarred Pickles",
"type": "subscription",
"updated_at": "2021-07-27T19:07:34+00:00"
}
}
Create a plan
Create a Plan
for a Product
in Recharge.
Multiple Plans
may exist for any Product
, see the Plan
object for restrictions.
Body Parameters
- channel_settingsstring
An object containing the availability of the plan through various supported channels.
Show object attributes - discount_amountstring
The discount amount applied to the product price when purchased through this plan. All plans for a product must have the same discount amount.
All
Plans
for a product must have the same discount amount. - discount_typestring
Possible values: percentage
Used in combination with discount amount to determine the discount applied on a product price when purchased through this plan. (accepts: percentage)
- external_product_idobject* Required
An object containing the product id as it appears in external platforms.
Show object attributes - sort_orderinteger
The number indicating the order which the plan will be in a list of related plans.
- subscription_preferencesobject* Required
An object containing the various subscription preferences associated with this plan.
Show object attributes - titlestring* Required
The title of the plan.
All
Plans
for a product must have the sametitle
. - typestring* Required
Possible values: subscription, prepaid, onetime
The type of the plan. Products with a prepaid plan cannot have plans of other types.
More Parameters
Responses
- 200
successful response
Show response object
curl 'https://api.rechargeapps.com/plans' \
-H 'X-Recharge-Version: 2021-11' \
-H 'Content-Type: application/json' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d '{
"discount_amount": "10",
"discount_type": "percentage",
"external_product_id": {
"ecommerce": "2103271587891"
},
"sort_order": 1,
"subscription_preferences": {
"charge_interval_frequency": 30,
"expire_after_specific_number_of_charges": 6,
"interval_unit": "day",
"order_day_of_month": 1,
"order_interval_frequency": 30
}
"title": "Concert T-Shirt",
"type": "subscription",
}'
{
"plan": {
"id": 88237,
"channel_settings": {
"api": {
"display": true
},
"checkout_page": {
"display": true
},
"customer_portal": {
"display": true
},
"merchant_portal": {
"display": true
}
},
"created_at": "2021-07-27T19:07:34+00:00",
"deleted_at": null,
"discount_amount": "10",
"discount_type": "percentage",
"external_product_id": {
"ecommerce": "2103271587891"
},
"sort_order": 1,
"subscription_preferences": {
"charge_interval_frequency": 30,
"cutoff_day_of_month": null,
"cutoff_day_of_week": null,
"expire_after_specific_number_of_charges": 6,
"interval_unit": "day",
"order_day_of_month": 1,
"order_day_of_week": null,
"order_interval_frequency": 30
},
"title": "Concert TShirt",
"type": "subscription",
"updated_at": "2021-07-27T19:07:34+00:00"
}
}
Update a plan
Update an existing Plan.
Body Parameters
- channel_settingsobject
An object containing the availability of the plan through various supported channels.
Show object attributes - discount_amountstring
The discount amount applied to the product price when purchased through this plan. All plans for a product must have the same discount amount.
All
Plans
for a product must have the same discount amount. - discount_typestring
Possible values: percentage
Used in combination with discount amount to determine the discount applied on a product price when purchased through this plan.
- external_product_idobject
An object containing the product id as it appears in external platforms.
Show object attributes - sort_orderstring
The number indicating the order which the plan will be in a list of related plans.
- subscription_preferencesobject
An object containing the various subscription preferences associated with this plan.
Show object attributes - titlestring
The title of the plan.
More Parameters
Responses
- 200
successful response
Show response object
curl -x PUT 'https://api.rechargeapps.com/plans/16909886' \
-H 'X-Recharge-Version: 2021-11' \
-H 'Content-Type: application/json' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d '{"discount_amount": "12.0","title": "Concert T-Shirt"}'
{
"plan": {
"id": 88237,
"channel_settings": {
"api": {
"display": true
},
"checkout_page": {
"display": true
},
"customer_portal": {
"display": true
},
"merchant_portal": {
"display": true
}
},
"created_at": "2021-07-27T19:07:34+00:00",
"deleted_at": null,
"discount_amount": "10",
"discount_type": "percentage",
"external_product_id": {
"ecommerce": "2103271587891"
},
"sort_order": 1,
"subscription_preferences": {
"charge_interval_frequency": 30,
"cutoff_day_of_month": null,
"cutoff_day_of_week": null,
"expire_after_specific_number_of_charges": 6,
"interval_unit": "day",
"order_day_of_month": 1,
"order_day_of_week": null,
"order_interval_frequency": 30
},
"title": "Concert TShirt",
"type": "subscription",
"updated_at": "2021-07-27T19:07:34+00:00"
}
}
Delete a plan
Delete a plan.
Responses
- 204
Content Deleted: The server has successfully fulfilled the request and deleted the desired object and there is no content to send in the response body.
Show response object
curl -X DELETE 'https://api.rechargeapps.com/plans/321654' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token'
{}
List plans
Returns a list of all plans from Store
.
Query Parameters
- limitstring
Default: 50
Max: 250
The number of results.
- pagestring*Deprecated
Default: 1
The page to show.
Page-based pagination has been deprecated but still available to use for pages up to a 100. If you need data past this point, use cursor pagination.
- external_product_idstring
Return the
Plans
linked to theProduct
record in Recharge with the indicatedexternal_product_id
- idsstring
Filter plans by id. If passing multiple values, must be comma separated. Non-integer values will result in a 422 error
- updated_at_maxdatetime
Return the plans updated before the given date.
- updated_at_mindatetime
Return the plans updated after the given date.
- typestring
Possible values: subscription, prepaid, onetime
Return the plans that are of a specific type.
More Parameters
Responses
- 200
successful response
Show response object
curl 'https://api.rechargeapps.com/plans' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d limit=3 -G
{
"plans": [
{
"id": 88237,
"channel_settings": {
"api": {
"display": true
},
"checkout_page": {
"display": true
},
"customer_portal": {
"display": true
},
"merchant_portal": {
"display": true
}
},
"created_at": "2021-07-27T19:07:34+00:00",
"deleted_at": null,
"discount_amount": null,
"discount_type": null,
"external_product_id": {
"ecommerce": "2103271587891"
},
"sort_order": 1,
"subscription_preferences": {
"charge_interval_frequency": 30,
"cutoff_day_of_month": null,
"cutoff_day_of_week": null,
"expire_after_specific_number_of_charges": null,
"interval_unit": "day",
"order_day_of_month": 1,
"order_day_of_week": null,
"order_interval_frequency": 30
},
"title": "Jarred Pickles",
"type": "subscription",
"updated_at": "2021-07-27T19:07:34+00:00"
}
]
}
Bulk create plans
Bulk create new plans.
There is a limit of 20 plans per request.
Body Parameters
- plansarray* Required
An array of plans to be created.
Show object attributes
More Parameters
Responses
- 200
successful response
Show response object
curl 'https://api.rechargeapps.com/products/2103271587891/plans-bulk' \
-H 'X-Recharge-Version: 2021-11' \
-H 'Content-Type: application/json' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d '{
"plans": [
{
"discount_amount": "10",
"discount_type": "percentage",
"sort_order": 1,
"title": "Concert T-Shirt",
"type": "subscription",
"subscription_preferences": {
"charge_interval_frequency": 30,
"expire_after_specific_number_of_charges": 6,
"interval_unit": "day",
"order_day_of_month": 1,
"order_interval_frequency": 30
}
},
{
"discount_amount": "10",
"discount_type": "percentage",
"sort_order": 2,
"title": "Concert T-Shirt",
"type": "subscription",
"subscription_preferences": {
"charge_interval_frequency": 60,
"expire_after_specific_number_of_charges": 6,
"interval_unit": "day",
"order_day_of_month": 1,
"order_interval_frequency": 60
}
}
]
}'
{
"plans": [
{
"id": 88237,
"channel_settings": {
"api": {
"display": true
},
"checkout_page": {
"display": true
},
"customer_portal": {
"display": true
},
"merchant_portal": {
"display": true
}
},
"created_at": "2021-07-27T19:07:34+00:00",
"discount_amount": "10",
"discount_type": "percentage",
"external_product_id": {
"ecommerce": "2103271587891"
},
"sort_order": 1,
"title": "Concert T-Shirt",
"type": "subscription",
"subscription_preferences": {
"charge_interval_frequency": 30,
"cutoff_day_of_month": null,
"cutoff_day_of_week": null,
"expire_after_specific_number_of_charges": 6,
"interval_unit": "day",
"order_day_of_month": 1,
"order_interval_frequency": 30
},
"updated_at": "2021-07-27T19:07:34+00:00"
},
{
"id": 88238,
"channel_settings": {
"api": {
"display": true
},
"checkout_page": {
"display": true
},
"customer_portal": {
"display": true
},
"merchant_portal": {
"display": true
}
},
"created_at": "2021-07-27T19:07:34+00:00",
"discount_amount": "10",
"discount_type": "percentage",
"external_product_id": {
"ecommerce": "2103271587891"
},
"sort_order": 2,
"title": "Concert T-Shirt",
"type": "subscription",
"subscription_preferences": {
"charge_interval_frequency": 60,
"expire_after_specific_number_of_charges": 6,
"interval_unit": "day",
"order_day_of_month": 1,
"order_interval_frequency": 60
},
"updated_at": "2021-07-27T19:07:34+00:00"
}
]
}
Bulk update plans
Bulk update existing plans.
There is a limit of 20 plans per request.
Body Parameters
- plansarray* Required
An array of plans to be updated. Must provide ID for each plan.
Show object attributes
More Parameters
Responses
- 200
successful response
Show response object
curl -X PUT 'https://api.rechargeapps.com/products/2103271587891/plans-bulk' \
-H 'X-Recharge-Version: 2021-11' \
-H 'Content-Type: application/json' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d '{
"plans": [
{
"id": 88237,
"title": "Updated T-Shirt"
},
{
"id": 88238,
"title": "Updated T-Shirt"
}
]
}'
{
"plans": [
{
"id": 88237,
"channel_settings": {
"api": {
"display": true
},
"checkout_page": {
"display": true
},
"customer_portal": {
"display": true
},
"merchant_portal": {
"display": true
}
},
"created_at": "2021-07-27T19:07:34+00:00",
"discount_amount": "10",
"discount_type": "percentage",
"external_product_id": {
"ecommerce": "2103271587891"
},
"sort_order": 1,
"title": "Concert T-Shirt",
"type": "subscription",
"subscription_preferences": {
"charge_interval_frequency": 30,
"cutoff_day_of_month": null,
"cutoff_day_of_week": null,
"expire_after_specific_number_of_charges": 6,
"interval_unit": "day",
"order_day_of_month": 1,
"order_interval_frequency": 30
},
"updated_at": "2021-07-27T19:07:34+00:00"
},
{
"id": 88238,
"channel_settings": {
"api": {
"display": true
},
"checkout_page": {
"display": true
},
"customer_portal": {
"display": true
},
"merchant_portal": {
"display": true
}
},
"created_at": "2021-07-27T19:07:34+00:00",
"discount_amount": "10",
"discount_type": "percentage",
"external_product_id": {
"ecommerce": "2103271587891"
},
"sort_order": 2,
"title": "Concert T-Shirt",
"type": "subscription",
"subscription_preferences": {
"charge_interval_frequency": 60,
"expire_after_specific_number_of_charges": 6,
"interval_unit": "day",
"order_day_of_month": 1,
"order_interval_frequency": 60
},
"updated_at": "2021-07-27T19:07:34+00:00"
}
]
}
Bulk delete plans
Bulk delete existing plans.
There is a limit of 20 plans per request.
Body Parameters
- plansarray* Required
An array of plans to be deleted. Must provide ID for each plan.
Show object attributes
More Parameters
Responses
- 204
Content Deleted: The server has successfully fulfilled the request and deleted the desired object and there is no content to send in the response body.
Show response object
curl -X DELETE 'https://api.rechargeapps.com/products/2103271587891/plans-bulk' \
-H 'X-Recharge-Version: 2021-11' \
-H 'Content-Type: application/json' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d '{
"plans": [
{
"id": 88237
},
{
"id": 88238
}
]
}'
{}
Products
Representation of the Products from your catalog in Recharge.
The product object
Attributes
- external_product_idstring
Unique numeric identifier of the product record in Recharge. The primary id of the record.
- brandstring
The brand name of the product.
- external_created_atdatetime
The date and time at which the product was created in an external product catalog.
- external_updated_atdatetime
The date and time at which the product was updated in an external product catalog.
- imagesarray
An array of urls for various sizes of image files.
Show object attributes - options
An array of options for the product record, such as size, color, etc.
Show object attributes - published_atdatetime
The datetime at which the product became available for purchase. To make the product available, this should be set in the past.
- requires_shippingboolean
Indicator of shipping requirement.
Dictates the default for the variants. Can be overriden at the variant level.
- titlestring
The title of the product.
- variantsarray
An array of variants of the product.
If your PIM does not use variants, create a single variant product.
Show object attributes - vendorstring
The vendor of the product.
Error related attributes
More Attributes
{
"brand": "Recharge",
"description": "A cotton shirt with the Recharge logo",
"external_created_at": "2021-01-01T00:00:00+00:00",
"external_product_id": "5",
"external_updated_at": "2021-01-01T00:00:00+00:00",
"images": [
{
"small": "https:...",
"medium": "https:...",
"large": "https:...",
"original": "https:...",
"sort_order": 2
}
],
"options": [
{
"name": "Size",
"position": 0,
"values": [
{
"label": "small",
"position": 0
},
{
"label": "medium",
"position": 1
},
{
"label": "large",
"position": 2
}
]
}
],
"published_at": "2021-01-01:00:00+00:00",
"requires_shipping": true,
"title": "Recharge Logo Shirt",
"variants": [
{
"external_variant_id": " 9",
"dimensions": {
"weight": 1234.5
},
"image": {
"small": "https:....jpeg",
"medium": "https:....jpeg",
"large": "https:....jpeg",
"original": "https:....jpeg"
},
"option_values": [
{
"label": "small"
}
],
"requires_shipping": true,
"sku": "CLO123",
"title": "Recharge Logo Shirt - Small",
"taxable": true,
"tax_code": "CLOTHING",
"prices": {
"compare_at_price": "14.22",
"unit_price": "12.22"
}
}
],
"vendor": "Recharge Apparel Store"
}
Create a product
Create a new product in your Recharge Product Catalog. After adding the record, subscription preferences may be added using the Products page of Recharge Admin Portal or you can use the Plans
resource currently in Alpha
.
Body Parameters
- external_product_idobject* Required
Unique numeric identifier of the product record in Recharge. The primary id of the record.
Show object attributes - brandstring
The brand name of the product.
- external_created_atdatetime
The date and time at which the product was created in an external product catalog.
- external_updated_atdatetime
The date and time at which the product was updated in an external product catalog.
- imagesarray
An array of urls for various sizes of image files.
small
is the default picture shown on the checkout. Ifsmall
is not present we renderoriginal
. Please note for better rendering we advise you populatesmall
.Show object attributes - options* Required
An array of options for the product record, such as size, color, etc.
Show object attributes - published_atdatetime
The date and time at which the product became available for purchase. To make the product available, this should be set in the past.
- requires_shippingstring
Indicator of shipping requirement. If
true
the product associated to the variant needs to be shipped.This is usually false for digital goods.
- titlestring* Required
The title of the product.
- variantsarray* Required
An array of variants of the product.
If your PIM does not use variants, create a single variant product.
Show object attributes - vendorstring* Required
The vendor of the product.
More Parameters
Responses
- 202
successful response
Show response object
curl 'https://api.rechargeapps.com/products' \
-H 'X-Recharge-Version: 2021-11'
-H 'Content-Type: application/json' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d '{
"external_product_id": "10",
"brand": "Recharge",
"description": "A cotton shirt with the Recharge logo",
"images": [
{
"small": "https:...",
"medium": "https:...",
"large": "https:...",
"original": "https:...",
"sort_order": 2
}
],
"options": [
{
"name": "Size",
"position": 0,
"values": [
{"label": "small", "position": 0},
{"label": "medium", "position": 1},
{"label": "large", "position": 2}
]
}
],
"published_at": "2021-01-01:00:00+00:00",
"requires_shipping": true,
"title": "Recharge Logo Shirt",
"variants": [
{
"external_variant_id": "9",
"dimensions": {
"weight": 1234.5
},
"image": {
"small": "https:...",
"medium": "https:...",
"large": "https:...",
"original": "https:..."
},
"option_values": [
{
"label": "small"
}
],
"sku": "CLO123",
"title": "Recharge Logo Shirt - Small",
"taxable": true,
"tax_code": "CLOTHING",
"prices": {
"compare_at_price": "14.22",
"unit_price": "12.22"
}
}
],
"vendor": "Recharge Apparel Store"
}'
{
"product": {
"brand": "Recharge",
"description": "A cotton shirt with the Recharge logo",
"external_created_at": "2021-01-01T00:00:00+00:00",
"external_product_id": "10",
"external_updated_at": "2021-01-01T00:00:00+00:00",
"images": [
{
"large": "https:...",
"medium": "https:...",
"original": "https:...",
"small": "https:...",
"sort_order": 2
}
],
"options": [
{
"name": "Size",
"position": 0,
"values": [
{
"label": "small",
"position": 0
},
{
"label": "medium",
"position": 1
},
{
"label": "large",
"position": 2
}
]
}
],
"published_at": "2021-01-01T00:00:00+00:00",
"requires_shipping": true,
"title": "Recharge Logo Shirt",
"variants": [
{
"dimensions": {
"weight": 1234.5,
"weight_unit": "lb"
},
"external_variant_id": "9",
"image": {
"large": "https:...",
"medium": "https:...",
"original": "https:...",
"small": "https:..."
},
"option_values": [
{
"label": "small"
}
],
"prices": {
"compare_at_price": "14.22",
"unit_price": "12.22"
},
"sku": "CLO123",
"tax_code": "CLOTHING",
"taxable": true,
"title": "Recharge Logo Shirt - Small",
"requires_shipping": true
}
],
"vendor": "Recharge Apparel Store"
}
}
Retrieve a product
Retrieves a Product
from store’s product catalog using the external_product_id
as lookup id.
Responses
- 200
successful response
Show response object
curl 'https://api.rechargeapps.com/products/10' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token'
{
"product": {
"brand": "Recharge",
"description": "A cotton shirt with the Recharge logo",
"external_created_at": "2021-01-01T00:00:00+00:00",
"external_product_id": "10",
"external_updated_at": "2021-01-01T00:00:00+00:00",
"images": [
{
"large": "https:...",
"medium": "https:...",
"original": "https:...",
"small": "https:...",
"sort_order": 2
}
],
"options": [
{
"name": "Size",
"position": 0,
"values": [
{
"label": "small",
"position": 0
},
{
"label": "medium",
"position": 1
},
{
"label": "large",
"position": 2
}
]
}
],
"published_at": "2021-01-01T00:00:00+00:00",
"requires_shipping": true,
"title": "Recharge Logo Shirt",
"variants": [
{
"dimensions": {
"weight": 1234.5,
"weight_unit": "lb"
},
"external_variant_id": "9",
"image": {
"large": "https:...",
"medium": "https:...",
"original": "https:...",
"small": "https:..."
},
"option_values": [
{
"label": "small"
}
],
"prices": {
"compare_at_price": "14.22",
"unit_price": "12.22"
},
"sku": "CLO123",
"tax_code": "CLOTHING",
"taxable": true,
"title": "Recharge Logo Shirt - Small",
"requires_shipping": true
}
],
"vendor": "Recharge Apparel Store"
}
}
Update a product
Update an existing product.
Body Parameters
- brandstring
The brand name of the product.
- external_created_atdatetime
The date and time at which the product was created in an external product catalog.
- external_updated_atdatetime
The date and time at which the product was updated in an external product catalog.
- imagesarray
An array of urls for various sizes of image files.
Show object attributes - options
An array of options for the product record, such as size, color, etc.
Show object attributes - published_atdatetime
The datetime at which the product became available for purchase. To make the product available, this should be set in the past.
- requires_shippingboolean
Indicator of shipping requirement. If
true
the product associated to the variant needs to be shipped.This is usually
false
for digital goods. - titlestring
The title of the product.
- variantsarray
An array of variants of the product.
If your PIM does not use variants, create a single variant product.
Show object attributes - vendorstring
The vendor of the product.
More Parameters
Responses
- 200
successful response
Show response object
curl -X PUT 'https://api.rechargeapps.com/products/1327844' \
-H 'X-Recharge-Version: 2021-11' \
-H 'Content-Type: application/json' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d '{
"brand": "Recharge",
"variants": [
{
"external_variant_id": "101",
"dimensions": {
"weight": 1234.5
},
"image": {
"small": "https:...",
"medium": "https:...",
"large": "https:...",
"original": "https:..."
},
"option_values": [{
"label": "small"
}],
"sku": "CLO123",
"title": "Small Recharge Logo Shirt",
"taxable": true,
"tax_code": "CLOTHING",
"prices": {
"compare_at_price": "14.22",
"unit_price": "12.22"
}
},
{
"external_variant_id": "102",
"dimensions": {
"weight": 1234.5
},
"image": {
"small": "https:...",
"medium": "https:...",
"large": "https:...",
"original": "https:..."
},
"option_values": [{
"label": "medium"
}],
"sku": "CLO124",
"title": "Medium Recharge Logo Shirt",
"taxable": true,
"tax_code": "CLOTHING",
"prices": {
"compare_at_price": "14.22",
"unit_price": "12.22"
}
}
]
}'
{
"product": {
"brand": "Recharge",
"description": "A cotton shirt with the Recharge logo",
"external_created_at": "2021-01-01T00:00:00+00:00",
"external_product_id": "10",
"external_updated_at": "2021-01-01T00:00:00+00:00",
"images": [
{
"large": "https:...",
"medium": "https:...",
"original": "https:...",
"small": "https:...",
"sort_order": 2
}
],
"options": [
{
"name": "Size",
"position": 0,
"values": [
{
"label": "small",
"position": 0
},
{
"label": "medium",
"position": 1
},
{
"label": "large",
"position": 2
}
]
}
],
"published_at": "2021-01-01T00:00:00+00:00",
"requires_shipping": true,
"title": "Recharge Logo Shirt",
"variants": [
{
"dimensions": {
"weight": 1234.5,
"weight_unit": "lb"
},
"external_variant_id": "9",
"image": {
"large": "https:...",
"medium": "https:...",
"original": "https:...",
"small": "https:..."
},
"option_values": [
{
"label": "small"
}
],
"prices": {
"compare_at_price": "14.22",
"unit_price": "12.22"
},
"sku": "CLO123",
"tax_code": "CLOTHING",
"taxable": true,
"title": "Recharge Logo Shirt - Small",
"requires_shipping": true
}
],
"vendor": "Recharge Apparel Store"
}
}
Delete a product
Delete product from Store
.
Responses
- 204
successful response
Show response object
curl -X DELETE 'https://api.rechargeapps.com/products/509780' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token'
{}
List products
List products from store’s product catalog.
Query Parameters
- external_product_idsstring
Filter products by external product ids.
GET /products/?external_product_ids=123,223
will return all products with the associatedexternal_product_ids
.
More Parameters
Responses
- 200
successful response
Show response object
curl 'https://api.rechargeapps.com/products' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token'
{
"next_cursor": null,
"previous_cursor": null,
"products": [
{
"brand": "Recharge",
"description": "A cotton shirt with the Recharge logo",
"external_created_at": null,
"external_product_id": "10",
"external_updated_at": "2021-07-21T17:25:46+00:00",
"images": [
{
"large": "https:...",
"medium": "https:...",
"original": "https:...",
"small": "https:...",
"sort_order": 2
}
],
"options": [
{
"name": "Size",
"position": 0,
"values": [
{
"label": "small",
"position": 0
},
{
"label": "medium",
"position": 1
},
{
"label": "large",
"position": 2
}
]
}
],
"published_at": "2021-01-01T00:00:00+00:00",
"requires_shipping": true,
"title": "Recharge Logo Shirt",
"variants": [
{
"dimensions": {
"weight": 1234.5,
"weight_unit": "lb"
},
"external_variant_id": "101",
"image": {
"large": "https:...",
"medium": "https:...",
"original": "https:...",
"small": "https:..."
},
"option_values": [
{
"label": "small"
}
],
"prices": {
"compare_at_price": "14.22",
"unit_price": "12.22"
},
"sku": "CLO123",
"tax_code": "CLOTHING",
"taxable": true,
"title": "Small Recharge Logo Shirt",
"requires_shipping": true
},
{
"dimensions": {
"weight": 1234.5,
"weight_unit": "lb"
},
"external_variant_id": "102",
"image": {
"large": "https:...",
"medium": "https:...",
"original": "https:...",
"small": "https:..."
},
"option_values": [
{
"label": "medium"
}
],
"prices": {
"compare_at_price": "14.22",
"unit_price": "12.22"
},
"sku": "CLO124",
"tax_code": "CLOTHING",
"taxable": true,
"title": "Medium Recharge Logo Shirt",
"requires_shipping": true
}
],
"vendor": "Recharge Apparel Store"
}
]
}
Retention Strategies
Retention Strategies are customer retention workflows that are used to win back customers who are in the process of canceling their subscription. The workflow is determined by the cancellation reason selected by the customer.
The retention strategy object
The RetentionStrategy
object holds a cancellation reason and an optional customer retention workflow. Possible retention workflows include delaying a shipment, skipping a shipment, swapping a product, and offering a discount.
Attributes
- idinteger
Unique numeric identifier for the
RetentionStrategy
. - cancellation_flow_typestring
Possible values: subscription, membership
The type of
PurchaseItem
that the retention strategy applies to. The default value is “subscription”. - created_atdatetime
The date and time the
RetentionStrategy
was created. - discount_codestring
Discount code to offer customer when used with an
incentive_type
ofdiscount
. - incentive_typestring
Possible values: delay_subscription, discount, skip_charge, swap_product
Type of incentive to offer customer.
- prevention_textstring
A short phrase or question to ask for more details or to provide an alternative to canceling.
- reasonstring
The reason for canceling.
- updated_atdatetime
The date and time the
RetentionStrategy
was last updated.
Error related attributes
More Attributes
{
"retention_strategy": {
"id": 12345,
"cancellation_flow_type": "subscription",
"created_at": "2021-07-31T20:45:00+00:00",
"discount_code": null,
"incentive_type": "swap_product",
"prevention_text": "Would you like to swap the product out for another item?",
"reason": "I want a different product or variety",
"updated_at": "2021-07-31T20:45:00+00:00"
}
}
Create a retention strategy
Create a retention strategy
Body Parameters
- cancellation_flow_typestring
Possible values: subscription, membership
The type of
PurchaseItem
that the retention strategy applies to. The default value is “subscription”. - incentive_typestring
Possible values: delay_subscription, discount, skip_charge, swap_product
Type of incentive to offer customer.
- discount_codestring
Discount code to offer customer when used with an
incentive_type
ofdiscount
.This field is required when
incentive_type
is set todiscount
. - prevention_textstring* Required
A short phrase or question to ask for more details or to provide an alternative to canceling.
- reasonstring* Required
The reason for canceling.
More Parameters
Responses
- 201
successful response
Show response object
curl -X POST -i 'https://api.rechargeapps.com/retention_strategies' \
-H 'X-Recharge-Access-Token: your_api_token' \
-H 'X-Recharge-Version: 2021-11' \
-H "Accept: application/json" \
-H "Content-Type: application/json" \
-d '{
"incentive_type": "swap_product",
"prevention_text": "Would you like to swap the product out for another item?",
"reason": "I want a different product or variety"
}'
{
"retention_strategy": {
"id": 12345,
"cancellation_flow_type": "subscription",
"incentive_type": "swap_product",
"discount_code": null,
"prevention_text": "Would you like to swap the product out for another item?",
"reason": "I want a different product or variety",
"created_at": "2021-07-31T20:45:00+00:00",
"updated_at": "2021-10-30T07:14:21+00:00"
}
}
Retrieve a retention strategy
Retrieve a retention strategy using the Recharge retention_strategy_id
.
Responses
- 200
successful response
Show response object
curl 'https://api.rechargeapps.com/retention_strategies/12345' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token'
{
"retention_strategy": {
"id": 12345,
"cancellation_flow_type": "subscription",
"incentive_type": "swap_product",
"discount_code": null,
"prevention_text": "Would you like to swap the product out for another item?",
"reason": "I want a different product or variety",
"created_at": "2021-07-31T20:45:00+00:00",
"updated_at": "2021-07-31T20:45:00+00:00"
}
}
Update a retention strategy
Update an existing retention strategy.
Body Parameters
- cancellation_flow_typestring
Possible values: subscription, membership
The type of
PurchaseItem
that the retention strategy applies to. The default value is “subscription”. - incentive_typestring
Possible values: delay_subscription, discount, skip_charge, swap_product
Type of incentive to offer customer.
- discount_codestring
Discount code to offer customer when used with an
incentive_type
ofdiscount
.This field is required when
incentive_type
is set todiscount
. - prevention_textstring
A short phrase or question to ask for more details or to provide an alternative to canceling.
- reasonstring
The reason for canceling.
More Parameters
Responses
- 200
successful response
Show response object
curl -X PUT 'https://api.rechargeapps.com/retention_strategies/12345' \
-H 'X-Recharge-Version: 2021-11' \
-H 'Content-Type: application/json' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d '{"reason": "I want a different product or variety"}'
{
"retention_strategy": {
"id": 12345,
"cancellation_flow_type": "subscription",
"incentive_type": "swap_product",
"discount_code": null,
"prevention_text": "Would you like to swap the product out for another item?",
"reason": "I want a different product or variety",
"created_at": "2021-07-31T20:45:00+00:00",
"updated_at": "2021-07-31T20:45:00+00:00"
}
}
Delete a retention strategy
Delete a retention strategy.
Responses
- 204
successful response
Show response object
curl -X DELETE 'https://api.rechargeapps.com/retention_strategies/12345' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token'
{}
List retention strategies
Retrieve all retention strategies
Responses
- 200
successful response
Show response object
curl -X GET -i 'https://api.rechargeapps.com/retention_strategies' \
-H 'X-Recharge-Access-Token: your_api_token' \
-H 'X-Recharge-Version: 2021-11' \
-H "Accept: application/json" \
-H "Content-Type: application/json"
{
"retention_strategies": [
{
"id": 12347,
"cancellation_flow_type": "subscription",
"incentive_type": null,
"discount_code": null,
"prevention_text": "Would you care to provide any additional details?",
"reason": "This was created by accident",
"created_at": "2021-11-09T16:00:00+00:00",
"updated_at": "2021-11-09T16:00:00+00:00"
},
{
"id": 12346,
"cancellation_flow_type": "subscription",
"incentive_type": "skip_charge",
"discount_code": null,
"prevention_text": "If you have more than you need, we can skip your upcoming order.",
"reason": "I already have more than I need",
"created_at": "2021-10-30T07:14:21+00:00",
"updated_at": "2021-10-30T07:14:21+00:00"
},
{
"id": 12345,
"cancellation_flow_type": "subscription",
"incentive_type": "swap_product",
"discount_code": null,
"prevention_text": "Would you like to swap the product out for another item?",
"reason": "I want a different product or variety",
"created_at": "2021-07-31T20:45:00+00:00",
"updated_at": "2021-07-31T20:45:00+00:00"
}
]
}
Store
The store endpoint includes ReCharge settings and other store specific information.
The store object
The store endpoint includes Recharge settings and other store specific information.
Attributes
- idinteger
Unique number identifier of the store.
- checkout_logo_urlstring
Checkout logo url.
- checkout_platformstring
Checkout platform.
- created_atdatetime
Date and time when the store is created.
- customer_portal_base_urlstring
Customer Portal Base URL.
- default_api_versioninteger
Default API version.
- emailstring
E-mail address of the store owner.
- enabled_presentment_currenciesarray
Enabled currencies the store sells in.
- enabled_presentment_currencies_symbolsarray
Currency symbol used for enabled currencies the store sells in.
Show object attributes - disabled_currencies_historicalarray
A ledger of currencies that the store no longer sells in. If a currency is present in both the enabled_presentment_currencies and disabled_currencies_historical ledger it is because it was disabled at one point in time but is now enabled.
- external_platformstring
External platform connected to Recharge.
Will be one of
shopify
,big_commerce
,headless
, ormagento
- identifierstring
Unique identifier of the store on the external platform.
- merchant_portal_base_urlstring
Merchant portal base URL.
- namestring
Name of the store.
- phonestring
Phone number of the store owner.
- timezoneobject
Timezone of the store.
Show object attributes - updated_atdatetime
The date and time when the address was last updated.
- weight_unitstring
Weight unit for the store.
Error related attributes
More Attributes
{
"store": {
"id": 89559201,
"checkout_logo_url": null,
"checkout_platform": "recharge",
"created_at": "2020-07-15T00:00:00+00:00",
"currency": "USD",
"customer_portal_base_url": null,
"default_api_version": "2021-01",
"email": "email@email.com",
"external_platform": "shopify",
"identifier": "store-id",
"merchant_portal_base_url": null,
"name": "store-name",
"phone": "5555555555",
"enabled_presentment_currencies": [
"USD"
],
"enabled_presentment_currencies_symbols": [
{
"currency": "USD",
"location": "before",
"suffix": "",
"symbol": "$"
}
],
"disabled_currencies_historical": [],
"timezone": {
"iana_name": "America/New_York",
"name": "(GMT-05:00) Eastern Time (US & Canada)"
},
"updated_at": "2020-07-15T00:00:00+00:00",
"weight_unit": "g"
}
}
Retrieve a store
Retrieve store details.
Responses
- 200
successful response
Show response object
curl 'https://api.rechargeapps.com/store' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token'
{
"store": {
"id": 4797,
"checkout_logo_url": null,
"checkout_platform": "recharge",
"created_at": "2020-04-22T00:20:52+00:00",
"currency": "USD",
"customer_portal_base_url": null,
"default_api_version": "2021-01",
"email": "email@email.com",
"enabled_presentment_currencies": [
"USD"
],
"enabled_presentment_currencies_symbols": [
{
"currency": "USD",
"location": "before",
"suffix": "",
"symbol": "$"
}
],
"disabled_currencies_historical": [],
"external_platform": "custom",
"identifier": "store-id",
"merchant_portal_base_url": null,
"name": "store-name",
"province": "Connecticut",
"phone": "5555555555",
"timezone": {
"iana_name": "America/New_York",
"name": "(GMT-05:00) Eastern Time (US & Canada)"
},
"updated_at": "2020-04-25T00:20:52+00:00",
"weight_unit": "g"
}
}
Subscriptions
Subscriptions are individual items a customer receives on a recurring basis.
A Subscription
is a Product
added to an Address
.
The subscription object
Subscriptions are individual items a customer benefit from on a recurring basis.
These fields are deprecated, however they will not be removed from this API version.
product_title
is deprecated. Use title
instead.
shipping_date
is deprecated. Use scheduled_at
instead.
shopify_id
is deprecated. Use shopify_order_id
instead.
address_is_active
is deprecated. Please ignore, not an applicable field.
Attributes
- idinteger
Unique numeric identifier for the subscription.
- address_idinteger
Unique numeric identifier for the address the subscription is associated with.
- customer_idinteger
Unique numeric identifier for the customer the subscription is tied to.
- analytics_dataobject
An object used to contain analytics data such as utm parameters.
Show object attributes - cancellation_reasonstring
Reason provided for cancellation.
- cancellation_reason_commentsstring
Additional comment for cancellation. Maximum length is 1024 characters.
- cancelled_atdatetime
The time the subscription was cancelled.
- charge_interval_frequencystring
Max: 1000
The number of units (specified in
order_interval_unit
) between eachCharge
. For example,order_interval_unit=month
andcharge_interval_frequency=3
, indicate charge every 3 months.Charges
must use the same unit types as orders. - created_atdatetime
The time the subscription was created.
- expire_after_specific_number_of_chargesinteger
Set the number of charges until subscription expires.
- external_product_idobject
An object containing the product id as it appears in external platforms.
Show object attributes - external_variant_idobject
An object containing the variant id as it appears in external platforms.
Show object attributes - has_queued_chargesboolean
Retrieves
true
if there is queued charge. Otherwise, retrievesfalse
. - is_prepaidboolean
Value is set to
true
if it is a prepaid item. - is_skippableboolean
Value is set to
true
if it is not a prepaid item - is_swappableboolean
Value is set to
true
if it is not a prepaid item and if in Customer portal settings swap is allowed for customers. - max_retries_reachedboolean
Retrieves
true
if charge has an error max retries reached. Otherwise, retrievesfalse
. - next_charge_scheduled_atdatetime
Date of the next charge for the subscription.
- order_day_of_monthinteger
The set day of the month order is created. Default is that there isn’t a strict day of the month when the order is created.
This is only applicable to subscriptions with
order_interval_unit:“month”
. - order_day_of_weekinteger
The set day of the week order is created. Default is that there isn’t a strict day of the week order is created.
This is only applicable to subscriptions with
order_interval_unit = “week”
.
Value of 0 equals to Monday, 1 to Tuesday etc. - order_interval_frequencyinteger
Max: 1000
The number of units (specified in
order_interval_unit
) between each order. For example, order_interval_unit=month and order_interval_frequency=3, indicate order every 3 months. Max value: 1000 - order_interval_unitstring
Possible values: day, week, month
The frequency unit used to determine when a subscription’s order is created.
- presentment_currencystring
The presentment currency of the subscription.
- pricestring
The price of the item before discounts, taxes, or shipping have been applied.
- product_titlestring
The name of the product in a store’s catalog.
- propertiesarray
A list of line item objects, each one containing information about the subscription. Custom key-value pairs can be installed here, they will appear on the connected queued charge and after it is processed on the order itself.
Show object attributes - quantityinteger
The number of items in the subscription.
- skustring
A unique identifier of the item in the fulfillment. In cases where SKU is blank, it will be dynamically pulled whenever it is used.
- sku_overrideboolean
Flag that is automatically updated to
true
when SKU is passed on create or update. Whensku_override
istrue
, the SKU on the subscription will be used to generate charges and orders. Whensku_override
isfalse
, Recharge will dynamically fetch the SKU from the corresponding external platform variant. - statusstring
Possible values: active, cancelled, expired
The status of the subscription.
EXPIRED
- This status occurs when the maximum number of charges for a product has been reached. - updated_atdatetime
The date time at which the purchase_item record was last updated.
- variant_titlestring
The name of the variant in a shop’s catalog.
Error related attributes
More Attributes
{
"subscription": {
"id": 89559201,
"address_id": 48563471,
"customer_id": 43845860,
"analytics_data": {
"utm_params": []
},
"cancellation_reason": null,
"cancellation_reason_comments": null,
"cancelled_at": null,
"charge_interval_frequency": "30",
"created_at": "2021-02-25T21:27:19+00:00",
"expire_after_specific_number_of_charges": null,
"external_product_id": {
"ecommerce": "2103271587891"
},
"external_variant_id": {
"ecommerce": "18261278883891"
},
"has_queued_charges": true,
"is_prepaid": true,
"is_skippable": false,
"is_swappable": false,
"max_retries_reached": false,
"next_charge_scheduled_at": "2020-07-15",
"order_day_of_month": null,
"order_day_of_week": null,
"order_interval_frequency": 15,
"order_interval_unit": "day",
"presentment_currency": "USD",
"price": 5,
"product_title": "Powder Milk 50.00% Off Auto renew",
"properties": [
{
"name": "Colour",
"value": "White"
},
{
"name": "Package Material",
"value": "Paper"
}
],
"quantity": 3,
"sku": null,
"sku_override": false,
"status": "active",
"updated_at": "2020-07-10T10:30:51+00:00",
"variant_title": "1 / Powder"
}
}
Create a subscription
When creating a subscription via API, order_interval_frequency
and charge_interval_frequency
values do not necessarily need to match the values set in the respective Plans
. The product, however, does need to have at least one Plan
in order to be added to a subscription.
Body Parameters
- address_idinteger* Required
Unique numeric identifier of the customer’s address.
- charge_interval_frequencyinteger* Required
The number of units, specified in
order_interval_unit
, between each charge. - expire_after_specific_number_of_chargesinteger
The number of charges until the subscription expires.
- next_charge_scheduled_atdatetime* Required
This will set the first charge date of a new subscription.
- order_day_of_monthinteger
This is populated when order_interval_unit has value “month”. Default value is 0.
- order_day_of_weekinteger
This is populated when order_interval_unit has value “week”.
- order_interval_frequencyinteger* Required
The number of units, specified in order_interval_unit, between each order.
- order_interval_unitstring* Required
Possible values: day, week, month
The frequency unit used to determine when a subscription order is created.
- plan_idinteger
A Recharge Plan id that provides interval information. Automatically fills the following properties from the plan if they were not provided directly: charge_interval_frequency, expire_after_specific_number_of_charges, order_day_of_month, order_day_of_week, order_interval_frequency, order_interval_unit.
- pricestring
The price of the product.
- product_titlestring
The name of the product.
- propertiesarray
A list of key/value pairs representing properties of the subscription.
Show object attributes - quantityinteger* Required
The quantity of the product.
- external_product_idobject
Unique numeric identifier of the product id.
Show object attributes - external_variant_idobject* Required
Unique numeric identifier of the product variant id.
Show object attributes - statusstring
Possible values: active, cancelled, expired
Default is set to
active
.
More Parameters
Responses
- 201
successful response
Show response object
curl 'https://api.rechargeapps.com/subscriptions' \
-H 'X-Recharge-Version: 2021-11' \
-H 'Content-Type: application/json' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d '{
"address_id": 48563471,
"charge_interval_frequency": "30",
"next_charge_scheduled_at": "2021-12-17",
"order_interval_frequency": "30",
"order_interval_unit": "day",
"properties": [
{
"name": "Colour",
"value": "Yellow"
},
{
"name": "Bottle Material",
"value": "Glass"
}
],
"external_variant_id": {
"ecommerce": "32165284380775"
},
"quantity": 3
}'
{
"subscription": {
"id": 63898947,
"address_id": 48563471,
"customer_id": 37657002,
"analytics_data": {
"utm_params": {
"utm_source": "facebook",
"utm_medium": "cpc"
}
},
"cancellation_reason": null,
"cancellation_reason_comments": null,
"cancelled_at": null,
"charge_interval_frequency": 30,
"created_at": "2020-02-19T17:40:09+00:00",
"expire_after_specific_number_of_charges": null,
"external_product_id": {
"ecommerce": "4381728735283"
},
"external_variant_id": {
"ecommerce": "32165284380775"
},
"has_queued_charges": true,
"is_prepaid": false,
"is_skippable": true,
"is_swappable": false,
"max_retries_reached": false,
"next_charge_scheduled_at": "2021-12-17",
"order_day_of_month": null,
"order_day_of_week": null,
"order_interval_frequency": 30,
"order_interval_unit": "day",
"presentment_currency": "USD",
"price": "10.00",
"product_title": "ABC Shirt",
"properties": [
{
"name": "Color",
"value": "Blue"
},
{
"name": "Size",
"value": "Large"
}
],
"quantity": 1,
"sku": "TOM0001",
"sku_override": false,
"status": "active",
"updated_at": "2020-02-19T17:40:10+00:00",
"variant_title": "Blue star"
}
}
Retrieve a subscription
Retrieve a subscription using the Recharge subscription_id
.
Responses
- 200
successful response
Show response object
curl 'https://api.rechargeapps.com/subscriptions/27363808' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token'
{
"subscription": {
"id": 63898947,
"address_id": 48563471,
"customer_id": 37657002,
"analytics_data": {
"utm_params": {
"utm_source": "facebook",
"utm_medium": "cpc"
}
},
"cancellation_reason": null,
"cancellation_reason_comments": null,
"cancelled_at": null,
"charge_interval_frequency": 30,
"created_at": "2020-02-19T17:40:09+00:00",
"expire_after_specific_number_of_charges": null,
"external_product_id": {
"ecommerce": "4381728735283"
},
"external_variant_id": {
"ecommerce": "32165284380775"
},
"has_queued_charges": true,
"is_prepaid": false,
"is_skippable": true,
"is_swappable": false,
"max_retries_reached": false,
"next_charge_scheduled_at": "2021-12-17",
"order_day_of_month": null,
"order_day_of_week": null,
"order_interval_frequency": 30,
"order_interval_unit": "day",
"presentment_currency": "USD",
"price": "10.00",
"product_title": "ABC Shirt",
"properties": [
{
"name": "Color",
"value": "Blue"
},
{
"name": "Size",
"value": "Large"
}
],
"quantity": 1,
"sku": "TOM0001",
"sku_override": false,
"status": "active",
"updated_at": "2020-02-19T17:40:10+00:00",
"variant_title": "Blue star"
}
}
Update a subscription
Update an existing subscription.
Updating parameters like frequency
, charge_interval_frequency
, order_interval_frequency
, order_interval_unit
will cause our algorithm to automatically recalculate the next charge date (next_charge_scheduled_at
). If you want to change the next charge date (next_charge_scheduled_at
) we recommend you to update these parameters first.
When updating subscription status
attribute from cancelled
to active
, following attributes will be set to null: cancelled_at
, cancellation_reason
and cancellation_reason_comments
When updating order_interval_unit
OR order_interval_frequency
OR charge_interval_frequency
all three parameters are required.
Query Parameters
- commitboolean
Controls whether the
QUEUED
charges linked to the subscription should be regenerated upon subscription update. By default the flag is set tofalse
which will delay charge regeneration 5 seconds. This enables running multiple calls to perform changes and receive responses much faster since the API won’t wait for a charge regeneration to complete. Setting this parameter totrue
will cause charge regeneration to complete before returning a response. - force_updateboolean
If set to
True
, updates will also be applied toCANCELLED
subscriptions. Ifnull
orFalse
, onlyACTIVE
subscriptions will be updated.
More Parameters
Body Parameters
- charge_interval_frequencyinteger
Max: 1000
The number of units (specified in order_interval_unit) between each charge. Required when updating order_interval_unit.
WARNING: This update will remove skipped and manually changed charges. - expire_after_specific_number_of_chargesinteger
The number of charges until subscription expires.
- external_variant_idobject
Unique number identifier of the product variant in your external platform.
Show object attributes - order_day_of_monthstring
The day of the month the order is created on.
WARNING: this update will remove skips and manually changed charges. - order_day_of_weekstring
The day of the week the order is created on.
WARNING: this update will remove skips and manually changed charges. - order_interval_frequencystring
The number of units (specified in order_interval_unit) between each order. Required when updating order_interval_unit.
WARNING: This update will remove skipped and manually changed charges. - order_interval_unitstring
Possible values: day, week, month
The frequency unit used to determine when a subscription order is created.
WARNING: This update will remove skipped and manually changed charges. - plan_idinteger
A Recharge Plan id that provides interval information. Automatically fills the following properties from the plan if they were not provided directly: charge_interval_frequency, expire_after_specific_number_of_charges, order_day_of_month, order_day_of_week, order_interval_frequency, order_interval_unit.
- pricestring
The price of the item before discounts, taxes, or shipping has been applied.
- product_titlestring
The name of the product.
- propertiesarray
A list of key/value pairs representing properties of the subscription.
Show object attributes - quantitystring
The number of items in the subscription.
- skustring
A unique identifier of the item in the fulfillment.
- sku_overrideboolean
Flag is automatically updated to
true
when SKU is passed on create or update. Whensku_override
istrue
, the SKU on the subscription will be used to generate charges and orders.
Whensku_override
isfalse
, Recharge will dynamically fetch the sku from the corresponding external platform variant. - use_external_variant_defaultsboolean
Flag instructing to pull the
price
from the product variant passed.You need to pass the variant_id under
external_variant_id.ecommerce
and set this attribute totrue
in the request for the flag to work. - variant_titlestring
The name of the product variant.
More Parameters
Responses
- 200
successful response
Show response object
curl -X PUT 'https://api.rechargeapps.com/subscriptions/27363808' \
-H 'X-Recharge-Version: 2021-11' \
-H 'Content-Type: application/json' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d '{
"quantity": 4
}'
{
"subscription": {
"id": 63898947,
"address_id": 48563471,
"customer_id": 37657002,
"analytics_data": {
"utm_params": {
"utm_source": "facebook",
"utm_medium": "cpc"
}
},
"cancellation_reason": null,
"cancellation_reason_comments": null,
"cancelled_at": null,
"charge_interval_frequency": 30,
"created_at": "2020-02-19T17:40:09+00:00",
"expire_after_specific_number_of_charges": null,
"external_product_id": {
"ecommerce": "4381728735283"
},
"external_variant_id": {
"ecommerce": "32165284380775"
},
"has_queued_charges": true,
"is_prepaid": false,
"is_skippable": true,
"is_swappable": false,
"max_retries_reached": false,
"next_charge_scheduled_at": "2021-12-17",
"order_day_of_month": null,
"order_day_of_week": null,
"order_interval_frequency": 30,
"order_interval_unit": "day",
"presentment_currency": "USD",
"price": "10.00",
"product_title": "ABC Shirt",
"properties": [
{
"name": "Color",
"value": "Blue"
},
{
"name": "Size",
"value": "Large"
}
],
"quantity": 1,
"sku": "TOM0001",
"sku_override": false,
"status": "active",
"updated_at": "2020-02-19T17:40:10+00:00",
"variant_title": "Blue star"
}
}
Delete a subscription
We now have a feature for deleting a subscription.
Body Parameters
- send_emailboolean
When your store setting indicates that cancellation emails should be sent, this value determines if the email should be sent for the specified subscription cancellation.
If set to
true
, cancellation emails will be sent for the specified subscription cancellations. If set tofalse
, cancellation emails will not be sent.
More Parameters
Responses
- 204
successful response
Show response object
curl -X DELETE 'https://api.rechargeapps.com/subscriptions/27363808' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token'
{}
List subscriptions
Returns a list of all your subscriptions.
HTTP request examples
GET /subscriptions
GET /subscriptions?created_at_min=2018-10-10&created_at_max=2019-10-16
GET /subscriptions?customer_id=<customer_id>
Query Parameters
- address_idstring
Return the subscriptions linked to the given
address_id
.Not compatible with
address_ids
. - address_idsstring
Return the subscriptions linked to the given
address_ids
.Accepts a comma separated list of address_ids as value.
Not compatible withaddress_id
- created_at_maxstring
Return the subscriptions created before the given date.
- created_at_minstring
Return the subscriptions created after the given date.
- cursorstring
Return either the next or previous page of subscriptions using
next_cursor
orprevious_cursor
attributes in the response. - customer_idstring
Return the subscriptions linked to the given Recharge customer id.
- external_variant_idstring
Return the subscriptions linked to the given external_variant_id
- idsstring
Comma-separated list of
subscription_ids
to filter - limitstring
Default: 50
Max: 250
The number of results.
- pagestring*Deprecated
Default: 1
The page to show.
Page-based pagination has been deprecated but still available to use for pages up to a 100. If you need data past this point, use cursor pagination.
- statusstring
Possible values: active, cancelled, expired
Return the subscriptions with specified status.
- updated_at_maxstring
Return the subscriptions updated before the given date.
- updated_at_minstring
Return the subscriptions updated after the given date.
More Parameters
Responses
- 200
successful response
Show response object
curl 'https://api.rechargeapps.com/subscriptions' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d limit=3 -G
{
"next_cursor": "next_cursor",
"previous_cursor": "previous_cursor",
"subscriptions": [
{
"id": 63898947,
"address_id": 42171447,
"customer_id": 37657002,
"analytics_data": {
"utm_params": {
"utm_source": "facebook",
"utm_medium": "cpc"
}
},
"cancellation_reason": null,
"cancellation_reason_comments": null,
"cancelled_at": null,
"charge_interval_frequency": 1,
"created_at": "2020-02-19T17:40:09+00:00",
"expire_after_specific_number_of_charges": 5,
"external_product_id": {
"ecommerce": "4381728735283"
},
"external_variant_id": {
"ecommerce": "32165284380775"
},
"has_queued_charges": false,
"is_prepaid": false,
"is_skippable": true,
"is_swappable": false,
"max_retries_reached": false,
"next_charge_scheduled_at": "2021-12-17",
"order_day_of_month": null,
"order_day_of_week": null,
"order_interval_frequency": 1,
"order_interval_unit": "month",
"presentment_currency": "USD",
"price": "10.00",
"product_title": "ABC Shirt",
"properties": [
{
"name": "Color",
"value": "Blue"
},
{
"name": "Size",
"value": "Large"
}
],
"quantity": 1,
"sku": "TOM0001",
"sku_override": false,
"status": "active",
"updated_at": "2020-02-19T17:40:10+00:00",
"variant_title": "Blue star"
}
]
}
Change a subscription next charge date
Update an existing subscription’s next charge date.
If there are two active subscriptions with the same address_id
, and you update their next_charge_date
parameters to match, their charges will get merged into a new charge with a new id
Body Parameters
- datestring* Required
The next charge date desired.
More Parameters
Responses
- 200
successful response
Show response object
curl 'https://api.rechargeapps.com/subscriptions/27363808/set_next_charge_date' \
-H 'X-Recharge-Version: 2021-11' \
-H 'Content-Type: application/json' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d '{"date": "2021-08-05"}'
{
"subscription": {
"id": 27363808,
"address_id": 42171447,
"customer_id": 37657002,
"analytics_data": {
"utm_params": {
"utm_source": "facebook",
"utm_medium": "cpc"
}
},
"cancellation_reason": null,
"cancellation_reason_comments": null,
"cancelled_at": null,
"charge_interval_frequency": 1,
"created_at": "2020-02-19T17:40:09+00:00",
"expire_after_specific_number_of_charges": null,
"external_product_id": {
"ecommerce": "4381728735283"
},
"external_variant_id": {
"ecommerce": "32309455192167"
},
"has_queued_charges": true,
"is_prepaid": false,
"is_skippable": true,
"is_swappable": false,
"max_retries_reached": false,
"next_charge_scheduled_at": "2021-08-05",
"order_day_of_month": null,
"order_day_of_week": null,
"order_interval_frequency": 1,
"order_interval_unit": "month",
"presentment_currency": "USD",
"price": "10.00",
"product_title": "ABC Shirt",
"properties": [
{
"name": "Color",
"value": "Blue"
},
{
"name": "Size",
"value": "Large"
}
],
"quantity": 1,
"sku": "TOM0001",
"sku_override": false,
"status": "active",
"updated_at": "2020-02-19T17:40:10+00:00",
"variant_title": "Blue star"
}
}
Change a subscription address
New
Update an existing subscription’s address.
Body Parameters
- address_idinteger* Required
Unique id of the address that need to be associated with subscription.
- next_charge_scheduled_atstring
The next charge date desired.
More Parameters
Responses
- 200
successful response
Show response object
curl 'https://api.rechargeapps.com/subscriptions/27363808/change_address' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token' \
-H 'Content-Type: application/json' \
-d '{"address_id": 23397943}'
{
"subscription": {
"id": 27363808,
"address_id": 42171447,
"customer_id": 37657002,
"analytics_data": {
"utm_params": {
"utm_source": "facebook",
"utm_medium": "cpc"
}
},
"cancellation_reason": null,
"cancellation_reason_comments": null,
"cancelled_at": null,
"charge_interval_frequency": 1,
"created_at": "2020-02-19T17:40:09+00:00",
"expire_after_specific_number_of_charges": null,
"external_product_id": {
"ecommerce": "4381728735283"
},
"external_variant_id": {
"ecommerce": "32309455192167"
},
"has_queued_charges": true,
"is_prepaid": false,
"is_skippable": true,
"is_swappable": false,
"max_retries_reached": false,
"next_charge_scheduled_at": "2021-08-05",
"order_day_of_month": null,
"order_day_of_week": null,
"order_interval_frequency": 1,
"order_interval_unit": "month",
"presentment_currency": "USD",
"price": "10.00",
"product_title": "ABC Shirt",
"properties": [
{
"name": "Color",
"value": "Blue"
},
{
"name": "Size",
"value": "Large"
}
],
"quantity": 1,
"sku": "TOM0001",
"sku_override": false,
"status": "active",
"updated_at": "2020-02-19T17:40:10+00:00",
"variant_title": "Blue star"
}
}
Cancel a subscription
Cancel an active subscription.
An involuntary subscription cancelled due to max retries reached will trigger the charge/max_retries_reached webhook.
Body Parameters
- cancellation_reasonstring* Required
Reason for subscription cancellation.
- cancellation_reason_commentsstring
Cancellation reason comment. Maximum length is 1024 characters.
- send_emailboolean
If set to
false
, subscription cancelled email will not be sent to customer and store owner. Note: even if set to True, there are some conditions where an email will not be sent. They are: inactive subscription_cancellation email template, customer or subscription was created on the same day, subscription is for a membership, email already sent for this subscription in the last 24 hours, customer has other active subscriptions or onetimes
More Parameters
Responses
- 200
successful response
Show response object
curl 'https://api.rechargeapps.com/subscriptions/27363808/cancel' \
-H 'X-Recharge-Version: 2021-11' \
-H 'Content-Type: application/json' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d '{"cancellation_reason": "other reason"}'
{
"subscription": {
"id": 27363808,
"address_id": 42171447,
"customer_id": 37657002,
"analytics_data": {
"utm_params": {
"utm_source": "facebook",
"utm_medium": "cpc"
}
},
"cancellation_reason": null,
"cancellation_reason_comments": null,
"cancelled_at": null,
"charge_interval_frequency": 1,
"created_at": "2020-02-19T17:40:09+00:00",
"expire_after_specific_number_of_charges": null,
"external_product_id": {
"ecommerce": "4381728735283"
},
"external_variant_id": {
"ecommerce": "32309455192167"
},
"has_queued_charges": true,
"is_prepaid": false,
"is_skippable": true,
"is_swappable": false,
"max_retries_reached": false,
"next_charge_scheduled_at": "2021-08-05",
"order_day_of_month": null,
"order_day_of_week": null,
"order_interval_frequency": 1,
"order_interval_unit": "month",
"presentment_currency": "USD",
"price": "10.00",
"product_title": "ABC Shirt",
"properties": [
{
"name": "Color",
"value": "Blue"
},
{
"name": "Size",
"value": "Large"
}
],
"quantity": 1,
"sku": "TOM0001",
"sku_override": false,
"status": "active",
"updated_at": "2020-02-19T17:40:10+00:00",
"variant_title": "Blue star"
}
}
Activate a subscription
Activate a cancelled subscription.
When activating subscription, following attributes will be set to null: cancelled_at
, cancellation_reason
and cancellation_reason_comments
.
Responses
- 200
successful response
Show response object
curl 'https://api.rechargeapps.com/subscriptions/27363808/activate' \
-H 'X-Recharge-Version: 2021-11' \
-H 'Content-Type: application/json' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d '{}'
{
"subscription": {
"id": 27363808,
"address_id": 42171447,
"customer_id": 37657002,
"analytics_data": {
"utm_params": {
"utm_source": "facebook",
"utm_medium": "cpc"
}
},
"cancellation_reason": null,
"cancellation_reason_comments": null,
"cancelled_at": null,
"charge_interval_frequency": 1,
"created_at": "2020-02-19T17:40:09+00:00",
"expire_after_specific_number_of_charges": null,
"external_product_id": {
"ecommerce": "4381728735283"
},
"external_variant_id": {
"ecommerce": "32309455192167"
},
"has_queued_charges": true,
"is_prepaid": false,
"is_skippable": true,
"is_swappable": false,
"max_retries_reached": false,
"next_charge_scheduled_at": "2021-08-05",
"order_day_of_month": null,
"order_day_of_week": null,
"order_interval_frequency": 1,
"order_interval_unit": "month",
"presentment_currency": "USD",
"price": "10.00",
"product_title": "ABC Shirt",
"properties": [
{
"name": "Color",
"value": "Blue"
},
{
"name": "Size",
"value": "Large"
}
],
"quantity": 1,
"sku": "TOM0001",
"sku_override": false,
"status": "active",
"updated_at": "2020-02-19T17:40:10+00:00",
"variant_title": "Blue star"
}
}
Gift a skipped subscription
Gift a skipped Subscription
to a recipient.
Body Parameters
- purchase_item_idsarray* Required
A list containing the
Subscription
IDs to be skipped. - recipient_addressobject* Required
An object that contains information associated with the giftee’s Address
Show object attributes
More Parameters
Responses
- 200
successful response
Show response object - 422
invalid data passed to the endpoint
Show response object
curl 'https://api.rechargeapps.com/subscriptions/skip_gift' \
-H 'X-Recharge-Version: 2021-11' \
-H 'Content-Type: application/json' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d '{"recipient_address": {
"address1": "1776 Washington Street",
"address2": "",
"city": "Los Angeles",
"company": "Recharge",
"country_code": "US",
"first_name": "John",
"last_name": "Doe",
"phone": "5551234567",
"province": "California",
"zip": "90404", "email": "fake@example.com"
},
"purchase_item_ids": [27363808, 27363809]}'
{
"onetimes": [
{
"id": 16909886,
"address_id": 45154492,
"created_at": "2021-05-24T19:14:25+00:00",
"customer_id": 40565990,
"external_product_id": {
"ecommerce": "4950280863846"
},
"external_variant_id": {
"ecommerce": "32139793137766"
},
"is_cancelled": false,
"next_charge_scheduled_at": "2021-12-17T05:00:00+00:00",
"price": "6.00",
"product_title": "ABC Shirt",
"properties": [
{
"name": "Color",
"value": "Blue"
}
],
"quantity": 1,
"sku": "TOM0001",
"sku_override": false,
"updated_at": "2021-05-24T19:30:14+00:00",
"variant_title": "Blue star"
}
]
}
Webhook endpoints
Webhooks are a mechanism for reacting to specific events that are triggered in the Recharge system.
For example, a checkout completion, a customer activation or subscription cancellation. Webhooks will deliver you the data of the specific event in real-time. This data can be used to custom code logic behind automated subscription management, dashboards creation, discounts applying…
When a webhook is triggered, the payload will be identical to the payload you would receive from another API endpoint.
For example, a webhook on subscription/created will be identical to the payload for retrieving a subscription by ID from the Recharge API.
There are a lot of things that can be done via Webhooks: It can be used to collect all kinds of data from our API and then create a custom Dashboard to show how much and when your customers are buying in real time, or use all this data to do Analytics of some kind in order to create a better customer experience. Webhooks can be used as a “Trigger” on your backend to update subscription products.
If you have some kind of a Subscription where you want to change the Product that the customer gets every month, you can do it by waiting for an order/created webhook on your backend, and when it fires you can make an API call to change the Product of that subscription or the next shipping date, etc.
Retries / Idempotency
Due to webhook retries, it’s possible that your application receives the same webhook more than once. Ensure idempotency of the webhook call by detecting such duplicates within your application.
Respond to a webhook
Your webhook acknowledges that it received data by sending a
200
OK response. Any response outside of the 200
range will let Recharge know that you didn’t receive your webhook. Recharge has implemented a 5 second time-out period. We wait 5 seconds, if our system doesn’t get a response in that period we consider that request as failed. Our system will try 20 times to send the same webhook over the next 2 days, if the request fails every time our system will delete this webhook. At this moment our system is logging those deleted webhooks.
The Webhook object
Please note that the store domain header has changed to X-Recharge-External-Platform-Domain
Related guides: Extending webhook responses
These topics are deprecated, however they will not be removed from this API version: checkout/completed
.
Attributes
- idinteger
Unique numeric identifier for the webhook.
- addressstring
The URI where the webhook should send the
POST
request when the event occurs. - included_objectsarray
Possible values: addresses, collections, customer, metafields
List of objects set to enrich the webhook payload. cf. extending responses
- topicstring
The event that will trigger the webhook.
- versionstring
The version of the API used to populate the body of the webhook. If not set, this will default to the store’s default API version.
Error related attributes
More Attributes
{
"webhook": {
"id": 19451,
"address": "https://request.in/foo",
"topic": "subscription/created",
"version": "2021-11"
}
}
Available webhooks
The list of all available webhooks.
Related guides: Webhook payload examples
Object | Topics | Scope Required |
---|---|---|
Address |
address/created address/updated |
read_customers |
Async_batch |
async_batch/processed |
read_batches |
BundleSelection |
bundle_selection/created bundle_selection/updated bundle_selection/deleted |
read_subscriptions |
Customer |
customer/activated customer/created customer/deactivated customer/payment_method_updated customer/updated customer/deleted |
read_customers |
Charge |
charge/created charge/failed charge/max_retries_reached charge/paid charge/refunded charge/uncaptured charge/upcoming charge/updated charge/deleted |
read_orders |
Checkout |
checkout/created checkout/completed checkout/processed checkout/updated Note: Deprecated and not available for the Shopify integration with Shopify hosted Checkout (aka SCI) |
read_orders |
Onetime |
onetime/created onetime/deleted onetime/updated |
read_subscriptions |
Order |
order/cancelled order/created order/deleted order/processed order/payment_captured order/upcoming order/updated order/success |
read_orders |
Plan |
plan/created plan/deleted plan/updated |
read_products |
Subscription |
subscription/activated subscription/cancelled subscription/created subscription/deleted subscription/skipped subscription/updated subscription/unskipped subscription/swapped subscription/paused |
read_subscriptions |
Store |
store/updated |
read_store |
Recharge |
recharge/uninstalled |
store_info |
Create a webhook
Create a webhook. Currently, only the API can be used to create a webhook.
To register a webhook endpoint for a specific object, you will need to have read permissions for that object on your Recharge API token. For example, if you want to register a webhook for subscription/created, you will need the read_subscriptions
permissions.
Each API token can register a maximum of 10 webhooks of the same topic.
Related guides: Webhook payload examples
Related guides: Extending webhook responses
Body Parameters
- addressinteger* Required
The URI where the webhook should send the
POST
request when the event occurs. - included_objectsarray
Possible values: addresses, collections, customer, metafields
List of objects set to enrich the webhook payload. cf. extending responses
- topicinteger* Required
The event that will trigger the webhook.
- versionstring
The version of the API used to populate the body of the webhook. If not set, this will default to the store’s default API version.
More Parameters
Responses
- 200
successful response
Show response object
curl -i -H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token' \
-H 'Content-Type: application/json' \
-H 'Accept: application/json' \
-X POST 'https://api.rechargeapps.com/webhooks' \
--data '{"address": "https://request.in/foo", "topic": "subscription/created"}'
{
"webhook": {
"id": 6,
"address": "https://request.in/foo",
"topic": "subscription/created",
"version": "2021-01"
}
}
Retrieve a webhook
Retrieve a webhook using the Recharge webhook id.
Responses
- 200
successful response
Show response object
curl -i -H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token' \
-H 'Content-Type: application/json' \
-H 'Accept: application/json' \
-X GET https://api.rechargeapps.com/webhooks/19451
{
"webhook": {
"id": 6,
"address": "https://request.in/foo",
"topic": "subscription/created",
"version": "2021-01"
}
}
Update a webhook
Modifies an existing Webhook
to match the specified parameters.
Related guides: Extending webhook responses
Body Parameters
- addressstring
The URI where the webhook should send the POST request when the event occurs.
- included_objectsarray
Possible values: addresses, collections, customer, metafields
List of objects set to enrich the webhook payload. cf. extending responses
- topicstring
The event that will trigger the webhook.
- versionstring
The version of the API used to populate the body of the webhook.
More Parameters
Responses
- 200
successful response
Show response object
curl -i -H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token' \
-H 'Content-Type: application/json' \
-H 'Accept: application/json' \
-X PUT 'https://api.rechargeapps.com/webhooks/958' \
--data '{"address": "https://request.in/foo"}'
{
"webhook": {
"id": 6,
"address": "https://request.in/foo",
"topic": "subscription/created",
"version": "2021-01"
}
}
Delete a webhook
Delete a Webhook
Responses
- 204
Content Deleted: The server has successfully fulfilled the request and deleted the desired object and there is no content to send in the response body.
Show response object
curl -i -H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token' \
-H 'Content-Type: application/json' \
-H 'Accept: application/json' \
-X DELETE 'https://api.rechargeapps.com/webhooks/958'
{}
List webhooks
Returns all the webhooks of the given store that are owned by the current requesting client (note that a a private token shows all of the store’s webhooks, an integration token only shows that integration’s webhooks).
Responses
- 200
successful response
Show response object
curl -i -H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token' \
-H 'Content-Type: application/json' \
-H 'Accept: application/json' \
-X GET 'https://api.rechargeapps.com/webhooks'
{
"webhooks": [
{
"id": 19451,
"address": "https://request.in/foo",
"topic": "subscription/created",
"version": "2021-11"
},
{
"id": 19453,
"address": "https://request.in/foo",
"topic": "subscription/cancelled",
"version": "2021-11"
}
]
}
Test webhooks
In order to test webhook connectivity, you can trigger the dispatch of a test webhook with our webhook test endpoint. Sending a POST request to the webhook endpoint containing a webhook id will dispatch an empty webhook body to the specified webhook’s destination url.
Responses
- 200
successful response
Show response object
curl -i -H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token' \
-H 'Content-Type: application/json' \
-H 'Accept: application/json' \
--data '{}'
-X POST https://api.rechargeapps.com/webhooks/<webhook_id>/test
{}
Webhooks explained
Here you will find what specific action triggers a given webhook.
Address webhooks
To use these webhooks your API Token must have read permissions for Customers
enabled ( read_customer
).
Topic | Explanation |
---|---|
address/created |
This will trigger when you create an address via API, or when you go through the checkout with a particular address for the first time with the same customer. |
address/updated |
This will trigger when you update an address via API, or when you update the address via UI. It will also trigger whenever a subscription has been activated or cancelled. |
Bundle webhooks
To use these webhooks your API Token must have read permissions for Subscriptions
enabled ( read_subscriptions
).
Topic | Explanation |
---|---|
bundle_selection/created |
This will trigger when a new selection is created for a Bundle subscription. |
bundle_selection/updated |
This will trigger when a Bundle Selection is succesfully updated. |
bundle_selection/deleted |
This will trigger when a Bundle Selection is deleted. |
Charge webhooks
To use these webhooks your API Token must have read permissions for Orders
enabled ( read_orders
).
Topic | Explanation |
---|---|
charge/created |
This will trigger when a charge is created. |
charge/failed |
This will trigger every time we try to process a charge and it fails due to various reasons (insufficient funds, invalid CC info, expired CC, etc.) on both API and UI. |
charge/max_retries_reached |
This will trigger after we attempt to process a charge 8 times, and it failed every time due to various CC issues. This can be triggered on both UI (manually retry a charge 8 times and fail) and API. |
charge/paid |
This will trigger when a charge is successfully processed, both manually via UI and automatic recurring charge. This will not trigger on the checkout itself. |
charge/refunded |
This will trigger when a charge is successfully refunded, either partially or in full. It will fire if a charge has been refunded both manually via UI and through an API request. |
charge/upcoming |
This will trigger X days before the upcoming charge is scheduled. The default is 3 days but your store specific setting can be verified on the Notification Settings page in the description of the Upcoming charge customer notification. |
charge/updated |
This will trigger when applying a discount, a change to charge that recalculates shipping rates as well as if next_charge_date is updated on charge endpoint charges/<charge_id>/change_next_charge_date. |
charge/deleted |
This will trigger when a subscription is cancelled and upcoming charges are deleted. |
Checkout webhooks
To use these webhooks your API Token must have read permissions for Orders
enabled ( read-orders
).
Topic | Explanation |
---|---|
checkout/created |
This will trigger when a checkout is successfully created. |
checkout/completed |
Will be deprecated. |
checkout/processed |
This will trigger when a checkout is successfully processed. |
checkout/updated |
This will trigger when a checkout is successfully updated. |
Customer webhooks
To use these webhooks your API Token must have read permissions for Customers
enabled ( read_customers
).
Topic | Explanation |
---|---|
customer/activated |
This will trigger when you activate* a customer * activating means that you have added a subscription to a customer who didn’t have an active subscription previously. |
customer/created |
This will trigger when you create a customer via API or go through the checkout with a particular email address for a first time customer. |
customer/deactivated |
This will trigger when the last subscription a customer had expires, so he no longer has ANY active subscriptions (which means there are no QUEUED charges/orders for this customer). |
customer/payment_method_updated |
This will trigger only* when you update the payment_token from the UI * We are working on triggering this when you do the update from the API as well. |
customer/updated |
This will trigger when you update a customer via both API and UI. |
customer/deleted |
This will trigger when you delete a customer via both API and UI. |
Onetime webhooks
To use these webhooks your API Token must have read permissions for Subscriptions
enabled ( read_subscriptions
).
Topic | Explanation |
---|---|
onetime/created |
This will trigger when you create a one time product via API. |
onetime/deleted |
This will trigger when you delete a one time product via API. |
onetime/updated |
This will trigger when you update a one time product via API. |
Order webhooks
To use these webhooks your API Token must have read permissions for Orders
enabled ( read_orders
).
Topic | Explanation |
---|---|
order/created |
This will trigger when an order is created (when a charge is successfully processed) * In case of prepaid Orders there will be multiple order/created webhooks fired, one for every queued order created at once. |
order/deleted |
This will trigger when an order is deleted. |
order/processed |
This will trigger when the order is processed (when an order goes from status queued to status success ). This will not trigger on checkout. |
order/upcoming |
This will trigger X days before a QUEUED (prepaid) order is scheduled to be processed. The default is 3 days. |
order/updated |
This will trigger when an order is updated. |
Plan webhooks
To use these webhooks your API Token must have read permissions for Products
enabled ( read_products
).
Topic | Explanation |
---|---|
plan/created |
This will trigger when a plan is created by one of the following methods: via API, via Merchant portal or when using the 2021-01 Products endpoint. |
plan/deleted |
This will trigger when a plan is deleted by one of the following methods: via API, via Merchant portal or when using the 2021-01 Products endpoint. |
plan/updated |
This will trigger when a plan is updated by one of the following methods: via API, via Merchant portal or when using the 2021-01 Products endpoint… |
Subscription webhooks
To use these webhooks your API Token must have read permissions for Subscriptions
enabled ( read_subscriptions
).
Topic | Explanation |
---|---|
subscription/activated |
This will trigger when you activate a subscription via API or UI. |
subscription/cancelled |
This will trigger when you cancel a subscription via API or UI. An involuntary subscription cancelled due to max retries reached will only trigger the charge/max_retries_reached webhook and not the subscription/cancelled webhook. |
subscription/created |
This will trigger when you create a subscription via API or when you go through the checkout on UI. |
subscription/deleted |
This will trigger when you delete a subscription via API or UI. |
subscription/skipped |
This will trigger when you skip a subscription within a charge, meaning that you only skip a particular subscription (the subscription_id you send in the body) in that charge if there are multiple subscriptions related to that charge. |
subscription/unskipped |
This will trigger when you unskip a subscription within a charge, meaning you only unskip a particular subscription (the subscription_id you send in the body) in that charge if there are multiple subscriptions related to that charge. |
subscription/updated |
This will trigger when you update a subscription via API (PUT method) or when you update the subscription via UI. This will also trigger when you update next charge date on Customer Portal, or when you change it using subscription endpoint subscriptions/ <subscription_id>set_next_charge_date . |
subscription/swapped |
This will trigger when you swap a subscription product for a given address to a different product or product variation API or UI. |
subscription/paused |
This will trigger when a customer pauses a subscription from within the customer portal. |
Other webhooks
To use these webhooks your API Token must have read permissions for Store
enabled ( read_store
).
Topic | Explanation |
---|---|
app/uninstalled |
This will trigger when you uninstall OAuth app on your store. |
recharge/uninstalled |
This will trigger when Recharge is uninstalled. |
store/updated |
This will trigger when and update has been made to the store. |
Webhook validation
Webhooks created through the API can be verified by calculating a digital signature. Each Webhook request includes an X-Recharge-Hmac-Sha256 header which is generated using the API Client Secret, along with the data sent in the request.
API Client Secret is not the same as your API token and it can be found at:
Recharge Dashboard—>Integrations—>API Tokens—>Click on your token
Edit API Token page will appear and there you will find API Client Secret
The request_body must be in JSON string format. Validation will fail even if one space is lost in process of JSON string generation.
Then use code similar to the example by adapting it to the programming language that you are using for your project.
Related guides: Example code for validating webhooks
Async batch Endpoints
The Async batches API can be used for processing large volumes of operations asynchronously, in order to reduce aggregate processing time and network traffic when interacting with many unique objects. For example, a user can leverage async_batches to create 1000 discounts with only 3 API requests.
As shown in the diagram below, the necessary steps to create and process a batch are:
1. Create an async_batch with the desired batch_type
2. Add tasks (individual operations) to your batch. You can add up to 1,000 tasks with each request, up to 10,000 tasks per batch.
3. Submit the batch for processing. Until a batch is submitted for processing, no tasks are attempted.
4. You may retrieve the batch to view progress details while it processes, or register for the async_batch/processed
webhook to receive immediate notification of batch completion.
5. Page through the tasks in the batch to view results of each completed or failed task.
Related guides: Examples of Async batches
The async batch object
Async batch
contains all the setups information and status update of a batch of operation to be performed collectively.
Batches will only leverage resources from the same version they are created in. e.g. a batch created with 2021-11
will only process tasks from the 2021-11
version.
Attributes
- idstring
Unique identifier of the batch, used for adding tasks to a batch and to initiate batch processing
- batch_typestring
Possible values: discount_create, discount_update, discount_delete, bulk_plans_create, bulk_plans_update, bulk_plans_delete, onetime_create, onetime_delete
Indicates the object type and operation required for all tasks in a batch
- closed_atstring
Indicates the date and time that processing was completed for a batch
- created_atstring
Indicates the date and time a batch was created
- fail_task_countstring
Indicates the number of failed tasks in a batch, during or after processing
- statusstring
Indicates the status of the batch. Available statuses are not_started, processing, completed, failed
- submitted_atstring
Indicates the date and time that a batch was triggered to process
- success_task_countstring
Indicates the number of successful tasks in a batch, during or after processing.
- total_task_countstring
Indicates the total number of tasks in a batch
- updated_atstring
Indicates the date and time at which a batch was last updated
- versionstring
Indicates the API version of the batch.
read-only field
Error related attributes
More Attributes
{
"async_batch": {
"id": 19,
"batch_type": "discount_create",
"closed_at": null,
"created_at": "2022-02-17T08:52:10+00:00",
"deleted_at": null,
"fail_task_count": 0,
"status": "not_started",
"submitted_at": null,
"success_task_count": 0,
"total_task_count": 0,
"updated_at": "2022-02-17T08:52:10+00:00",
"version": "2021-11"
}
}
Create a batch
In order to create, retrieve, or process batches, the API token requires the write_batches permission. Additional permissions are required, dependent upon the indicated batch_type. For example, for a batch type of discount_create, the API token requires the write_discounts permission.
Create a new batch, and indicate the desired outcome of the batch using batch_type.
CAUTION
Async batches and their tasks will only be retained in Recharge for one month after batch creation.
Available batch types
The async_batches API supports many functions, each identified as a batch_type. Typically, the task body for a batch_type will be representative of a single request to our standard endpoints, however, some variations may be present.
Related guides: Examples of Async Batches
Batches will only leverage resources from the same version they are created in. e.g. a batch created with 2021-11
will only process tasks from the 2021-11
version.
Body Parameters
- batch_typestring
Possible values: discount_create, discount_update, discount_delete, bulk_plans_create, bulk_plans_update, bulk_plans_delete, onetime_create, onetime_delete
Desired batch type
More Parameters
Responses
- 201
successful response
Show response object
curl -i -H 'X-Recharge-Access-Token: your_api_token' \
-H 'X-Recharge-Version: 2021-11' \
-H "Accept: application/json" \
-H "Content-Type: application/json" \
-X POST 'https://api.rechargeapps.com/async_batches' \
--data '{ "batch_type": "desired_batch_type"}'
{
"async_batch": {
"id": 19,
"batch_type": "discount_create",
"closed_at": null,
"created_at": "2022-10-12T20:16:09+00:00",
"deleted_at": null,
"fail_task_count": 0,
"status": "not_started",
"submitted_at": null,
"success_task_count": 0,
"total_task_count": 0,
"updated_at": "2022-10-12T20:16:09+00:00",
"version": "2021-11"
}
}
Retrieve a batch
Retrieve a batch using the Recharge batch id. A batch can be retrieved during processing to evaluate batch progress.
## HTTP request examples
GET /async_batches/<batch_id>
Responses
- 200
successful response
Show response object
curl -i -H 'X-Recharge-Access-Token: your_api_token' \
-H 'X-Recharge-Version: 2021-11' \
-H "Accept: application/json" \
-H "Content-Type: application/json" \
-X GET 'https://api.rechargeapps.com/async_batches/16'
{
"async_batch": {
"id": 19,
"batch_type": "discount_create",
"closed_at": null,
"created_at": "2022-10-12T20:16:09+00:00",
"deleted_at": null,
"fail_task_count": 0,
"status": "not_started",
"submitted_at": null,
"success_task_count": 0,
"total_task_count": 0,
"updated_at": "2022-10-12T20:16:09+00:00",
"version": "2021-11"
}
}
List batches
Returns a list of all your async_batches.
Responses
- 200
successful response
Show response object
curl -i -H 'X-Recharge-Access-Token: your_api_token' \
-H 'X-Recharge-Version: 2021-11' \
-H "Accept: application/json" \
-H "Content-Type: application/json" \
-X GET 'https://api.rechargeapps.com/async_batches'
{
"async_batches": [
{
"id": 20,
"batch_type": "discount_delete",
"closed_at": null,
"created_at": "2022-10-12T20:16:09+00:00",
"deleted_at": null,
"fail_task_count": 0,
"status": "not_started",
"submitted_at": null,
"success_task_count": 0,
"total_task_count": 40,
"updated_at": "2022-10-12T20:16:09+00:00",
"version": "2021-11"
},
{
"id": 19,
"batch_type": "discount_update",
"closed_at": null,
"created_at": "2022-10-12T20:16:09+00:00",
"deleted_at": null,
"fail_task_count": 0,
"status": "not_started",
"submitted_at": null,
"success_task_count": 0,
"total_task_count": 0,
"updated_at": "2022-10-12T20:16:09+00:00",
"version": "2021-11"
},
{
"id": 16,
"batch_type": "discount_create",
"closed_at": "2022-10-12T20:16:09+00:00",
"created_at": "2022-10-12T20:16:09+00:00",
"deleted_at": null,
"fail_task_count": 0,
"status": "completed",
"submitted_at": "2022-10-12T20:16:09+00:00",
"success_task_count": 2000,
"total_task_count": 2000,
"updated_at": "2022-10-12T20:16:09+00:00",
"version": "2021-11"
}
]
}
Process a batch
Until a batch is submitted for processing, no tasks are attempted. Once you have ensured the tasks in a batch are ready for processing, you can submit for processing using this endpoint and the associated batch id.
Webhooks - Batches process quickly, and may result in Recharge dispatching many webhooks at a high rate. Ensure any systems subscribed to resulting webhooks are capable of handling such load.
Responses
- 201
successful response
Show response object
curl -X POST 'https://api.rechargeapps.com/async_batches/21/process' \
-H 'X-Recharge-Version: 2021-11' \
-H 'Content-Type: application/json' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d '{}'
{
"async_batch": {
"id": 21,
"batch_type": "discount_create",
"closed_at": null,
"created_at": "2022-06-05T10:53:36+00:00",
"deleted_at": null,
"fail_task_count": 0,
"status": "processing",
"submitted_at": "2022-06-05T10:54:54+00:00",
"success_task_count": 0,
"total_task_count": 10,
"updated_at": "2022-06-05T10:54:54+00:00",
"version": "2021-11"
}
}
Async batch Tasks
Most commonly, the response from listing tasks on an async_batch id will be identical to the body of a singular request to standard Recharge API endpoints. However, in some instances there are variations from the standard list task response body. See below for examples corresponding to each batch_type.
Related guides: Examples of async batches
The async batch Tasks object
Attributes
- batch_idinteger
Indicates the batch that the task is contained by
- bodyobject
This object contains the body of a standard API request. For example, when creating discounts with a discount_create batch, this parameter contains a standard body for POST /discounts
- completed_atdatetime
Indicates the date and time a task was completed
- created_atdatetime
Indicates the date and time that a task was created and added to the batch
- deleted_atdatetime
Indicates the date and time that a task was deleted from the batch
- idinteger
Unique identifier for the task within a batch
- queued_atdatetime
Indicates the date and time a task was queued for processing within batch processing
- resultstring
Contains the individual response body for the task
- started_atdatetime
Indicates the date and time a task was processed
- statusstring
Possible values: pending, processing, failed, success
Indicates the status of the task within a batch.
- updated_atdatetime
Indicates the date and time a task was most recently updated
Error related attributes
More Attributes
Create a batch task
A batch contains operations of similar type (indicated by batch_type), called tasks. A task is typically representative of a single API request, with a body parameter containing the standard request body for the associated batch_type. A single request to add tasks may contain up to 1,000 tasks, and a batch may contain up to 10,000 tasks.
##HTTP examples
POST /async_batches/<batch_id>/tasks
Batches will only leverage resources from the same version they are created in. e.g. a batch created with 2021-11
will only process tasks from the 2021-11
version.
Body Parameters
- batch_idinteger
Indicates the batch that the task is contained by
- bodyobject
This object contains the body of a standard API request. For example, when creating discounts with a discount_create batch, this parameter contains a standard body for POST /discounts
- completed_atdatetime
Indicates the date and time a task was completed
- created_atdatetime
Indicates the date and time that a task was created and added to the batch
- deleted_atdatetime
Indicates the date and time that a task was deleted from the batch
- idinteger
Unique identifier for the task within a batch
- queued_atdatetime
Indicates the date and time a task was queued for processing within batch processing
- resultstring
Contains the individual response body for the task
- started_atdatetime
Indicates the date and time a task was processed
- statusstring
Possible values: pending, processing, failed, success
Indicates the status of the task within a batch.
- updated_atdatetime
Indicates the date and time a task was most recently updated
More Parameters
Responses
- 200
successful response
Show response object
curl -i -H 'X-Recharge-Access-Token: your_api_token' \
-H 'X-Recharge-Version: 2021-11' \
-H "Accept: application/json" \
-H "Content-Type: application/json" \
-X POST https://api.rechargeapps.com/async_batches/21/tasks \
--data '{
"tasks": [
{
"body": {
"code": "indexing",
"discount_type": "shipping",
"duration": "forever",
"status": "enabled",
"value": 100
}
},
{
"body": {
"code": "maroon",
"discount_type": "shipping",
"duration": "forever",
"status": "enabled",
"value": 100
}
},
{
"body": {
"code": "web services",
"value": 100,
"discount_type": "shipping",
"duration": "forever",
"status": "enabled"
}
},
{
"body": {
"code": "PCI",
"discount_type": "shipping",
"duration": "forever",
"status": "enabled",
"value": 100
}
},
{
"body": {
"code": "Cheese",
"discount_type": "shipping",
"duration": "forever",
"status": "enabled",
"value": 100
}
},
{
"body": {
"code": "overriding",
"discount_type": "shipping",
"duration": "forever",
"status": "enabled",
"value": 100
}
},
{
"body": {
"code": "bandwidth-monitored",
"value": 100,
"discount_type": "shipping",
"duration": "forever",
"status": "enabled"
}
},
{
"body": {
"code": "Falkland Islands (Malvinas)",
"discount_type": "shipping",
"duration": "forever",
"status": "enabled",
"value": 100
}
},
{
"body": {
"code": "Computer",
"discount_type": "shipping",
"duration": "forever",
"status": "enabled",
"value": 100
}
},
{
"body": {
"code": "digital",
"discount_type": "shipping",
"duration": "forever",
"status": "enabled",
"value": 100
}
}
]
}'
{
"count": 10
}
List batch tasks
Returns a list of all tasks in the indicated batch, using batch id. For batches with many tasks, pagination is supported when listing tasks. Use this function to evaluate the task-level results of a batch during, or after processing. Task results will be contained in the results
object of each task.
Query Parameters
- idsstring
Filter tasks by id. If passing multiple values, must be comma separated. Non-integer values will result in a 422 error
More Parameters
Responses
- 200
successful response
Show response object
curl -i -H 'X-Recharge-Access-Token: your_api_token' \
-H 'X-Recharge-Version: 2021-11' \
-H "Accept: application/json" \
-H "Content-Type: application/json" \
-X GET https://api.rechargeapps.com/async_batches/16/tasks
{
"next_cursor": null,
"previous_cursor": null,
"async_batch_tasks": [
{
"id": 1490831511,
"batch_id": 765278,
"body": {
"code": "Computer",
"discount_type": "shipping",
"duration": "forever",
"status": "enabled",
"value": 100
},
"completed_at": null,
"created_at": "2022-02-17T06:40:53+00:00",
"deleted_at": null,
"queued_at": null,
"result": null,
"started_at": null,
"status": "pending",
"updated_at": "2022-02-17T06:40:53+00:00"
},
{
"id": 1490831510,
"batch_id": 765278,
"body": {
"code": "Falkland Islands (Malvinas)",
"discount_type": "shipping",
"duration": "forever",
"status": "enabled",
"value": 100
},
"completed_at": null,
"created_at": "2022-02-17T06:40:53+00:00",
"deleted_at": null,
"queued_at": null,
"result": null,
"started_at": null,
"status": "pending",
"updated_at": "2022-02-17T06:40:53+00:00"
},
{
"id": 1490831509,
"batch_id": 765278,
"body": {
"code": "bandwidth-monitored",
"discount_type": "shipping",
"duration": "forever",
"status": "enabled",
"value": 100
},
"completed_at": null,
"created_at": "2022-02-17T06:40:53+00:00",
"deleted_at": null,
"queued_at": null,
"result": null,
"started_at": null,
"status": "pending",
"updated_at": "2022-02-17T06:40:53+00:00"
},
{
"id": 1490831508,
"batch_id": 765278,
"body": {
"code": "overriding",
"discount_type": "shipping",
"duration": "forever",
"status": "enabled",
"value": 100
},
"completed_at": null,
"created_at": "2022-02-17T06:40:53+00:00",
"deleted_at": null,
"queued_at": null,
"result": null,
"started_at": null,
"status": "pending",
"updated_at": "2022-02-17T06:40:53+00:00"
},
{
"id": 1490831507,
"batch_id": 765278,
"body": {
"code": "Cheese",
"discount_type": "shipping",
"duration": "forever",
"status": "enabled",
"value": 100
},
"completed_at": null,
"created_at": "2022-02-17T06:40:53+00:00",
"deleted_at": null,
"queued_at": null,
"result": null,
"started_at": null,
"status": "pending",
"updated_at": "2022-02-17T06:40:53+00:00"
},
{
"id": 1490831506,
"batch_id": 765278,
"body": {
"code": "PCI",
"discount_type": "shipping",
"duration": "forever",
"status": "enabled",
"value": 100
},
"completed_at": null,
"created_at": "2022-02-17T06:40:53+00:00",
"deleted_at": null,
"queued_at": null,
"result": null,
"started_at": null,
"status": "pending",
"updated_at": "2022-02-17T06:40:53+00:00"
},
{
"id": 1490831505,
"batch_id": 765278,
"body": {
"code": "web services",
"discount_type": "shipping",
"duration": "forever",
"status": "enabled",
"value": 100
},
"completed_at": null,
"created_at": "2022-02-17T06:40:53+00:00",
"deleted_at": null,
"queued_at": null,
"result": null,
"started_at": null,
"status": "pending",
"updated_at": "2022-02-17T06:40:53+00:00"
},
{
"id": 1490831504,
"batch_id": 765278,
"body": {
"code": "maroon",
"discount_type": "shipping",
"duration": "forever",
"status": "enabled",
"value": 100
},
"completed_at": null,
"created_at": "2022-02-17T06:40:53+00:00",
"deleted_at": null,
"queued_at": null,
"result": null,
"started_at": null,
"status": "pending",
"updated_at": "2022-02-17T06:40:53+00:00"
},
{
"id": 1490831503,
"batch_id": 765278,
"body": {
"code": "indexing",
"discount_type": "shipping",
"duration": "forever",
"status": "enabled",
"value": 100
},
"completed_at": null,
"created_at": "2022-02-17T06:40:53+00:00",
"deleted_at": null,
"queued_at": null,
"result": null,
"started_at": null,
"status": "pending",
"updated_at": "2022-02-17T06:40:53+00:00"
}
]
}
Token information
This resource allows a caller to inspect basic information regarding the token
in use. It will only return a single object related to the calling token
( ie - a call with api_token.id=1
will only return information regarding api_token.id=1
.
The token information object
Details of the client which generated the token.
If the API token was generated by an OAuth app, the object will contain associated client information.
If the API token was generated by the merchant, the object will reflect the name
prefaced by ‘[Private App]’ and contact_email
of the token.
Attributes
- clientobject
If the API Token was generated by an Oauth app, an object containing associated client information.
Show object attributes - contact_emailstring
The email associated with the API Token record.
For tokens created via OAuth App the contact can be
null
- namestring
The name of the API Token as created by merchant.
- scopesarray
A list of scopes on the API Token.
Error related attributes
More Attributes
{
"client": {
"name": "PARTNER",
"contact_email": "ed@partner.com"
},
"contact_email": "jbluhm@example.com",
"name": "token name",
"scopes": [
"read_shop",
"write_payments"
]
}
Retrieve token information
Retrieve token details.
Responses
- 200
successful response
Show response object
curl 'https://api.rechargeapps.com/token_information' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token'
{
"token_information": {
"client": {
"name": "PARTNER",
"contact_email": "ed@partner.com"
},
"contact_email": "jbluhm@example.com",
"name": "token name",
"scopes": [
"read_store",
"write_payment_methods"
]
}
}
Accounts
This resource allows a caller to inspect basic information regarding the staff accounts in the store.
The account object
An Account
record represents a staff account details for the Store
. One Store
can have many staff’s account.
Attributes
- idinteger
Unique numeric identifier for the
Account
. - user_idinteger
The unique numeric identifier of the user associated to the
Account
.There can only be one
Account
per user perStore
. - created_atdatetime
The date and time when the
Account
was created. - invited_atdatetime
The date and time the user was last invited to open their
Account
null
for the store creator.
If an invite to open anAccount
is re-issued for a user,invited_at
will hold the timestamp of the most recent invite sent. - is_ownerboolean
Flags whether this user is the store owner.
A
Store
can have multiple owners.
Error related attributes
More Attributes
{
"account": {
"id": 3315125,
"user_id": 2057785,
"created_at": "2021-07-20T09:10:19+00:00",
"invited_at": "2021-07-18T09:10:19+00:00",
"is_owner": false
}
}
Retrieve an account
Retrieves an account for the store.
Responses
- 200
successful response
Show response object
curl 'https://api.rechargeapps.com/accounts/331512' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token'
{
"account": {
"id": 3315125,
"user_id": 2057785,
"created_at": "2021-07-20T09:10:19+00:00",
"invited_at": "2021-07-18T09:10:19+00:00",
"is_owner": false
}
}
List accounts
Returns all accounts associated to the store.
Responses
- 200
successful response
Show response object
curl 'https://api.rechargeapps.com/accounts' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d limit=3 -G
{
"accounts": [
{
"id": 3315125,
"user_id": 2057785,
"created_at": "2021-07-20T09:10:19+00:00",
"invited_at": "2021-07-18T09:10:19+00:00",
"is_owner": false
},
{
"id": 3316125,
"user_id": 2056785,
"created_at": "2021-05-20T09:10:19+00:00",
"invited_at": null,
"is_owner": true
}
]
}
Events
This resource allows a caller to inspect recent events in the store.
The event object
Pro plan
An Event
record represents an action that took place at a point in time.
Attributes
- idinteger
Unique numeric identifier for the
Event
. - object_idinteger
The unique numeric identifier of the object associated with the
Event
. - customer_idinteger
The unique numeric identifier of the customer associated with the
Event
.This value can be
null
for events not directly associated with a customer. - created_atdatetime
The date and time when the
Event
was created. - object_typestring
The resource type of the object associated with the
Event
, e.g. ‘customer’ or ‘subscription’ - verbstring
The action describing the
Event
, e.g. ‘created’ or ‘updated’ - descriptionstring
The short description that summarizes the
Event
. - updated_attributesobject
A list of objects describing attributes that were changed during the
Event
.Show object attributes - sourceobject
An object containing details about the origin of the
Event
.Show object attributes - custom_attributesobject
A list of objects passed explicitly to a Recharge API call that created the
Event
.This field will only be populated on events created using the Recharge API.
Show object attributes
Error related attributes
More Attributes
{
"event": {
"id": 7160141294,
"object_id": 362310818,
"customer_id": 36885098,
"created_at": "2023-05-31T14:16:07",
"object_type": "subscription",
"verb": "updated",
"description": "Updated subscription #362310818",
"updated_attributes": [
{
"attribute": "price",
"previous_value": "12.00",
"value": "15.00"
},
{
"attribute": "quantity",
"previous_value": "3",
"value": "5"
}
],
"source": {
"account_id": null,
"api_token_id": 987654321,
"api_token_name": "Subscription API token",
"account_email": null,
"origin": "api",
"user_type": null
},
"custom_attributes": [
{
"key": "ab_testing_flag",
"value": "a"
}
]
}
}
List events
Returns all events associated with the store. The Events API is limited to events that occurred in the last 7 days
Granting the read_events
scope to a token will expose some details about other Recharge API resources via the Events API.
Query Parameters
- created_at_minstring
Show events that occurred after a certain date
- created_at_maxstring
Show events that occurred before a certain date
- object_typestring
Show events that occurred for a certain
object_type
, such ascustomers
,addresses
,charges
, etc. - object_idinteger
Show events with a specific
object_id
. This filter should be used with theobject_type
filter. - verbsstring
Show events with a specific
verb
or comma-separated list ofverbs
- customer_idinteger
Show events related to a specific
customer
using the customer’s primary identifier - originstring
Show events with a specific source.origin, such as
api
,customer_portal
,merchant_portal
, orrecharge_process
.
More Parameters
Responses
- 200
successful response
Show response object
curl 'https://api.rechargeapps.com/events' \
-H 'X-Recharge-Version: 2021-11' \
-H 'X-Recharge-Access-Token: your_api_token' \
-d limit=3 -G
{
"events": [
{
"id": 7160141294,
"object_id": 362310818,
"customer_id": 36885098,
"created_at": "2023-05-31T14:16:07",
"object_type": "subscription",
"verb": "updated",
"description": "Updated subscription #362310818",
"updated_attributes": [
{
"attribute": "price",
"previous_value": "12.00",
"value": "15.00"
},
{
"attribute": "quantity",
"previous_value": "3",
"value": "5"
}
],
"source": {
"account_id": null,
"api_token_id": 987654321,
"api_token_name": "Subscription API token",
"account_email": null,
"origin": "api",
"user_type": null
},
"custom_attributes": [
{
"key": "ab_testing_flag",
"value": "a"
}
]
}
]
}